filmov
tv
JavaScript Problem: Flattening an Array of Sub-arrays
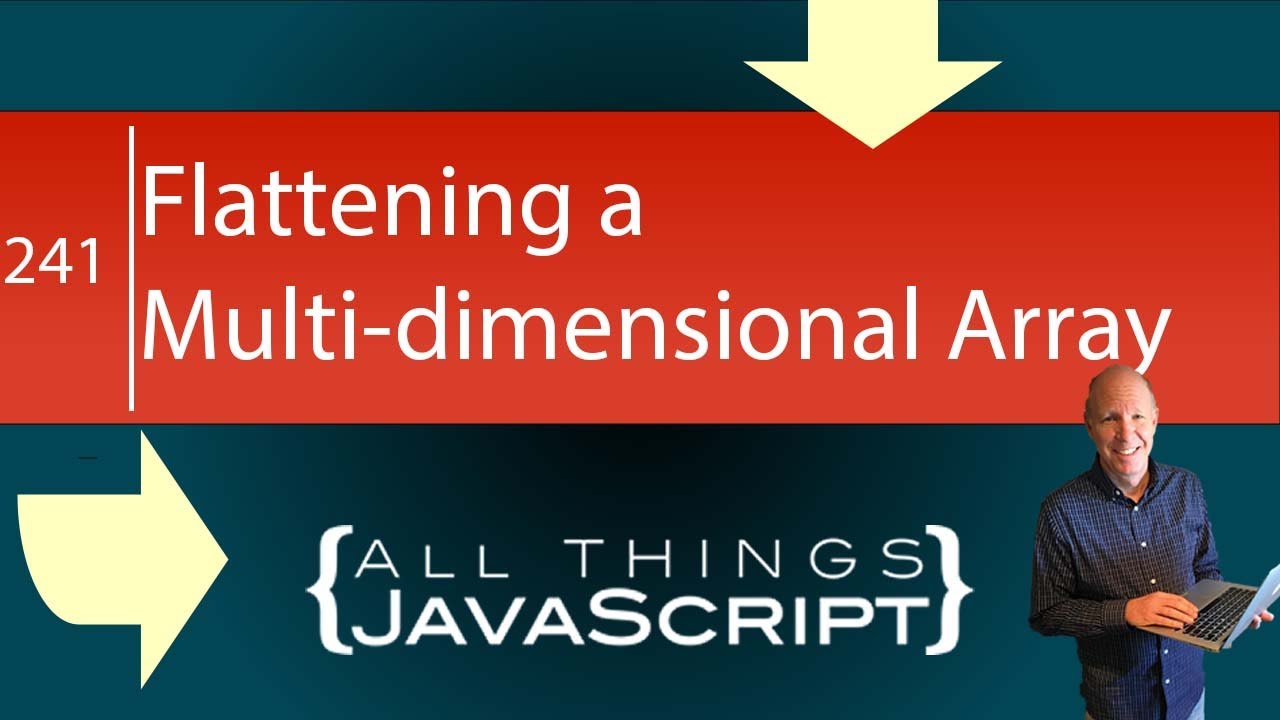
Показать описание
A multi-dimensional array is basically an array of sub-arrays. What if you had such an array and you needed to flatten it; take all of the elements and include them in a single array without any sub-arrays. That is the problem we will solve in this tutorial. The solutions involves recursion and uses other syntax in interesting ways.
Would you like to help keep this channel going?
Tutorials referred to in this video:
For more resources on JavaScript:
#javascript #AllThingsJavaScriptLLC
Would you like to help keep this channel going?
Tutorials referred to in this video:
For more resources on JavaScript:
#javascript #AllThingsJavaScriptLLC
JavaScript Problem: Flattening an Array of Sub-arrays
JavaScript Question: How Can I Flatten an Array?
Q3 Flatten an Array | Flipkart Frontend Interview Questions | Javascript Questions for Interviews
Flatten Deeply Nested Array - Leetcode 2625 - JavaScript 30-Day Challenge
Flatten an array using JavaScript | Beginner level | Code along with Vishal
How to Flatten an Array in JavaScript
Flatten Array & Object in JavaScript || Interview Question
How to Flatten an Array in JavaScript
Q4 Flatten Deeply Nested Object | Adobe Frontend Interview Questions | Javascript Questions
Flatten Nested Arrays with Recursion in JavaScript
Flatten Nested Arrays
Javascript Coding Interview Question #3 - Flatten nested array using recursion
Flattening an array in JS (array.flat() polyfill).
JavaScript Interview Question - 18 | Deep flatten an object
🔴 Flat an array without built-in method | Js interview question | Js Tutorial | In Tamil
Flattening an array of array in JavaScript | geeksforcode
JavaScript Array Flattening Made Easy with Array.flat()#javascript #array #coding #tutorial
Javascript Flattening Two Dimension array
JavaScript interview Question || Flattening the given array interview question 2023
How to Flatten JavaScript Object of Unknown Hierarchy | Recursion 4K
Frontend Interview Question | How to Flatten Array of Array ? | Recursion| Javascript Interview
JavaScript Array Methods , flat , flatMap , reduceRight, copyWithin
Convert Multidimensional Array to Single Array in JavaScript | Array flattening in JavaScript
Recursion - Flattening An Array
Комментарии