filmov
tv
Python One‑Liner: Sum Values in a List of Dicts by Key! 💡 #PythonTips #CodingShorts
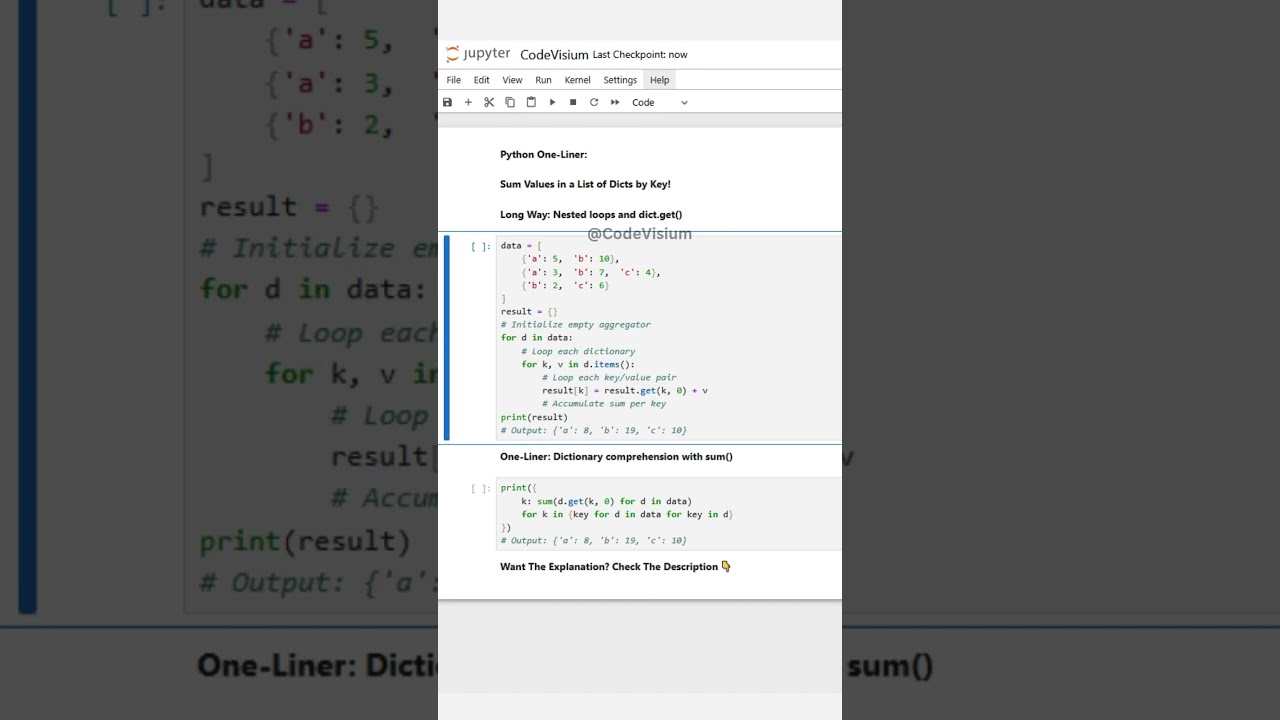
Показать описание
Aggregating numeric values by key across multiple dictionaries is essential in analytics, logging, and data consolidation tasks
Long Way Explanation
Initialize Aggregator:
Start with an empty dictionary result = {} to hold sums per key.
Outer Loop:
Iterate over each dict d in data (for d in data:)
Inner Loop & Accumulation:
Result:
After processing all entries, result maps each key to the total of its values—e.g., 'b': 10 + 7 + 2 = 19.
One‑Liner Explanation
{
for k in {key for d in data for key in d}
}
Key Set Comprehension:
{key for d in data for key in d} builds the set of all unique keys across data in O(n·m) time, where n = number of dicts and m = avg keys per dict
Value Summation:
Conciseness & Readability:
This single expression clearly states “for each key, compute the sum of its occurrences,” without explicit loops.
Why This Matters
Data Consolidation: Quickly merge metrics (sales, clicks, counts) from disparate sources.
Clean Code: Replace boilerplate nested loops with a clear, declarative comprehension.
Performance: Runs in linear time relative to the total number of key/value pairs processed.
Codes:
data = [
{'a': 5, 'b': 10},
{'a': 3, 'b': 7, 'c': 4},
{'b': 2, 'c': 6}
]
result = {}
# Initialize empty aggregator
for d in data:
# Loop each dictionary
# Loop each key/value pair
# Accumulate sum per key
print(result)
# Output: {'a': 8, 'b': 19, 'c': 10}
One‑Liner: Dictionary comprehension with sum():
print({
for k in {key for d in data for key in d}
})
# Output: {'a': 8, 'b': 19, 'c': 10}
Long Way Explanation
Initialize Aggregator:
Start with an empty dictionary result = {} to hold sums per key.
Outer Loop:
Iterate over each dict d in data (for d in data:)
Inner Loop & Accumulation:
Result:
After processing all entries, result maps each key to the total of its values—e.g., 'b': 10 + 7 + 2 = 19.
One‑Liner Explanation
{
for k in {key for d in data for key in d}
}
Key Set Comprehension:
{key for d in data for key in d} builds the set of all unique keys across data in O(n·m) time, where n = number of dicts and m = avg keys per dict
Value Summation:
Conciseness & Readability:
This single expression clearly states “for each key, compute the sum of its occurrences,” without explicit loops.
Why This Matters
Data Consolidation: Quickly merge metrics (sales, clicks, counts) from disparate sources.
Clean Code: Replace boilerplate nested loops with a clear, declarative comprehension.
Performance: Runs in linear time relative to the total number of key/value pairs processed.
Codes:
data = [
{'a': 5, 'b': 10},
{'a': 3, 'b': 7, 'c': 4},
{'b': 2, 'c': 6}
]
result = {}
# Initialize empty aggregator
for d in data:
# Loop each dictionary
# Loop each key/value pair
# Accumulate sum per key
print(result)
# Output: {'a': 8, 'b': 19, 'c': 10}
One‑Liner: Dictionary comprehension with sum():
print({
for k in {key for d in data for key in d}
})
# Output: {'a': 8, 'b': 19, 'c': 10}