filmov
tv
9 Searching in a Nearly Sorted Array
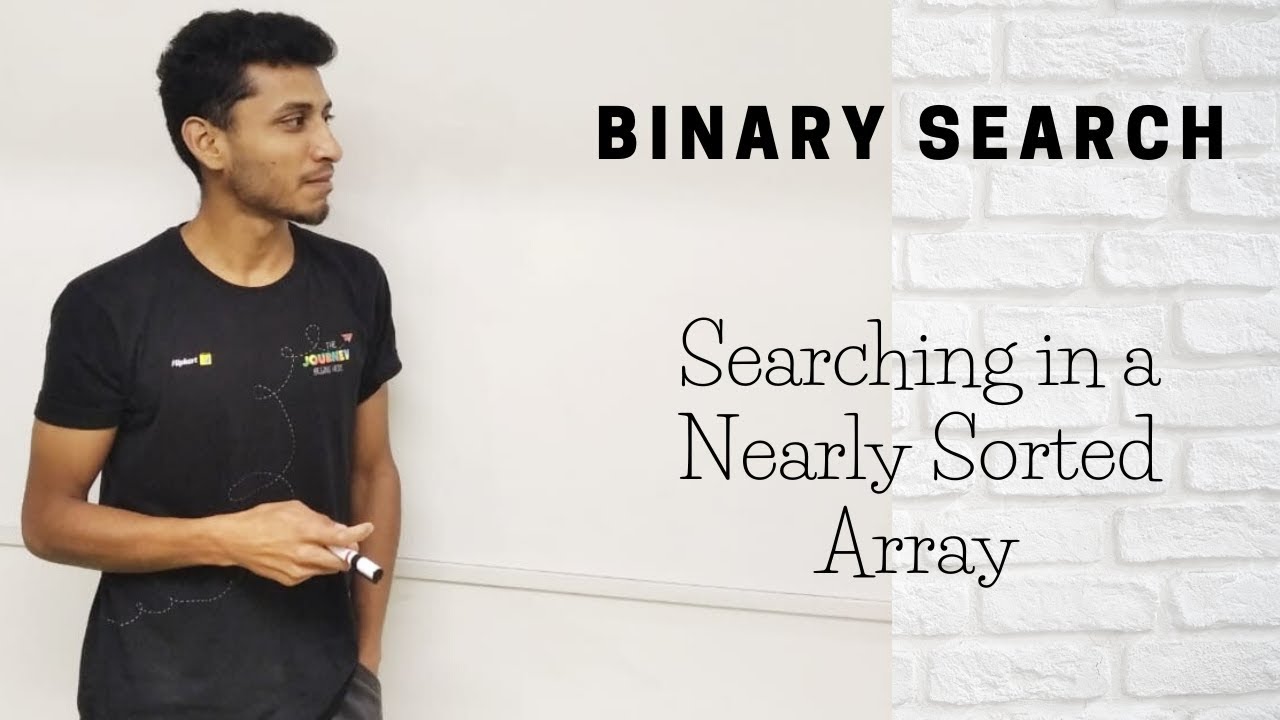
Показать описание
SEARCH IN A NEARLY SORTED ARRAY:
Given an array which is sorted, but after sorting some elements are moved to either of the adjacent positions, i.e., arr[i] may be present at arr[i+1] or arr[i-1]. Write an efficient function to search an element in this array. Basically the element arr[i] can only be swapped with either arr[i+1] or arr[i-1].
For example consider the array {2, 3, 10, 4, 40}, 4 is moved to next position and 10 is moved to previous position.
Example :
Input: arr[] = {10, 3, 40, 20, 50, 80, 70}, key = 40
Output: 2
Output is index of 40 in given array
------------------------------------------------------------------------------------------
Here are some of the gears that I use almost everyday:
PS: While having good gears help you perform efficiently, don’t get under the impression that they will make you successful without any hard work.
Given an array which is sorted, but after sorting some elements are moved to either of the adjacent positions, i.e., arr[i] may be present at arr[i+1] or arr[i-1]. Write an efficient function to search an element in this array. Basically the element arr[i] can only be swapped with either arr[i+1] or arr[i-1].
For example consider the array {2, 3, 10, 4, 40}, 4 is moved to next position and 10 is moved to previous position.
Example :
Input: arr[] = {10, 3, 40, 20, 50, 80, 70}, key = 40
Output: 2
Output is index of 40 in given array
------------------------------------------------------------------------------------------
Here are some of the gears that I use almost everyday:
PS: While having good gears help you perform efficiently, don’t get under the impression that they will make you successful without any hard work.
9 Searching in a Nearly Sorted Array
iPhone 6 Plus approximately 9 jumpers in the first layer and 5 in the second layer, long screw
YouTube… Where it all started nearly 9 years ago back in my shed
The Google Monopoly Is Coming To An End
Store Shelves are Nearly Full Again; Supply Chain Success
9-foot gator nearly breaks into Tampa couple's home
Federal politicians to get biggest pay rise in nearly a decade | 9 News Australia
FX Parabol Review (+- 9% Growth Per Month For Nearly 3 years)
Sheriff's office searching for missing Liberty Township man not seen for nearly a week
PROJECT 10 USES #9! (nearly all rolling out!!)
A first look at the 9-8-8 crisis lifeline, nearly 500 GA callers ‘rescued’
9 Nearly Hairless Mammals #trending #shorts #facts || Zeerak's Biology
9 facts about Anne Boleyn: 2. Anne nearly married her Irish cousin, James Butler, 9th Earl of Ormond
Nearly 3 million people filed for unemployment for week ending May 9
Unemployed Arizonan receives nearly $5,000 back after 9-month battle with Arizona DES | FOX 10 News
Xiaomi Mi 9 nearly bezel-less display with a waterdrop notch||Millet concept
Man charged with murder of Sydney woman nearly four months on | 9 News Australia
Nearly 9-foot alligator pulled from Florida pool
Black lab Parkes Nearly 13 years old . #dogparkour 2022 , 9
Nearly 3,000 flags in place at ISU to remember 9/11 victims
#TheMoment a runaway tortoise was rescued nearly a kilometre from home
A Short History of Nearly Everything - Chapter 9. The Mighty Atom
Raleigh rental apartment booms, new downtown complex nearly full in 9 months
$3.50 for 9 items at the Nearly New Thrift Store #shopping #thrifting
Комментарии