filmov
tv
Adding adding to my language (Compiler Pt.4)
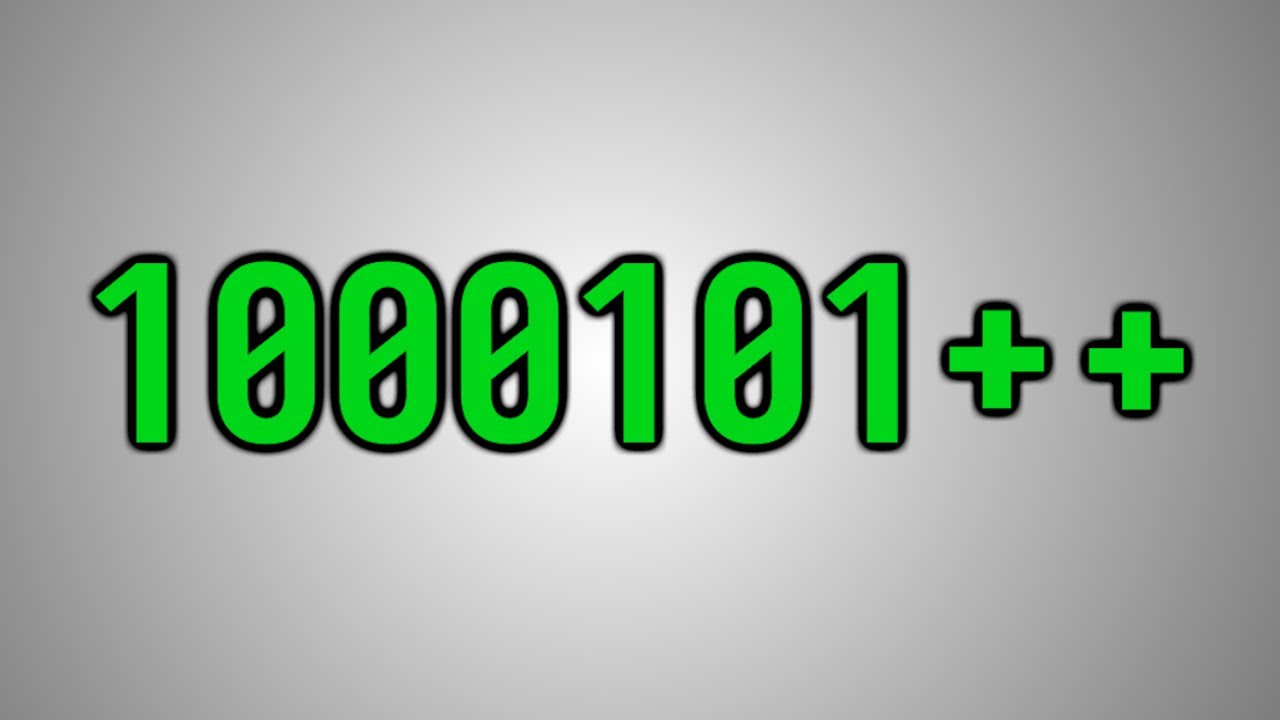
Показать описание
Chapters
========
0:00 Intro
0:45 Math Grammar
6:00 Updating AST in Parser
8:53 The Problem with Pointers
11:44 Explaining an Arena Allocator
15:47 Implementing an Arena Allocator
24:20 Using the Arena Allocator
33:30 Parsing Math Expressions
43:15 Debugging
45:41 Restructuring the Grammar
54:08 Updating the Code Generation
56:27 Addition in Assembly
1:01:11 Surprise Functionality
1:04:53 Refactoring
FAQ
===
CLion Theme: One Dark theme
Keyboard: Keychron V6, Gateron G Pro V2 Brown Switches
Adding adding to my language (Compiler Pt.4)
Adding Local Variables to My Language
Adding Function Arguments to My Language
Multi-Language Management in REDCap, Part 1: Adding Languages
Adding more machine language instructions to the CPU
Adding and Language and Keyboard to your computer
Adding Raw Pointers to My Language
Module 1 - Adding a language
Adding a Multi Purpose Rug to My Learning Area for Multisensory Learning Through Play
Adding a second language to my Samsung Keyboard
Adding another KEYBOARD LANGUAGE In Windows
Windows 10 Mobile adding a language
Episode 6 - Adding multilanguage on public Portals and change default language in ServiceNow
Adding second language on Windows 10
New letter added to my language: Ww (pronunced as We)
Windows 10 - Adding another language
Adding stats to my language app | Unity GameDev Stream
Localization in .NET MAUI - Adding Multi-Language to Your Apps
Adding Google Language Translator to WordPress | The Simplest Method
Adding Vietnamese Language Keyboard to my Iphone 4s running iOS 7. Same as iOS 6.
Google Translate API - Adding Multiple Language Translation Feature to Your Website
Tatoeba Stream #4 - Adding a new language to Tatoeba, with Ricardo
Annoyance: Ubuntu 16.04 Adding a keyboard language.
Adding preprocessor statements to my language
Комментарии