filmov
tv
Understanding the fprintf Error in C: Debugging Print Issues While Calling Functions
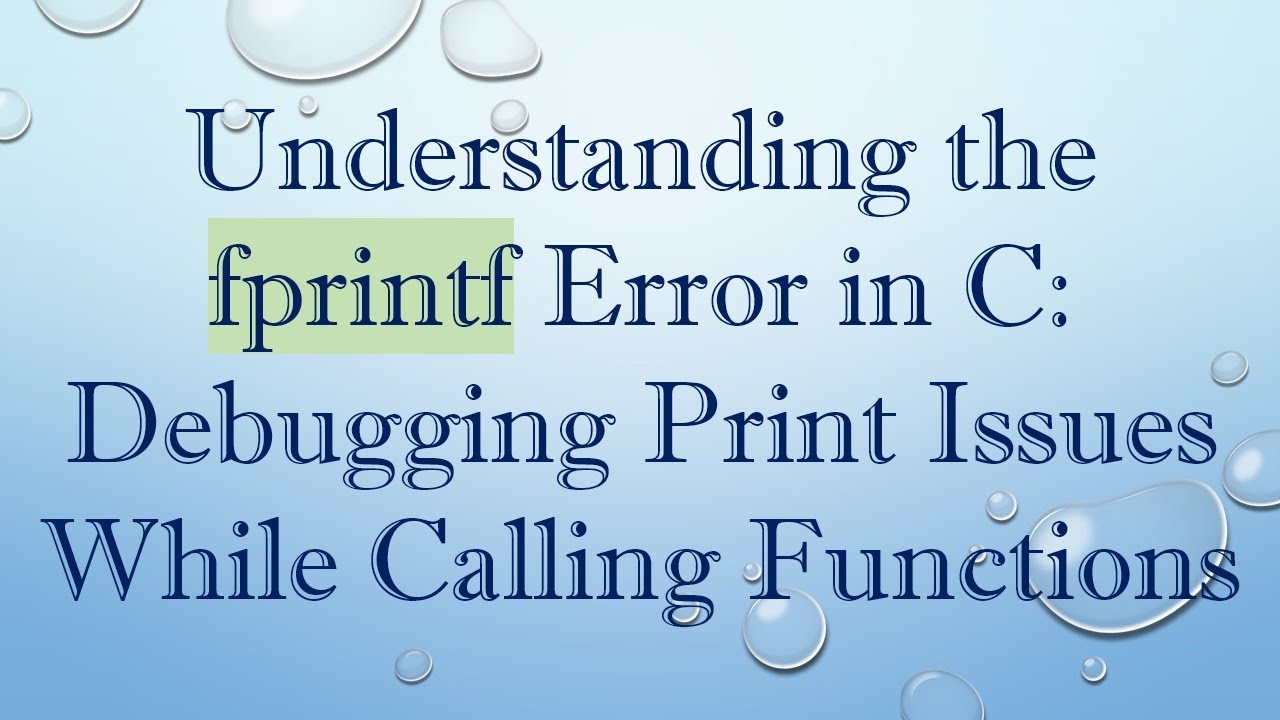
Показать описание
Discover how to resolve issues with `fprintf` in C programming when calling functions. This post breaks down the problem and provides a step-by-step solution for better debugging.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Calling a function causes error with "fprintf"
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the fprintf Error in C Programming
When programming in C, encountering errors when calling functions can be frustrating—especially when it leads to issues with output functions like fprintf. In this guide, we will address a common scenario where using fprintf to write to an output file doesn't work as expected after calling certain functions. We will explore the problem, dissect the code, and provide you with a clear solution to fix it.
The Issue at Hand
In the provided code, we see a scenario where the fprintf function operates correctly, outputting a message to a file when certain functions are not called. However, once any of those functions—InitBoardRand, InitBoardChecker, or InitBoardAllWhite—are invoked, the fprintf fails to work. This presents a puzzling dilemma for many developers who may wonder:
Why does calling a function after the fprintf statement affect its ability to print to the output file?
Key Observations
The fprintf statement works correctly if the function calls are commented out.
If the functions are uncommented, nothing gets printed to the output file, even though the program runs without crashing.
The error seems related to pointer allocation or memory management.
Breakdown of the Code
Let’s examine the relevant sections of the code.
Opening the Output File:
The program attempts to open an output file after reading its name from another input file.
If the file can't be opened, an error is printed to stderr, and the function returns early.
Memory Allocation for 2-D Array:
Memory is allocated for a two-dimensional array of integers.
Errors in memory allocation result in error messages being printed to both stderr and the output file.
Function Calls:
Upon reaching the block containing the function calls, consider what these functions do with boardpp. If any of these functions alter the memory pointed to by boardpp or affect the output file pointer in some way, it could lead to unexpected behavior.
Solution: Correcting the Memory Allocation
The key problem likely arises from incorrect memory allocation of the 2-D array. This line:
[[See Video to Reveal this Text or Code Snippet]]
should be corrected to:
[[See Video to Reveal this Text or Code Snippet]]
Why the Change Matters
The initial code allocates memory incorrectly, leading to potential corruption of pointer values.
By allocating sizeof(int*), you ensure that each row in the 2-D array can correctly point to its own dynamically allocated memory.
Summary
In summary, the error you're encountering with fprintf not working after function calls is likely linked to incorrect memory allocation of the pointer for the 2-D array. Ensuring that your memory allocation matches the expected size for what you're trying to achieve is crucial in C programming. Always double-check your pointer assignments and memory management practices to prevent issues like this in the future.
By understanding and implementing these corrections, you can enhance your program's reliability and functionality. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Calling a function causes error with "fprintf"
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the fprintf Error in C Programming
When programming in C, encountering errors when calling functions can be frustrating—especially when it leads to issues with output functions like fprintf. In this guide, we will address a common scenario where using fprintf to write to an output file doesn't work as expected after calling certain functions. We will explore the problem, dissect the code, and provide you with a clear solution to fix it.
The Issue at Hand
In the provided code, we see a scenario where the fprintf function operates correctly, outputting a message to a file when certain functions are not called. However, once any of those functions—InitBoardRand, InitBoardChecker, or InitBoardAllWhite—are invoked, the fprintf fails to work. This presents a puzzling dilemma for many developers who may wonder:
Why does calling a function after the fprintf statement affect its ability to print to the output file?
Key Observations
The fprintf statement works correctly if the function calls are commented out.
If the functions are uncommented, nothing gets printed to the output file, even though the program runs without crashing.
The error seems related to pointer allocation or memory management.
Breakdown of the Code
Let’s examine the relevant sections of the code.
Opening the Output File:
The program attempts to open an output file after reading its name from another input file.
If the file can't be opened, an error is printed to stderr, and the function returns early.
Memory Allocation for 2-D Array:
Memory is allocated for a two-dimensional array of integers.
Errors in memory allocation result in error messages being printed to both stderr and the output file.
Function Calls:
Upon reaching the block containing the function calls, consider what these functions do with boardpp. If any of these functions alter the memory pointed to by boardpp or affect the output file pointer in some way, it could lead to unexpected behavior.
Solution: Correcting the Memory Allocation
The key problem likely arises from incorrect memory allocation of the 2-D array. This line:
[[See Video to Reveal this Text or Code Snippet]]
should be corrected to:
[[See Video to Reveal this Text or Code Snippet]]
Why the Change Matters
The initial code allocates memory incorrectly, leading to potential corruption of pointer values.
By allocating sizeof(int*), you ensure that each row in the 2-D array can correctly point to its own dynamically allocated memory.
Summary
In summary, the error you're encountering with fprintf not working after function calls is likely linked to incorrect memory allocation of the pointer for the 2-D array. Ensuring that your memory allocation matches the expected size for what you're trying to achieve is crucial in C programming. Always double-check your pointer assignments and memory management practices to prevent issues like this in the future.
By understanding and implementing these corrections, you can enhance your program's reliability and functionality. Happy coding!