filmov
tv
How to Set a Conditional Value Inside an Object in JavaScript
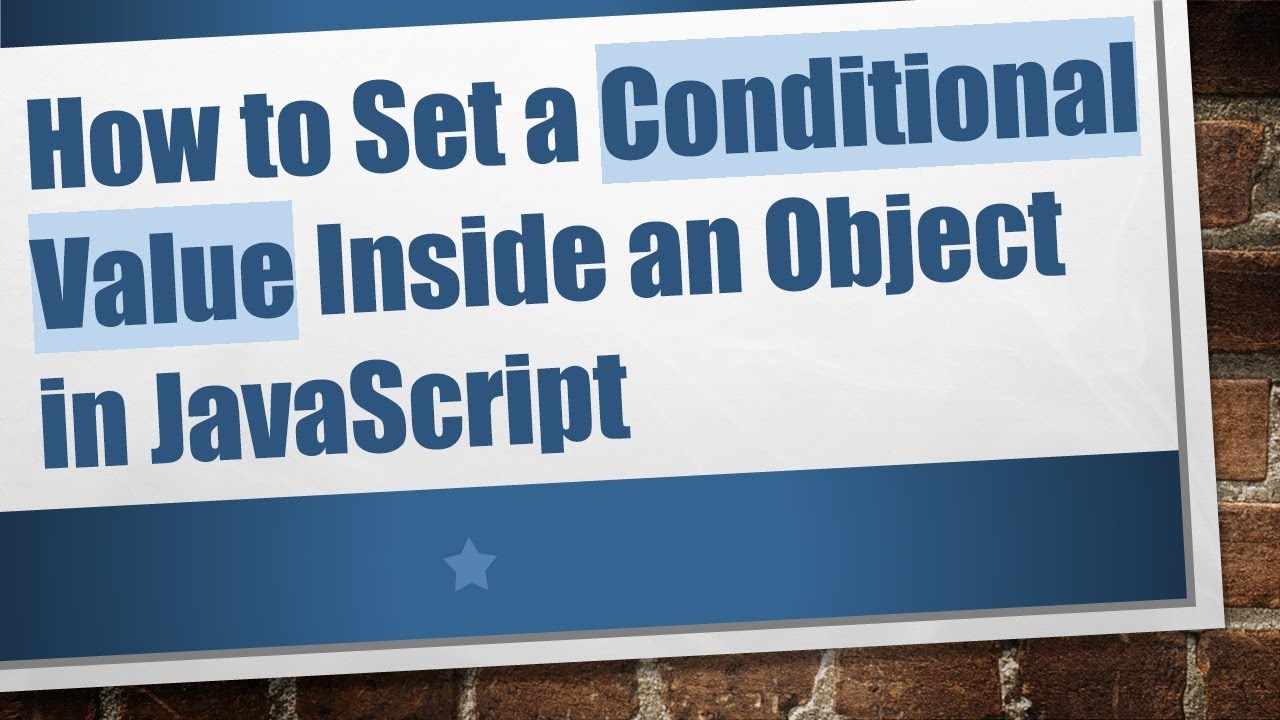
Показать описание
Discover effective methods to set a conditional value inside of an object in JavaScript, simplifying your code and avoiding compilation issues.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to set conditional value inside of an object?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Set a Conditional Value Inside an Object in JavaScript
When programming in JavaScript, developers often face the challenge of managing state effectively, especially when incorporating conditionals. This is particularly true when dealing with objects. If you're trying to set a conditional value inside an object and your code refuses to compile, don’t worry! In this guide, we will explain how to properly inject a conditional value as a value to a dynamic key in your state object.
The Problem
You might be trying to dynamically assign values within an object based on specific conditions. For instance, you need to set different values when value is equal to 'foo' compared to when it is not. However, you may find your attempts falling short, resulting in compilation errors.
Example Code Falling Short
Consider the following examples that don’t work as intended:
[[See Video to Reveal this Text or Code Snippet]]
This code is a good starting point but may feel cumbersome and repetitive. Let's look at the non-working attempts:
[[See Video to Reveal this Text or Code Snippet]]
[[See Video to Reveal this Text or Code Snippet]]
Both attempts utilize incorrect syntax for setting conditional values leading to compilation errors.
The Solution
To efficiently manage conditional values in JavaScript objects, there's a more concise and effective approach.
Simplified Code to Set Conditional Values
Use the following structure:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Spread Operator:
The ...inicialErrState spreads the previous state into the new object, ensuring that all existing properties are retained.
Conditional Logic:
The expression value === 'foo' ? { [value]: true } : { fixedValue: [true, ''] } acts as a decision-maker.
If value is 'foo', a key will be created with the name provided by value, and it's assigned true.
If value is not 'foo', it sets the fixedValue key to an array containing [true, ''].
Benefits of This Approach
Conciseness: The solution is more concise and readable.
Maintainability: With this pattern, it's easier to manage changes or expand functionality.
Less Error-Prone: Reduces the risk of mistakes by minimizing repeated code.
Conclusion
In conclusion, understanding how to set conditional values inside an object in JavaScript can dramatically improve your code's readability and maintainability. By utilizing the structure shown above, you transition toward a clearer, more functional style of programming, ensuring your code compiles smoothly without unnecessary duplication.
Now you're equipped to tackle similar problems in your coding journey with confidence. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to set conditional value inside of an object?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Set a Conditional Value Inside an Object in JavaScript
When programming in JavaScript, developers often face the challenge of managing state effectively, especially when incorporating conditionals. This is particularly true when dealing with objects. If you're trying to set a conditional value inside an object and your code refuses to compile, don’t worry! In this guide, we will explain how to properly inject a conditional value as a value to a dynamic key in your state object.
The Problem
You might be trying to dynamically assign values within an object based on specific conditions. For instance, you need to set different values when value is equal to 'foo' compared to when it is not. However, you may find your attempts falling short, resulting in compilation errors.
Example Code Falling Short
Consider the following examples that don’t work as intended:
[[See Video to Reveal this Text or Code Snippet]]
This code is a good starting point but may feel cumbersome and repetitive. Let's look at the non-working attempts:
[[See Video to Reveal this Text or Code Snippet]]
[[See Video to Reveal this Text or Code Snippet]]
Both attempts utilize incorrect syntax for setting conditional values leading to compilation errors.
The Solution
To efficiently manage conditional values in JavaScript objects, there's a more concise and effective approach.
Simplified Code to Set Conditional Values
Use the following structure:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Spread Operator:
The ...inicialErrState spreads the previous state into the new object, ensuring that all existing properties are retained.
Conditional Logic:
The expression value === 'foo' ? { [value]: true } : { fixedValue: [true, ''] } acts as a decision-maker.
If value is 'foo', a key will be created with the name provided by value, and it's assigned true.
If value is not 'foo', it sets the fixedValue key to an array containing [true, ''].
Benefits of This Approach
Conciseness: The solution is more concise and readable.
Maintainability: With this pattern, it's easier to manage changes or expand functionality.
Less Error-Prone: Reduces the risk of mistakes by minimizing repeated code.
Conclusion
In conclusion, understanding how to set conditional values inside an object in JavaScript can dramatically improve your code's readability and maintainability. By utilizing the structure shown above, you transition toward a clearer, more functional style of programming, ensuring your code compiles smoothly without unnecessary duplication.
Now you're equipped to tackle similar problems in your coding journey with confidence. Happy coding!