filmov
tv
Efficiently Sum Neighbors in a NumPy Matrix Using Python
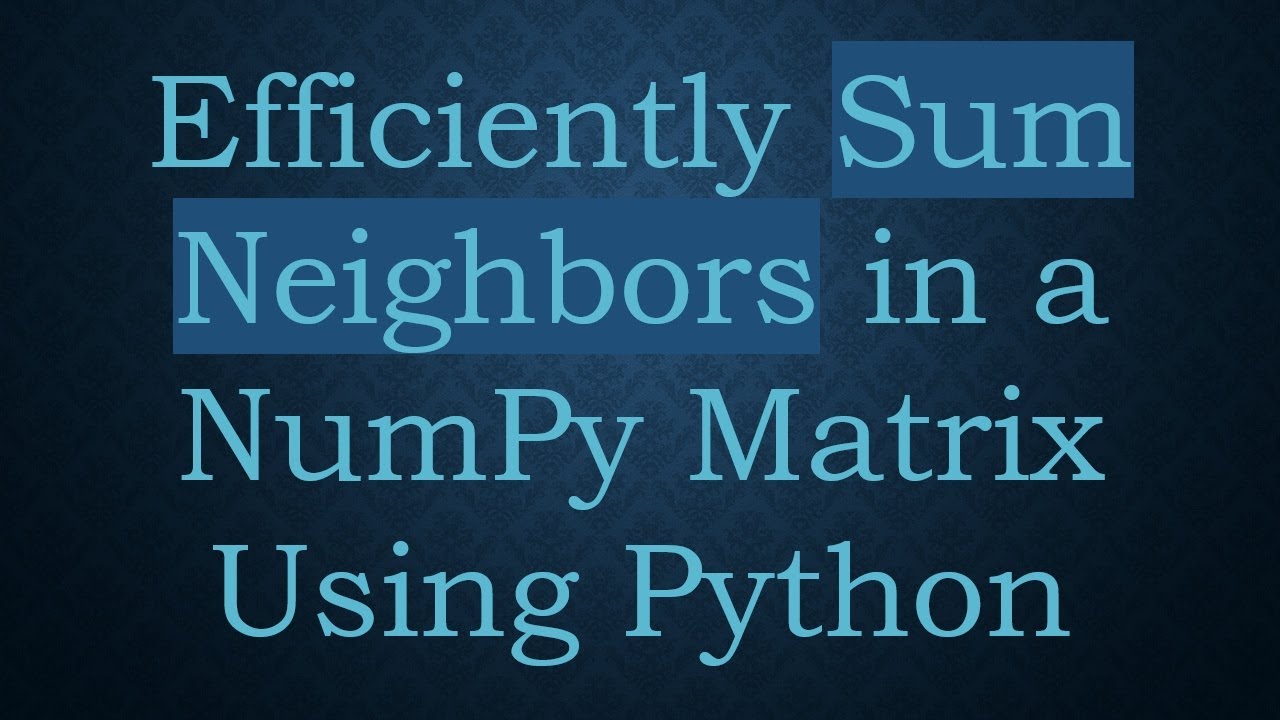
Показать описание
Learn how to efficiently add 1 to the neighbors of a value in a NumPy matrix using convolution techniques in Python.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: numpy array of 2 dimensions indexing and sum
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficiently Sum Neighbors in a NumPy Matrix
If you’re working with matrices in Python using NumPy, you may encounter situations where you need to modify a matrix based on the values of its neighboring cells. For instance, adding a value to all neighbors of a certain target value can be tricky, especially at the edges and corners of the matrix. In this guide, we’ll explore a clever and efficient way to achieve this without using cumbersome conditional statements.
The Problem
Consider the following example of a 2D NumPy array (or matrix):
[[See Video to Reveal this Text or Code Snippet]]
Here, if we want to add 1 to each neighbor of the cell containing the value 7, we may quickly find ourselves needing complex logic to handle matrix edges and corners. Instead of becoming bogged down by multiple conditional checks, there's a more efficient solution.
The Solution
Using Convolution
The key to solving this problem lies in using convolution to sum the neighbor cells. Convolution operations can efficiently compute the sum of an array with its neighbors, essentially allowing us to apply a kernel (a small matrix) over the original matrix.
Step-by-Step Implementation
Import Necessary Libraries: First, we need to import NumPy and any required functions.
[[See Video to Reveal this Text or Code Snippet]]
Define the Kernel: For our purpose, we’ll define a kernel that indicates which neighboring cells to sum.
[[See Video to Reveal this Text or Code Snippet]]
This kernel will sum up all the adjacent cells while ignoring the center cell.
Perform the Convolution: Once we have our kernel, we can convolve it with the condition of the target value (in our case, 7).
[[See Video to Reveal this Text or Code Snippet]]
Output
After applying the convolution, the output matrix would look like this:
[[See Video to Reveal this Text or Code Snippet]]
In this result, every cell that was a neighbor to the 7 cells has been incremented by 1.
Conclusion
Using convolution to sum neighbors in a NumPy matrix is not just efficient but also elegant, avoiding the pitfalls of complex conditional checks. The method we discussed efficiently updates the matrix while keeping your code clean and concise. If you frequently work with 2D arrays and need to apply similar updates, mastering this approach can significantly enhance your productivity in Python programming.
Feel free to adapt the kernel for different types of neighbor summations, and explore the vast capabilities of convolution in your numerical tasks!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: numpy array of 2 dimensions indexing and sum
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Efficiently Sum Neighbors in a NumPy Matrix
If you’re working with matrices in Python using NumPy, you may encounter situations where you need to modify a matrix based on the values of its neighboring cells. For instance, adding a value to all neighbors of a certain target value can be tricky, especially at the edges and corners of the matrix. In this guide, we’ll explore a clever and efficient way to achieve this without using cumbersome conditional statements.
The Problem
Consider the following example of a 2D NumPy array (or matrix):
[[See Video to Reveal this Text or Code Snippet]]
Here, if we want to add 1 to each neighbor of the cell containing the value 7, we may quickly find ourselves needing complex logic to handle matrix edges and corners. Instead of becoming bogged down by multiple conditional checks, there's a more efficient solution.
The Solution
Using Convolution
The key to solving this problem lies in using convolution to sum the neighbor cells. Convolution operations can efficiently compute the sum of an array with its neighbors, essentially allowing us to apply a kernel (a small matrix) over the original matrix.
Step-by-Step Implementation
Import Necessary Libraries: First, we need to import NumPy and any required functions.
[[See Video to Reveal this Text or Code Snippet]]
Define the Kernel: For our purpose, we’ll define a kernel that indicates which neighboring cells to sum.
[[See Video to Reveal this Text or Code Snippet]]
This kernel will sum up all the adjacent cells while ignoring the center cell.
Perform the Convolution: Once we have our kernel, we can convolve it with the condition of the target value (in our case, 7).
[[See Video to Reveal this Text or Code Snippet]]
Output
After applying the convolution, the output matrix would look like this:
[[See Video to Reveal this Text or Code Snippet]]
In this result, every cell that was a neighbor to the 7 cells has been incremented by 1.
Conclusion
Using convolution to sum neighbors in a NumPy matrix is not just efficient but also elegant, avoiding the pitfalls of complex conditional checks. The method we discussed efficiently updates the matrix while keeping your code clean and concise. If you frequently work with 2D arrays and need to apply similar updates, mastering this approach can significantly enhance your productivity in Python programming.
Feel free to adapt the kernel for different types of neighbor summations, and explore the vast capabilities of convolution in your numerical tasks!