filmov
tv
C Programming Tutorial - 41 - Sorting Arrays
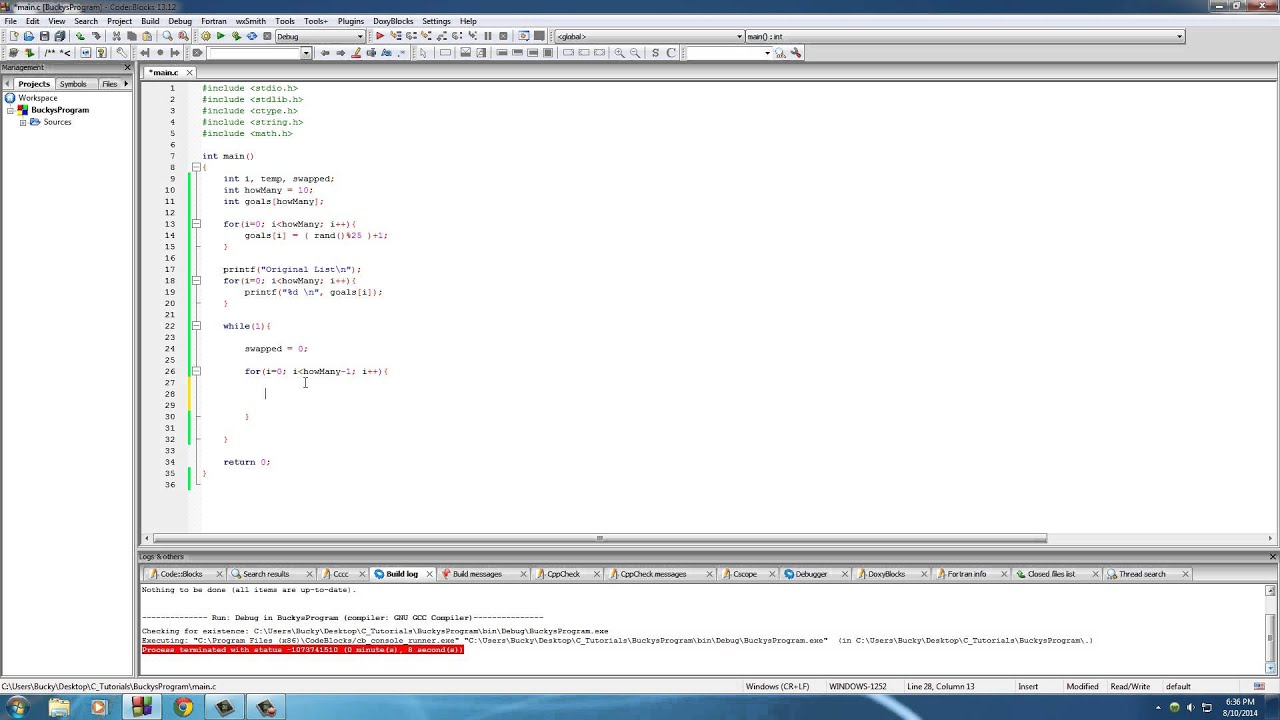
Показать описание
C Programming Tutorial - 41 - Sorting Arrays
C Programming Tutorial 41 Sorting Arrays
C Programming Tutorial 41 - Quiz 1
C Programming Tutorial - 41: The Continue Statement
C Programming All-in-One Tutorial Series (10 HOURS!)
C Programming Tutorial - 41 - Return Values
C Programming Tutorial 41 (Sorting Arrays)
C Programming Tutorial 41, Unions
Blueprint41 C# ORM for Graph Databases part 2
C Programming Tutorial 41 Sorting Arrays HD
C Programming Tutorial 41 Sorting Arrays
C Programming Tutorial for Beginners
C Programming Tutorial 41 Unions
C Programming Tutorial 41 - fscanf() - feof() - Reading From A File - Part 1
C Programming Tutorial 41 Sorting Arraysvia torchbrowser com
C Programming Tutorial 41 Sorting Arrays
C Programming Tutorial # 41 - fscanf() - feof() - Reading From A File - Part 1 [HD]
C++ Programming Tutorial 41 - Conditional Operator (Ternary Operator)
#41🖥🖲C Programming Exercise💻 C Language Tutorial👨🏫📂📓#shorts #coding
C Programming Tutorial - 41 - String Length
C Operator Precedence & Associativity - C Programming Tutorial 41
C_41 Break statement in C | C Language Tutorials
C Programming Tutorial # 41 fscanf feof Reading From A File Part 1 HD
C Programming Basics : Learn C Fundamentals by Coding I PART-41 while loops
Комментарии