filmov
tv
JAVA : What is a try-catch block in Java? SDET Automation Testing Interview Questions & Answers
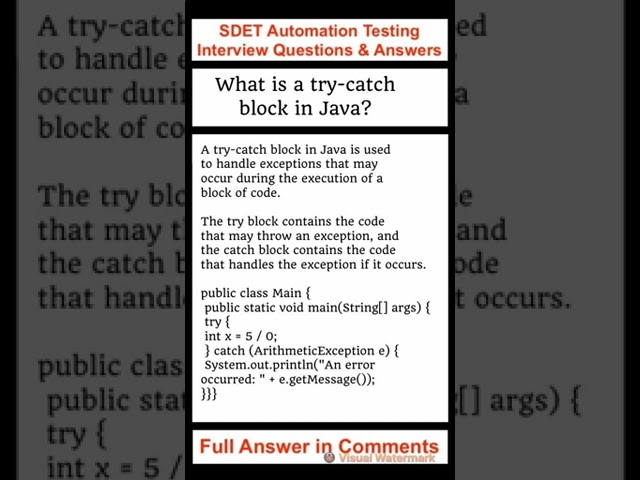
Показать описание
JAVA : What is a try-catch block in Java?
SDET Automation Testing Interview Questions & Answers
We will be covering a wide range of topics including QA manual testing, automation testing, Selenium, Java, Jenkins, Cucumber, Maven, and various testing frameworks.
JAVA : What is a try-catch block in Java?
JAVA : What is a try-catch block in Java?
A try-catch block in Java is used to handle exceptions that may occur during the execution of a block of code.
The try block contains the code that may throw an exception, and the catch block contains the code that handles the exception if it occurs.
Here's an example of a try-catch block in Java:
public class Main {
public static void main(String[] args) {
try {
int x = 5 / 0;
} catch (ArithmeticException e) {
}
}
}
This code attempts to divide the integer 5 by 0, which will throw an ArithmeticException.
The exception is caught by the catch block, which prints an error message to the console.
Without the try-catch block, the exception would cause the program to terminate with an error message.
SDET Automation Testing Interview Questions & Answers
We will be covering a wide range of topics including QA manual testing, automation testing, Selenium, Java, Jenkins, Cucumber, Maven, and various testing frameworks.
JAVA : What is a try-catch block in Java?
JAVA : What is a try-catch block in Java?
A try-catch block in Java is used to handle exceptions that may occur during the execution of a block of code.
The try block contains the code that may throw an exception, and the catch block contains the code that handles the exception if it occurs.
Here's an example of a try-catch block in Java:
public class Main {
public static void main(String[] args) {
try {
int x = 5 / 0;
} catch (ArithmeticException e) {
}
}
}
This code attempts to divide the integer 5 by 0, which will throw an ArithmeticException.
The exception is caught by the catch block, which prints an error message to the console.
Without the try-catch block, the exception would cause the program to terminate with an error message.
Комментарии