filmov
tv
Implement min stack | Leetcode #155
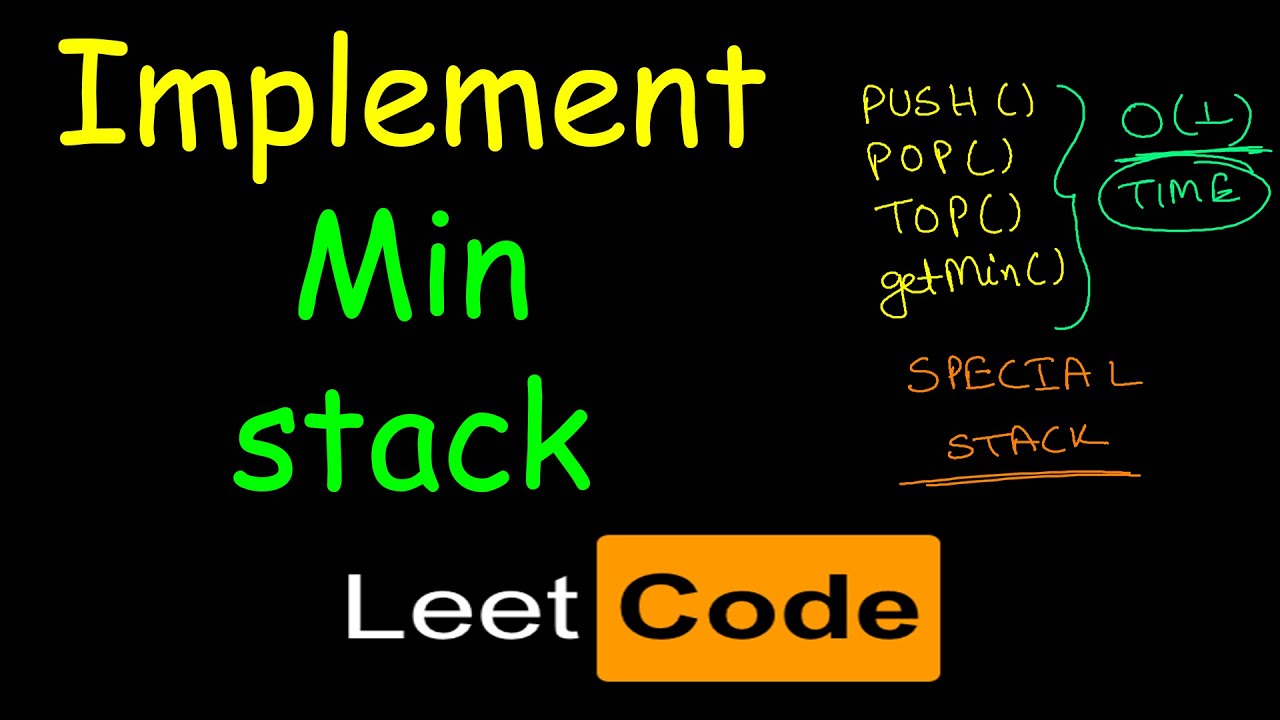
Показать описание
This video explains how to implement a stack with get minimum operation in just O(1) time. This video explains how to implement getmin(), top(), push(), pop() in constant time O(1). You can also implement max stack in the same way as min stack. I have explained using a simple example and have also explained the code for the same. As usual, CODE LINK is present below. If you find any difficulty or have any query then do COMMENT below. PLEASE help our channel by SUBSCRIBING and LIKE our video if you found it helpful...CYA :)
Design Min Stack - Amazon Interview Question - Leetcode 155 - Python
LeetCode 155. Min Stack (Algorithm Explained)
Implement min stack | Leetcode #155
L4. Implement Min Stack | Stack and Queue Playlist
Min Stack - Leetcode 155 - Stacks (Python)
Implement Min Stack | O(2N) and O(N) Space Complexity
Min Stack Leetcode | Get Min at pop | solution stack Hindi Explained | Data structure & Algorith...
Leetcode Grind October - I like to Suffer (Top 50)
LeetCode 155: Min Stack
Min Stack - 155. LeetCode - Java
LeetCode Day 10 - Min Stack C++ Implementation
Min Stack Leetcode | 3 methods/solutions | Code + Explanation
Design special stack with getmin in O(1) time time and O(1) space
Minimum Stack - 1 | Solution
Amazon Coding Interview Question - Min Stack (LeetCode)
Min Stack | Leetcode#155 | Java | Technical Interview | Amazon | Microsoft | Google
LEETCODE 155 (JAVASCRIPT) | MIN STACK
Designing a New DATA STRUCTURE for a Coding Interview! | Min Stack - Leetcode 155
Minimum Stack - 2 | Solution
min stack | min stack leetcode | leetcode 155 | 2 stacl | 1 stack | no stack solutions
Min Stack - Leet Code 155 - TypeScript
LeetCode 155 : Min Stack || MEDIUM || JAVA || Detailed Solution
Min Stack | Leetcode 155 | Live coding session 🔥🔥🔥
Leetcode 155. Min Stack - Python
Комментарии