filmov
tv
Python Tkinter threading button command and main loop
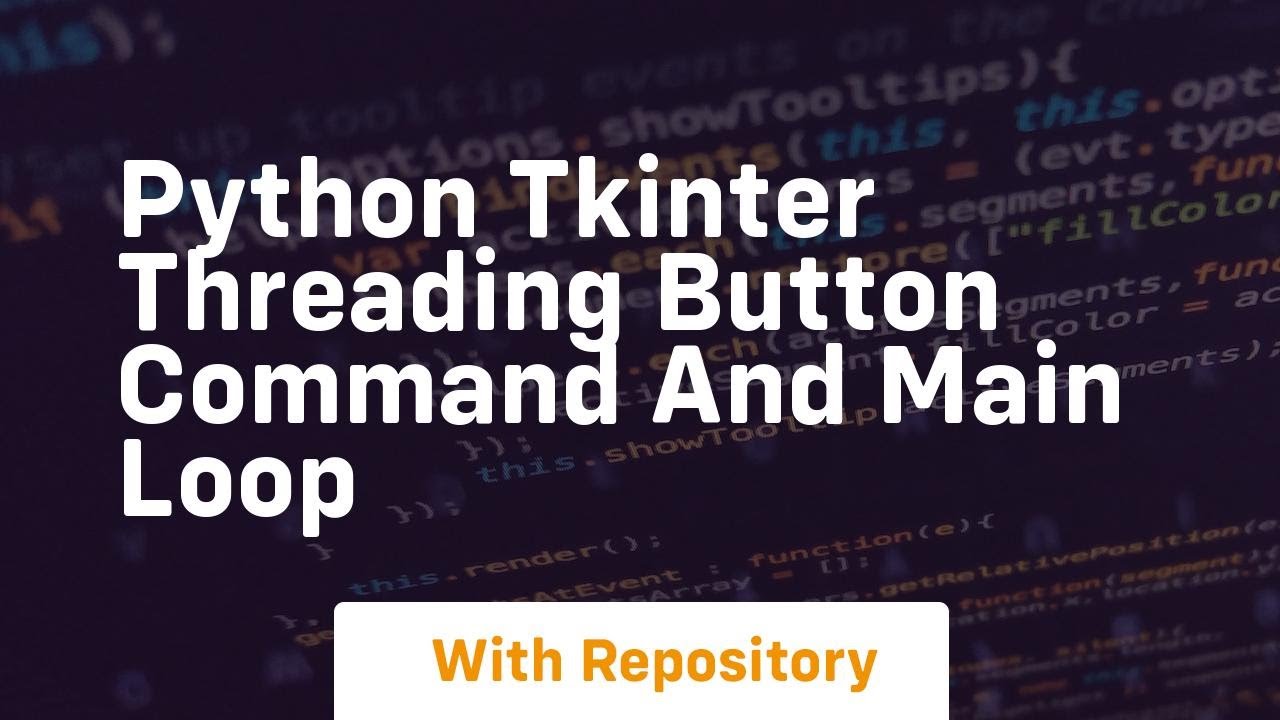
Показать описание
Sure, let's create a tutorial on using Python's Tkinter with threading for handling button commands. In this tutorial, I'll guide you through the process of creating a simple GUI application with a button that runs a task in a separate thread, allowing the main GUI to remain responsive.
Tkinter is Python's standard GUI (Graphical User Interface) library, and threading is a way to run tasks concurrently. Combining Tkinter with threading allows you to create responsive GUI applications that can perform time-consuming tasks without freezing the user interface.
In this tutorial, we'll create a Tkinter application with a button. When the button is pressed, a time-consuming task will be executed in a separate thread, leaving the GUI responsive.
Make sure you have Python installed on your system. Tkinter is included with most Python installations.
We start by importing the necessary modules: tkinter, messagebox for displaying messages, threading for managing threads, and time for simulating a time-consuming task.
The MyApp class is created to represent our main application. In the constructor (__init__), we set up the main window and call the create_widgets method to initialize the GUI components.
The create_widgets method creates a button with the label "Run Task" and associates it with the run_task method using the command attribute.
The run_task method is called when the button is pressed. It starts a new thread (task_thread) that runs the time_consuming_task method.
The show_task_complete_message method displays a message box to notify the user that the task is complete.
This simple example demonstrates how to use threading in Tkinter to keep your GUI responsive while performing time-consuming tasks. Remember to handle synchronization carefully when working with threads to avoid potential issues.
ChatGPT
Tkinter is Python's standard GUI (Graphical User Interface) library, and threading is a way to run tasks concurrently. Combining Tkinter with threading allows you to create responsive GUI applications that can perform time-consuming tasks without freezing the user interface.
In this tutorial, we'll create a Tkinter application with a button. When the button is pressed, a time-consuming task will be executed in a separate thread, leaving the GUI responsive.
Make sure you have Python installed on your system. Tkinter is included with most Python installations.
We start by importing the necessary modules: tkinter, messagebox for displaying messages, threading for managing threads, and time for simulating a time-consuming task.
The MyApp class is created to represent our main application. In the constructor (__init__), we set up the main window and call the create_widgets method to initialize the GUI components.
The create_widgets method creates a button with the label "Run Task" and associates it with the run_task method using the command attribute.
The run_task method is called when the button is pressed. It starts a new thread (task_thread) that runs the time_consuming_task method.
The show_task_complete_message method displays a message box to notify the user that the task is complete.
This simple example demonstrates how to use threading in Tkinter to keep your GUI responsive while performing time-consuming tasks. Remember to handle synchronization carefully when working with threads to avoid potential issues.
ChatGPT