filmov
tv
Converting Nested Tuples to Lists in Python
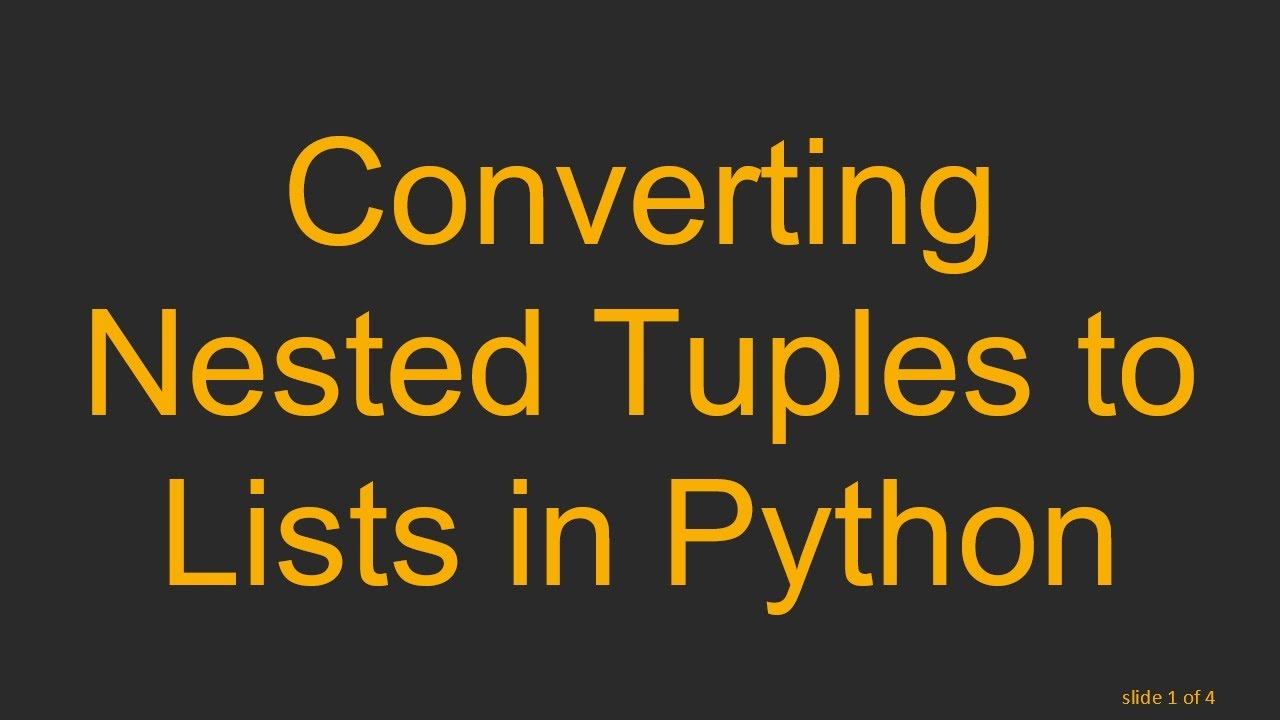
Показать описание
Learn how to efficiently convert nested tuples to lists in Python. Explore examples and understand the nuances of handling nested structures in Python programming.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Converting Nested Tuples to Lists in Python
Tuples and lists are both essential data structures in Python, each with its unique characteristics. Tuples are immutable, ordered collections, while lists are mutable and can be modified. Sometimes, you might encounter nested tuples and find it necessary to convert them into lists for easier manipulation. In this guide, we'll explore different approaches to achieve this conversion.
Understanding Nested Tuples
Nested tuples refer to tuples within tuples, creating a hierarchical structure. For example:
[[See Video to Reveal this Text or Code Snippet]]
Here, nested_tuple contains three inner tuples. If you need to convert this structure into a list, you can employ several methods.
Method 1: Using List Comprehension
List comprehensions provide a concise and readable way to convert nested tuples to lists. Here's an example:
[[See Video to Reveal this Text or Code Snippet]]
In this code snippet, the inner tuples are iterated over, and the list() function is used to convert each inner tuple into a list. The result is stored in nested_list.
Method 2: Map Function
The map() function can also be employed to apply the list() function to each inner tuple:
[[See Video to Reveal this Text or Code Snippet]]
This approach provides a more functional programming style, where the list() function is applied to each element of nested_tuple.
Method 3: Using Iterative Approach
For those who prefer a more traditional iterative approach, a loop can be utilized:
[[See Video to Reveal this Text or Code Snippet]]
This method explicitly iterates over the inner tuples and appends the corresponding lists to nested_list.
Conclusion
Converting nested tuples to lists in Python can be accomplished through various methods, each with its own advantages. List comprehensions, the map() function, and iterative approaches provide flexibility based on your coding style and preference.
Understanding these techniques empowers you to efficiently manipulate nested structures, ensuring your code remains both readable and maintainable.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Converting Nested Tuples to Lists in Python
Tuples and lists are both essential data structures in Python, each with its unique characteristics. Tuples are immutable, ordered collections, while lists are mutable and can be modified. Sometimes, you might encounter nested tuples and find it necessary to convert them into lists for easier manipulation. In this guide, we'll explore different approaches to achieve this conversion.
Understanding Nested Tuples
Nested tuples refer to tuples within tuples, creating a hierarchical structure. For example:
[[See Video to Reveal this Text or Code Snippet]]
Here, nested_tuple contains three inner tuples. If you need to convert this structure into a list, you can employ several methods.
Method 1: Using List Comprehension
List comprehensions provide a concise and readable way to convert nested tuples to lists. Here's an example:
[[See Video to Reveal this Text or Code Snippet]]
In this code snippet, the inner tuples are iterated over, and the list() function is used to convert each inner tuple into a list. The result is stored in nested_list.
Method 2: Map Function
The map() function can also be employed to apply the list() function to each inner tuple:
[[See Video to Reveal this Text or Code Snippet]]
This approach provides a more functional programming style, where the list() function is applied to each element of nested_tuple.
Method 3: Using Iterative Approach
For those who prefer a more traditional iterative approach, a loop can be utilized:
[[See Video to Reveal this Text or Code Snippet]]
This method explicitly iterates over the inner tuples and appends the corresponding lists to nested_list.
Conclusion
Converting nested tuples to lists in Python can be accomplished through various methods, each with its own advantages. List comprehensions, the map() function, and iterative approaches provide flexibility based on your coding style and preference.
Understanding these techniques empowers you to efficiently manipulate nested structures, ensuring your code remains both readable and maintainable.