filmov
tv
How to Retrieve a Single API Value in Python Using the Requests Library
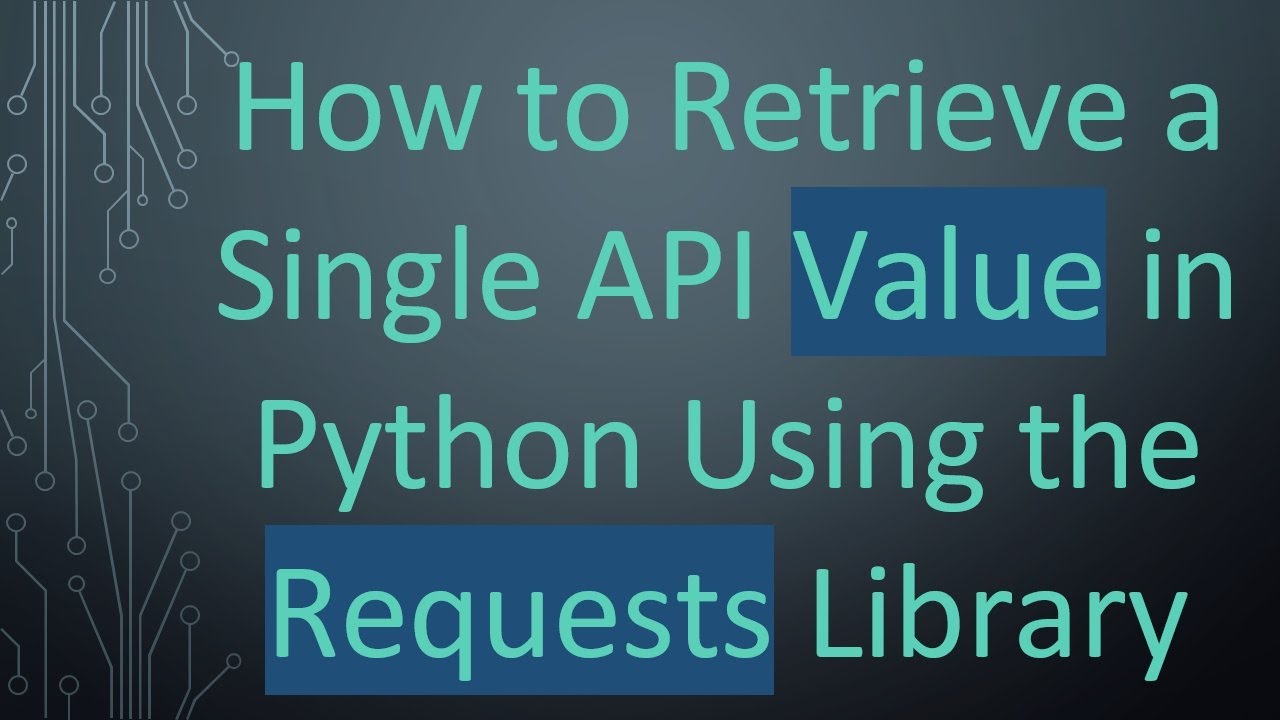
Показать описание
Discover how to extract just the `username` from an API response with Python's Requests library. Step-by-step guide included.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can I have only one api value?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Retrieve a Single API Value in Python Using the Requests Library
When you start working with APIs in Python, it can be a bit overwhelming, especially if you're new to programming. You might find yourself receiving copious amounts of data when you only need a specific piece of information. For instance, after setting up a request to an API, you might get a huge JSON response, but all you really want is a single value like a username. In this post, we'll tackle how to extract just that - the username - from an API response using Python's Requests library.
The Problem
You are likely working on a project where you've started using the Requests library to fetch data from an API. You've set up your API call correctly, but instead of getting just the account name you need, you're bombarded with a lot of irrelevant information. Here's the crux of the issue: you want to simplify your code to get only the username from the API response.
Example Response Data
Take a look at a sample response you might receive from your API call:
[[See Video to Reveal this Text or Code Snippet]]
As seen in the JSON response above, the username field is nested inside multiple layers. Your goal is to retrieve just this single value.
The Solution
To fetch only the username, you'll need to make a couple of simple changes to your existing code. Below, I will guide you through the necessary steps to achieve this.
Step 1: Make the API Request
You start by importing the requests library, defining your user variable, and constructing your API request as you have done previously. Here's the initial portion of your code:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Extract the Username
Now comes the key part - extracting the username from the JSON response. You will need to navigate through the JSON structure to get to the username field. Here's the modification you will make to your code:
[[See Video to Reveal this Text or Code Snippet]]
Full Code Implementation
Here’s how the complete code looks after all the modifications:
[[See Video to Reveal this Text or Code Snippet]]
Summary of the Code Changes
You still send the API request with the same endpoint and payload.
After receiving the JSON response, instead of printing the entire response, you pinpoint the path to the username field.
Finally, you print only the username, which is what you wanted in the first place.
Conclusion
Working with APIs often involves dealing with a lot of data, but with some straightforward adjustments to your code, you can extract only the information you need. In this case, using the Requests library in Python to fetch just the username was a matter of navigating through the JSON structure effectively.
Happy coding and enjoy your programming journey as you explore the world of APIs and data manipulation!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can I have only one api value?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Retrieve a Single API Value in Python Using the Requests Library
When you start working with APIs in Python, it can be a bit overwhelming, especially if you're new to programming. You might find yourself receiving copious amounts of data when you only need a specific piece of information. For instance, after setting up a request to an API, you might get a huge JSON response, but all you really want is a single value like a username. In this post, we'll tackle how to extract just that - the username - from an API response using Python's Requests library.
The Problem
You are likely working on a project where you've started using the Requests library to fetch data from an API. You've set up your API call correctly, but instead of getting just the account name you need, you're bombarded with a lot of irrelevant information. Here's the crux of the issue: you want to simplify your code to get only the username from the API response.
Example Response Data
Take a look at a sample response you might receive from your API call:
[[See Video to Reveal this Text or Code Snippet]]
As seen in the JSON response above, the username field is nested inside multiple layers. Your goal is to retrieve just this single value.
The Solution
To fetch only the username, you'll need to make a couple of simple changes to your existing code. Below, I will guide you through the necessary steps to achieve this.
Step 1: Make the API Request
You start by importing the requests library, defining your user variable, and constructing your API request as you have done previously. Here's the initial portion of your code:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Extract the Username
Now comes the key part - extracting the username from the JSON response. You will need to navigate through the JSON structure to get to the username field. Here's the modification you will make to your code:
[[See Video to Reveal this Text or Code Snippet]]
Full Code Implementation
Here’s how the complete code looks after all the modifications:
[[See Video to Reveal this Text or Code Snippet]]
Summary of the Code Changes
You still send the API request with the same endpoint and payload.
After receiving the JSON response, instead of printing the entire response, you pinpoint the path to the username field.
Finally, you print only the username, which is what you wanted in the first place.
Conclusion
Working with APIs often involves dealing with a lot of data, but with some straightforward adjustments to your code, you can extract only the information you need. In this case, using the Requests library in Python to fetch just the username was a matter of navigating through the JSON structure effectively.
Happy coding and enjoy your programming journey as you explore the world of APIs and data manipulation!