filmov
tv
Avoid premature abstraction with Unstyled Components
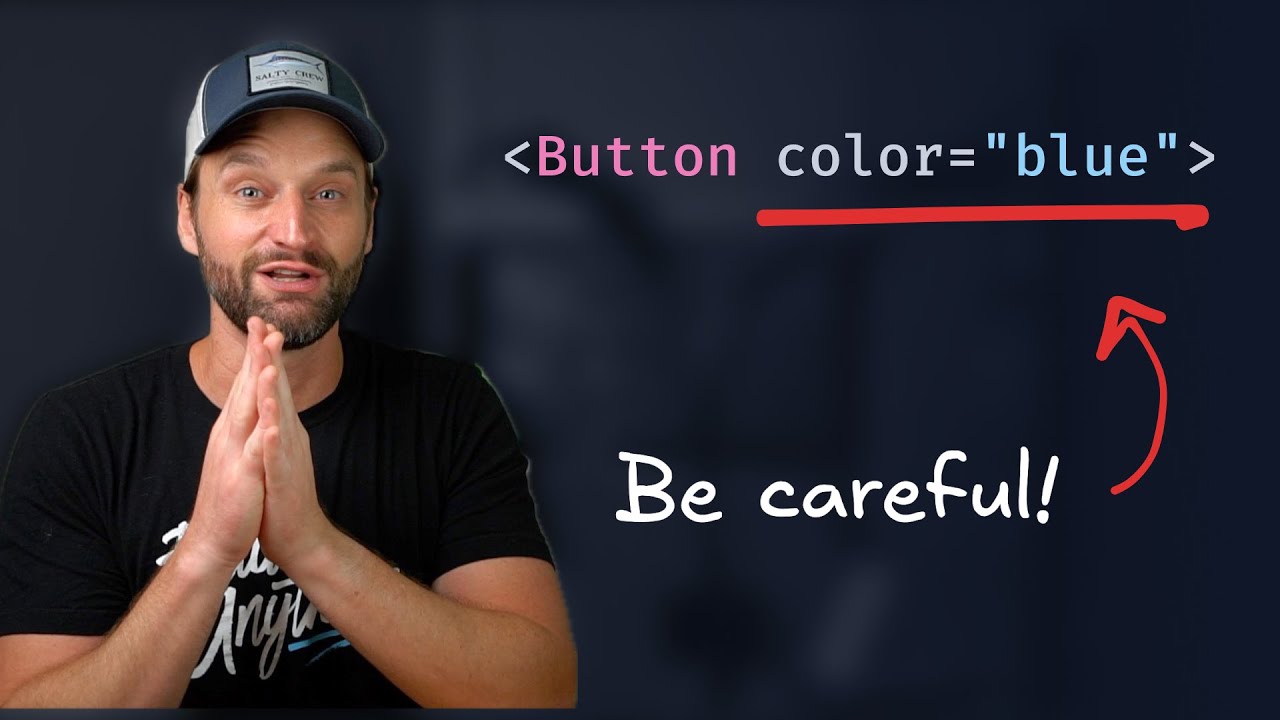
Показать описание
As programmers we love abstractions – but if you abstract the wrong part of your code, the cure is worse than the disease. Learn how unstyled React components can help you extract smaller chunks of logic without jumping straight to a premature, overly abstracted component.
Timestamps:
0:00 - Intro
2:10 - Extracting a Button
5:41 - The problem with our abstraction
8:24 - Extracting an Unstyled Component
12:05 - Using ComponentProps and the JSX spread syntax
14:30 - Final demo
16:39 - Advanced React Component Patterns
Timestamps:
0:00 - Intro
2:10 - Extracting a Button
5:41 - The problem with our abstraction
8:24 - Extracting an Unstyled Component
12:05 - Using ComponentProps and the JSX spread syntax
14:30 - Final demo
16:39 - Advanced React Component Patterns
Avoid premature abstraction with Unstyled Components
Ep 193 - Unstyled React components
I WISH I Knew These Tailwind Tips Earlier
Facebook Tried Tailwind, Then Built This Instead
Why Is Everyone So Mad?
How to Build a Universal Design System - Axel Delafosse - React Native London - June 2022
(FINALLY!) Email for Developers
React's Next Big Change?
AngularJS + REST Made Simple: Connecting AngularJS to a Backend with REST & JSON
Automate Performance Boosts Using Experiments
FOUC and the Death of Progressive Enhancement by Kyle Simpson | JSCAMP 2019
Stop using JS for that: moving features from JS to CSS & HTML | Kilian Valkhof | Conf42 JS 2023
Rethinking Design Practices — Mark Dalgleish
How Nuxt 3 is allowing us to build faster applications? by Filip Rakowski: Nuxt Nation 2022
Project Cambria: managing schema change in distributed systems, Geoffrey Litt and Peter van Hard...
Patterns for Reusable JavaScript Components - Cory House
BlinkOn 6 Day 2 Talk 2: Client side performance from the perspective of a developer
Angular: Let's read the code!
Laying out your Django projects - Promises and Pitfalls - PyCon India 2015
Harry Roberts - Architecting Scalable CSS
7. Abstract Art In A Time Of Minification / Jenn Schiffer / ffconf 2017
30-minute Challenge vs. Real Design Process: Which One Wins?
UIT 5: Thoughts on GatsbyJS, the React-based SSG framework
Eric Bailey :: The intersection of accessibility and performance:: #PerfMatters 2019
Комментарии