filmov
tv
Writing a Shader in OpenGL
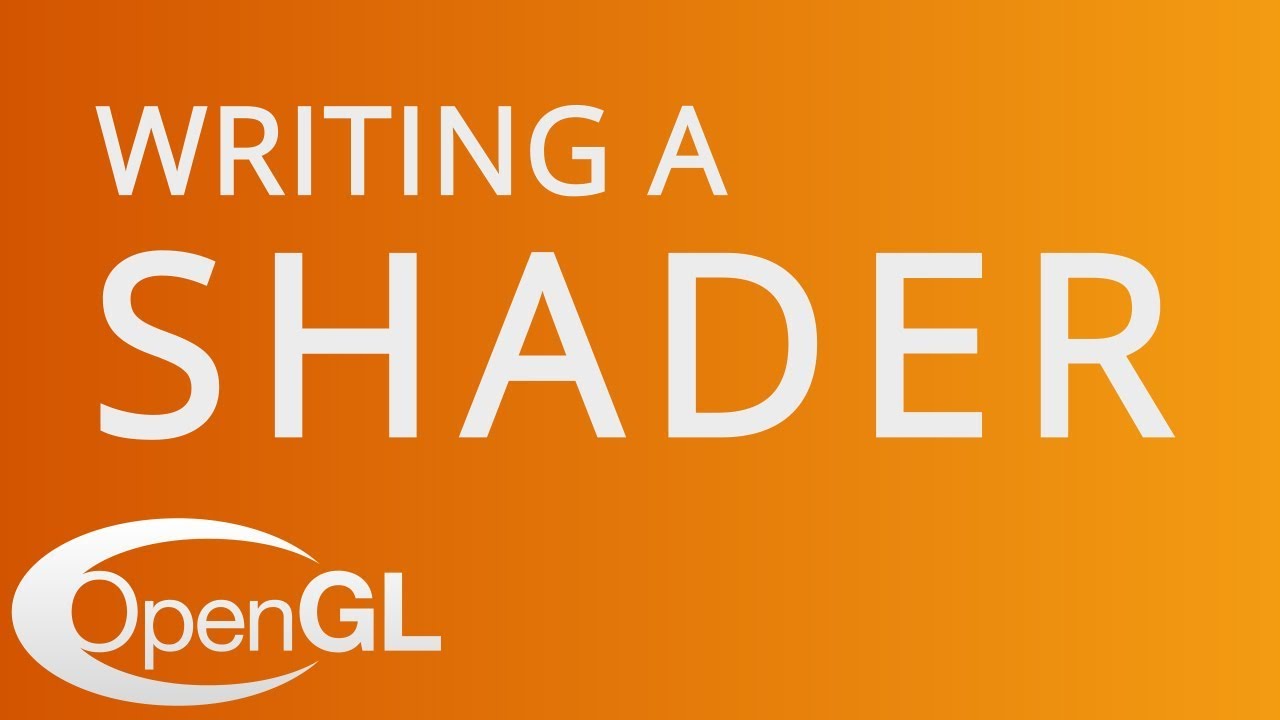
Показать описание
Thank you to the following Patreon supporters:
- Samuel Egger
- Dominic Pace
- Kevin Gregory Agwaze
- Sébastien Bervoets
- Tobias Humig
Gear I use:
-----------------
Writing a Shader in OpenGL
How Shaders Work (in OpenGL) | How to Code Minecraft Ep. 3
How I Deal with Shaders in OpenGL
How Shaders Work in OpenGL
Tutorial 3 - Introduction to OpenGL Shaders
Modern OpenGL Tutorial - Compute Shaders
Tutorial 08 - Creating Simple OpenGL Shaders
OpenGL with C++ 2: Writing our First Shader
FXGL 25 Tutorial: Shaders
7 Examples Proving Shaders are Amazing
OpenGL Tunnel Effect Shader in GLSL
Recreating Winston's shield in OpenGL/C++ | Intersection Shaders
OpenGL Shader Hello World
02 01 Vertex and Fragment Shaders in OpenGL
OpenGL Writing GLSL Shaders in Text Files vs String Literals
Simple C++ OpenGL Shader Editor
Online Graphics OpenGL 1: Shaders
OpenGL Shader Examples
OpenGL Tutorial 20 - Geometry Shader
OpenGL Ep7 | The Realm Of Shader Possibilities | How-to Apply ANY Fragment (or Pixel) Shader
Shader Basics, Blending & Textures • Shaders for Game Devs [Part 1]
Writing GLSL Shaders | OpenGL 3D Renderer #2
OpenGL Tutorial 5 - Shaders
OpenGL Shaders | Game Engine series
Комментарии