filmov
tv
Data Structures in Python: Singly Linked Lists -- Merge Two Sorted Lists
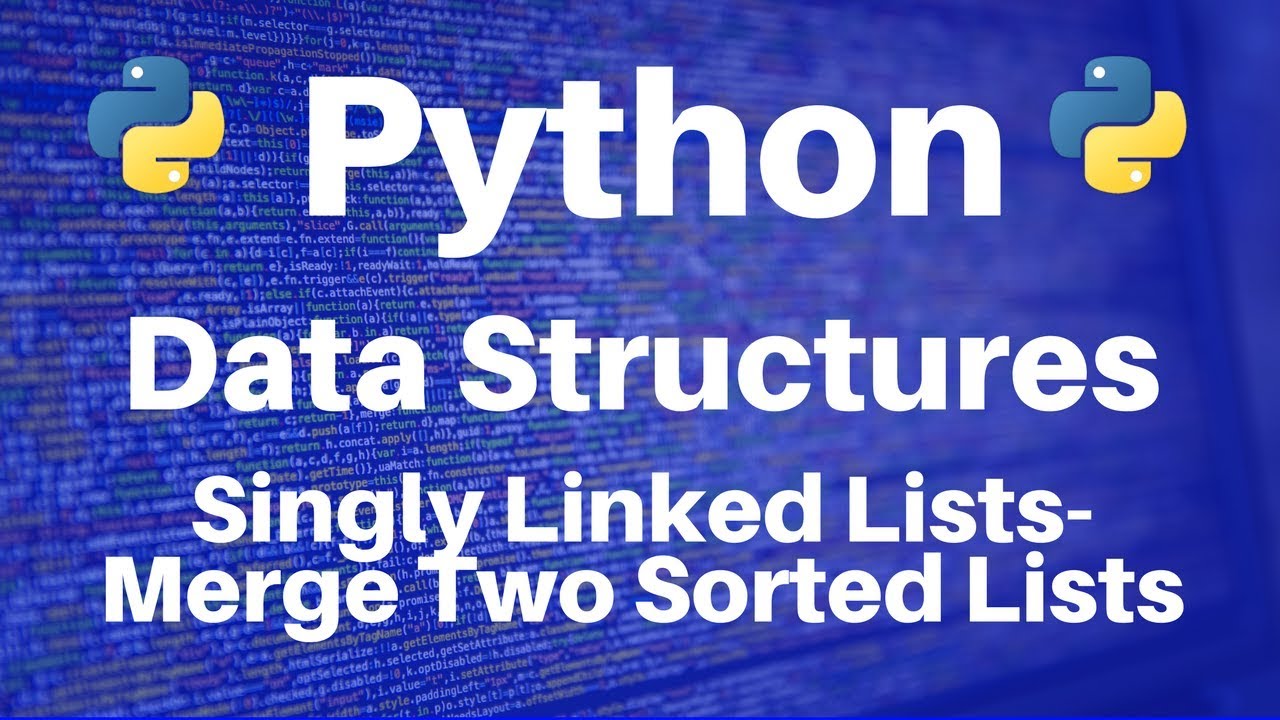
Показать описание
In this video, we investigate how to merge two sorted singly linked lists. We first cover the general approach for how we will go about performing this action. We then implement our algorithm in Python.
The software written in this video is available at:
Do you like the development environment I'm using in this video? It's a customized version of vim that's enhanced for Python development. If you want to see how I set up my vim, I have a series on this here:
If you've found this video helpful and want to stay up-to-date with the latest videos posted on this channel, please subscribe:
The software written in this video is available at:
Do you like the development environment I'm using in this video? It's a customized version of vim that's enhanced for Python development. If you want to see how I set up my vim, I have a series on this here:
If you've found this video helpful and want to stay up-to-date with the latest videos posted on this channel, please subscribe:
Data Structures in Python: Singly Linked Lists -- Length
Data Structures in Python: Singly Linked Lists -- Insertion
Linked List - Data Structures & Algorithms Tutorials in Python #4
Python: Linked Lists (fast)
This Algorithm is SUPER HELPFUL for Coding Interviews! | Fast & Slow Pointers for Linked Lists
2 Simple Ways To Code Linked Lists In Python
Python Data Structures #2: Linked List
Python Tutorials - Singly Linked List | Program | Part 1
Linked List Part 1 Data Structure Explained | Types, Operations & Implementation
The Majestic Battle of Circular Linked Lists! 🔁💥
Recursive Data Structure in Python - Singly Linked List Introductory Tutorial
How Insertion in Linked List Works ? 🤔😏
Linked Lists - Singly & Doubly Linked - DSA Course in Python Lecture 3
Introduction to Linked List
python create linked list
Data Structures in Python: Singly Linked Lists -- Rotate
Linked lists in 4 minutes
Learn Linked Lists in 13 minutes 🔗
Linked List - Data Structures in Python #1
Circular queue in data structure #coding #programming #trending #shorts
Data Structures in Python: Singly Linked Lists -- Merge Two Sorted Lists
4 Leetcode Mistakes
Data Structures in Python: Singly Linked Lists -- Sum Two Lists
Data Structures: Singly Linked List in Python 3 (Part 1/10)
Комментарии