filmov
tv
Understanding Local and Nested Functions in Python
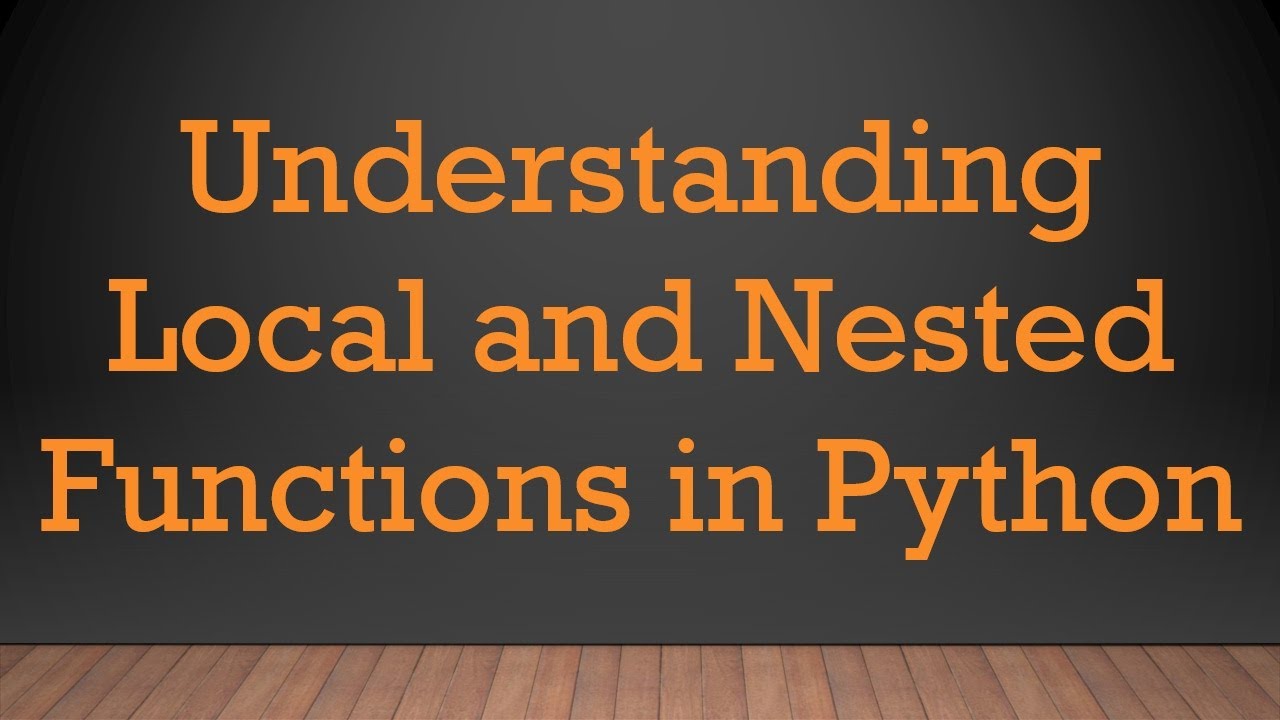
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Explore the concepts of local and nested functions in Python, their definitions, usage, examples, and how they integrate into classes and lambda expressions.
---
Understanding Local and Nested Functions in Python
Python is a versatile programming language known for its readability and flexibility. Among its many features is the ability to define functions with different scopes, including local and nested functions. This guide will delve into these concepts, providing examples and insights into their usage.
Local Functions in Python
Local functions in Python are defined inside other functions and are scoped locally to the enclosing function. This means they can only be accessed within the function in which they are defined.
Here’s a basic example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, local_function is a local function within outer_function. It is only accessible within the outer_function.
Nested Functions in Python
Nested functions are essentially the same as local functions; they are functions defined within other functions. These nested functions can be powerful, especially when dealing with closures or creating decorator functions.
Nested Functions with Examples
Consider the following example that demonstrates nested functions:
[[See Video to Reveal this Text or Code Snippet]]
In this example, child_function is defined inside parent_function and has access to the message parameter, demonstrating the concept of closures.
Local Method in Python
A local method in Python refers to a method defined within the scope of another method inside a class. Here’s an example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, local_method is a method defined within outer_method and has access to its parameters.
Local Variables in Functions in Python
Local variables in functions are variables that are defined within a function and can only be accessed within that function. This is essential for encapsulation and avoiding variable name conflicts.
[[See Video to Reveal this Text or Code Snippet]]
Local Time Function in Python
The localtime() function in Python provides the local time from the time module. Here’s how to use it:
[[See Video to Reveal this Text or Code Snippet]]
Local Functions in Class Python
A local function within a class method can be useful for encapsulating functionality that doesn't need to be exposed outside the method.
[[See Video to Reveal this Text or Code Snippet]]
Nested Lambda Functions in Python
Lambda functions can also be nested within other functions or lambda functions. They provide a concise way to create small anonymous functions.
[[See Video to Reveal this Text or Code Snippet]]
In this example, nested_lambda is a function that returns a lambda function, showcasing the concept of nested lambda functions.
Conclusion
Understanding local and nested functions in Python can greatly improve your coding practice by enhancing encapsulation and modularity. Whether you are defining simple functions, working within classes, or creating concise lambda functions, these concepts provide a foundation for writing clean and efficient code.
Delve into these examples, experiment with your own code, and see how local and nested functions can streamline your Python programming experience.
---
Summary: Explore the concepts of local and nested functions in Python, their definitions, usage, examples, and how they integrate into classes and lambda expressions.
---
Understanding Local and Nested Functions in Python
Python is a versatile programming language known for its readability and flexibility. Among its many features is the ability to define functions with different scopes, including local and nested functions. This guide will delve into these concepts, providing examples and insights into their usage.
Local Functions in Python
Local functions in Python are defined inside other functions and are scoped locally to the enclosing function. This means they can only be accessed within the function in which they are defined.
Here’s a basic example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, local_function is a local function within outer_function. It is only accessible within the outer_function.
Nested Functions in Python
Nested functions are essentially the same as local functions; they are functions defined within other functions. These nested functions can be powerful, especially when dealing with closures or creating decorator functions.
Nested Functions with Examples
Consider the following example that demonstrates nested functions:
[[See Video to Reveal this Text or Code Snippet]]
In this example, child_function is defined inside parent_function and has access to the message parameter, demonstrating the concept of closures.
Local Method in Python
A local method in Python refers to a method defined within the scope of another method inside a class. Here’s an example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, local_method is a method defined within outer_method and has access to its parameters.
Local Variables in Functions in Python
Local variables in functions are variables that are defined within a function and can only be accessed within that function. This is essential for encapsulation and avoiding variable name conflicts.
[[See Video to Reveal this Text or Code Snippet]]
Local Time Function in Python
The localtime() function in Python provides the local time from the time module. Here’s how to use it:
[[See Video to Reveal this Text or Code Snippet]]
Local Functions in Class Python
A local function within a class method can be useful for encapsulating functionality that doesn't need to be exposed outside the method.
[[See Video to Reveal this Text or Code Snippet]]
Nested Lambda Functions in Python
Lambda functions can also be nested within other functions or lambda functions. They provide a concise way to create small anonymous functions.
[[See Video to Reveal this Text or Code Snippet]]
In this example, nested_lambda is a function that returns a lambda function, showcasing the concept of nested lambda functions.
Conclusion
Understanding local and nested functions in Python can greatly improve your coding practice by enhancing encapsulation and modularity. Whether you are defining simple functions, working within classes, or creating concise lambda functions, these concepts provide a foundation for writing clean and efficient code.
Delve into these examples, experiment with your own code, and see how local and nested functions can streamline your Python programming experience.