filmov
tv
Mastering File Manipulation in C#: Removing, Splitting, and Retrieving Extensions
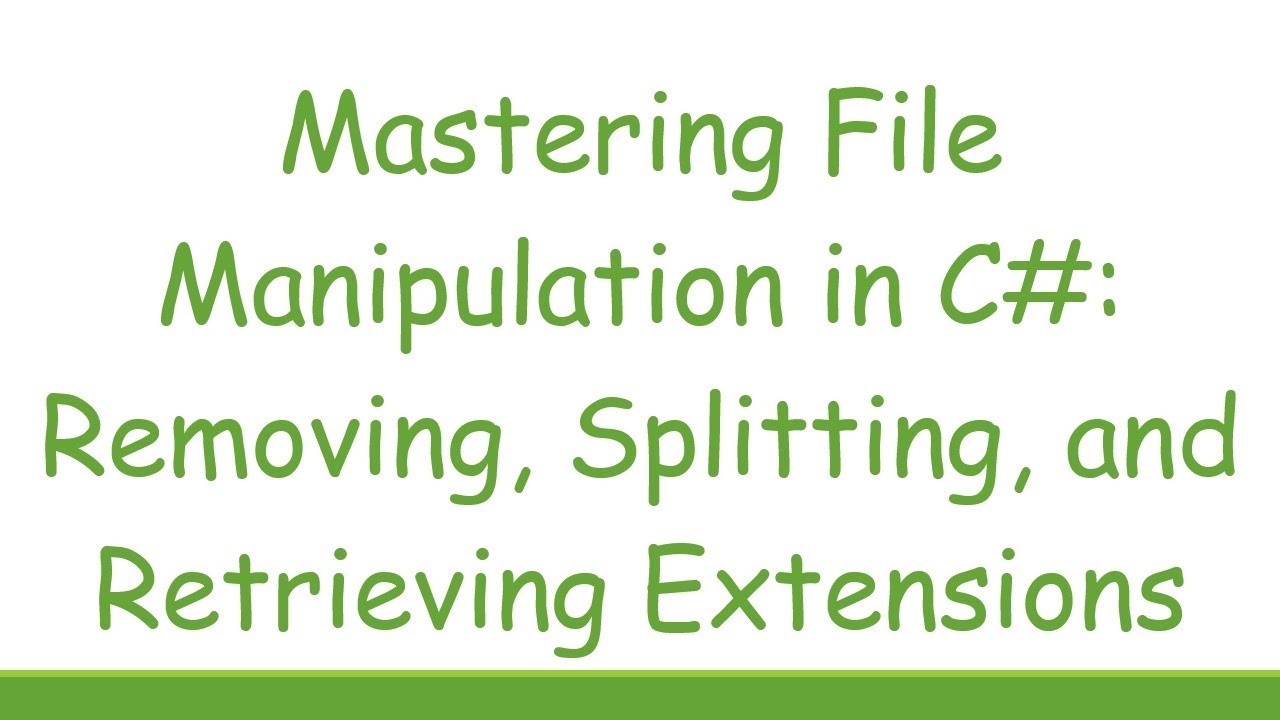
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to efficiently manage file names and extensions in C# by mastering techniques to remove, split, and retrieve extensions from file names.
---
Mastering File Manipulation in C: Removing, Splitting, and Retrieving Extensions
When dealing with file operations in C, there are common tasks that one might encounter such as manipulating file names and their extensions. Whether you need to remove an extension, split a file name and its extension, or retrieve the file name and extension, C provides straightforward ways to achieve this.
Here are some practical techniques to handle these tasks efficiently:
Removing Extension from a File Name in C
To remove the extension from a file name, you can use the Path.GetFileNameWithoutExtension method provided by the System.IO namespace. This method extracts the file name without its extension:
[[See Video to Reveal this Text or Code Snippet]]
Splitting File Name and Extension in C
Splitting a file name and its extension involves separating the base name from its extension. You can achieve this by using the Path.GetFileNameWithoutExtension and Path.GetExtension methods:
[[See Video to Reveal this Text or Code Snippet]]
In this code snippet, Path.GetFileNameWithoutExtension extracts the file name, and Path.GetExtension retrieves the file extension.
Retrieving File Name and Extension in C
To retrieve both the file name and the extension, you can use the Path.GetFileName method. This method returns the file name with its extension:
[[See Video to Reveal this Text or Code Snippet]]
This example demonstrates using Path.GetFileName, which returns the full file name including its extension.
Conclusion
Manipulating file names and extensions is a fundamental aspect of file handling in C. By utilizing the built-in methods provided by the System.IO namespace, such as Path.GetFileNameWithoutExtension, Path.GetExtension, and Path.GetFileName, you can efficiently remove, split, and retrieve file names and their extensions. These methods streamline the process, making your code cleaner and more readable.
Are there other file operations you're interested in exploring? Feel free to share in the comments below.
---
Summary: Learn how to efficiently manage file names and extensions in C# by mastering techniques to remove, split, and retrieve extensions from file names.
---
Mastering File Manipulation in C: Removing, Splitting, and Retrieving Extensions
When dealing with file operations in C, there are common tasks that one might encounter such as manipulating file names and their extensions. Whether you need to remove an extension, split a file name and its extension, or retrieve the file name and extension, C provides straightforward ways to achieve this.
Here are some practical techniques to handle these tasks efficiently:
Removing Extension from a File Name in C
To remove the extension from a file name, you can use the Path.GetFileNameWithoutExtension method provided by the System.IO namespace. This method extracts the file name without its extension:
[[See Video to Reveal this Text or Code Snippet]]
Splitting File Name and Extension in C
Splitting a file name and its extension involves separating the base name from its extension. You can achieve this by using the Path.GetFileNameWithoutExtension and Path.GetExtension methods:
[[See Video to Reveal this Text or Code Snippet]]
In this code snippet, Path.GetFileNameWithoutExtension extracts the file name, and Path.GetExtension retrieves the file extension.
Retrieving File Name and Extension in C
To retrieve both the file name and the extension, you can use the Path.GetFileName method. This method returns the file name with its extension:
[[See Video to Reveal this Text or Code Snippet]]
This example demonstrates using Path.GetFileName, which returns the full file name including its extension.
Conclusion
Manipulating file names and extensions is a fundamental aspect of file handling in C. By utilizing the built-in methods provided by the System.IO namespace, such as Path.GetFileNameWithoutExtension, Path.GetExtension, and Path.GetFileName, you can efficiently remove, split, and retrieve file names and their extensions. These methods streamline the process, making your code cleaner and more readable.
Are there other file operations you're interested in exploring? Feel free to share in the comments below.