filmov
tv
Converting Epoch Time in C#
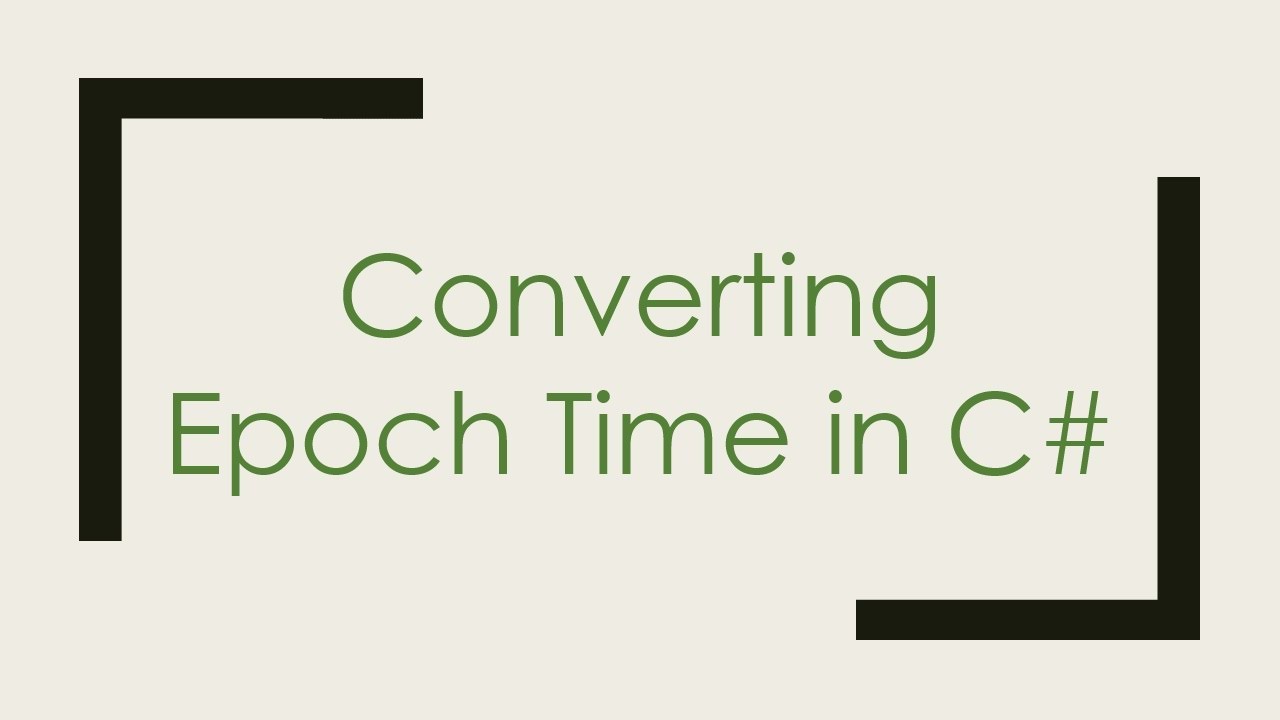
Показать описание
Summary: Learn how to convert epoch time to DateTime and vice versa in C#. This guide provides step-by-step instructions and code examples for handling epoch time conversion in C# applications.
---
Epoch time, also known as Unix time, represents the number of seconds that have elapsed since January 1, 1970 (midnight UTC/GMT). In C, converting between epoch time and DateTime is a common task that can be accomplished using built-in methods. This guide will guide you through the process with clear examples.
Converting Epoch Time to DateTime
To convert epoch time to DateTime in C, you can use the DateTimeOffset class, which provides methods to work with epoch time.
Example
Here's a simple example of how to convert epoch time to DateTime:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
Epoch Time: A long integer representing the number of seconds since January 1, 1970.
DateTimeOffset.FromUnixTimeSeconds: Converts the epoch time to a DateTimeOffset object.
DateTime: Extracts the DateTime from the DateTimeOffset.
This method ensures that the conversion takes into account the time zone and daylight saving time adjustments.
Converting DateTime to Epoch Time
To convert a DateTime object to epoch time, you can use the DateTimeOffset class again. The ToUnixTimeSeconds method converts a DateTimeOffset object to epoch time.
Example
Here's how to convert a DateTime to epoch time:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
DateTime: A DateTime object representing a specific date and time.
DateTimeKind.Utc: Specifies that the DateTime object is in Coordinated Universal Time (UTC).
ToUnixTimeSeconds: Converts the DateTimeOffset object to epoch time.
This approach ensures the accurate conversion of DateTime to epoch time, considering the UTC offset.
Handling Different Time Zones
When working with different time zones, it's essential to be aware of how time zone differences can affect your conversions. Ensure that you convert all times to UTC before performing the epoch conversion.
Example
[[See Video to Reveal this Text or Code Snippet]]
Explanation
DateTimeKind.Local: Specifies that the DateTime object is in the local time zone.
ToUniversalTime: Converts the local time to UTC.
ToUnixTimeSeconds: Converts the DateTimeOffset object to epoch time.
By converting local time to UTC before converting to epoch time, you ensure that the epoch time is accurate and consistent across different time zones.
Conclusion
Converting epoch time to DateTime and vice versa in C is straightforward with the DateTimeOffset class. By following the examples provided, you can easily handle epoch time conversions in your C applications. Remember to always consider the time zone when performing these conversions to ensure accuracy.
---
Epoch time, also known as Unix time, represents the number of seconds that have elapsed since January 1, 1970 (midnight UTC/GMT). In C, converting between epoch time and DateTime is a common task that can be accomplished using built-in methods. This guide will guide you through the process with clear examples.
Converting Epoch Time to DateTime
To convert epoch time to DateTime in C, you can use the DateTimeOffset class, which provides methods to work with epoch time.
Example
Here's a simple example of how to convert epoch time to DateTime:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
Epoch Time: A long integer representing the number of seconds since January 1, 1970.
DateTimeOffset.FromUnixTimeSeconds: Converts the epoch time to a DateTimeOffset object.
DateTime: Extracts the DateTime from the DateTimeOffset.
This method ensures that the conversion takes into account the time zone and daylight saving time adjustments.
Converting DateTime to Epoch Time
To convert a DateTime object to epoch time, you can use the DateTimeOffset class again. The ToUnixTimeSeconds method converts a DateTimeOffset object to epoch time.
Example
Here's how to convert a DateTime to epoch time:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
DateTime: A DateTime object representing a specific date and time.
DateTimeKind.Utc: Specifies that the DateTime object is in Coordinated Universal Time (UTC).
ToUnixTimeSeconds: Converts the DateTimeOffset object to epoch time.
This approach ensures the accurate conversion of DateTime to epoch time, considering the UTC offset.
Handling Different Time Zones
When working with different time zones, it's essential to be aware of how time zone differences can affect your conversions. Ensure that you convert all times to UTC before performing the epoch conversion.
Example
[[See Video to Reveal this Text or Code Snippet]]
Explanation
DateTimeKind.Local: Specifies that the DateTime object is in the local time zone.
ToUniversalTime: Converts the local time to UTC.
ToUnixTimeSeconds: Converts the DateTimeOffset object to epoch time.
By converting local time to UTC before converting to epoch time, you ensure that the epoch time is accurate and consistent across different time zones.
Conclusion
Converting epoch time to DateTime and vice versa in C is straightforward with the DateTimeOffset class. By following the examples provided, you can easily handle epoch time conversions in your C applications. Remember to always consider the time zone when performing these conversions to ensure accuracy.