filmov
tv
Arduino Bipolar Stepper Motor Control | Electronics Developer
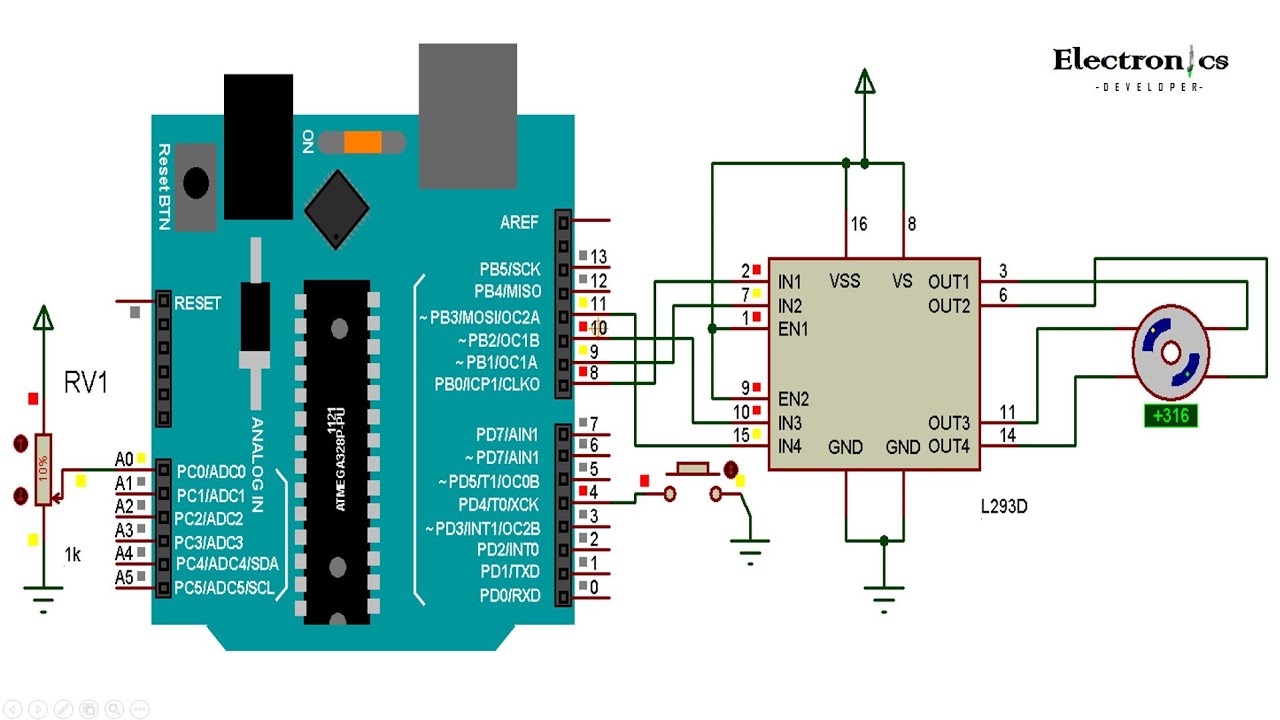
Показать описание
-------------------------
📌Introduction
-------------------------
This small post shows how to control speed and direction of rotation of bipolar stepper motor using Arduino UNO board and L293D motor driver chip.
The stepper motor used in this example is just a PC CD-ROM (or DVD-ROM) drive which has 4 wires.
Proteus simulation of the example is provided at the end of the topic.
Basically there are two types of stepper motors: bipolar and unipolar. The bipolar stepper motor is a two-phase brushless motor which has two coils (windings), this motor has 4 wires (2 wires for each coil).
The other type is the unipolar stepper motor, it is 4-phase brushless motor which has 5 or 6 wires.The popular controlling modes of of the stepper motor are: full step and half step. The full step can be divided into 2 types: one-phase and two-phase.In full step one-phase mode the driver energizes one coil at a time. This type of controlling requires the least amount of power but provides the least torque.
In full step two-phase mode the driver energizes the two coils at the same time. This mode provides the highest torque but it requires twice as much power as one-phase mode.
Half step mode is a combination of the two full step modes (one-phase and two-phase). This mode increases accuracy by dividing each step by 2. it requires power in-between one-phase and two-phase modes, torque also is in-between.
There is another controlling type called microstepping, this type is more accurate than the half step mode, it requires two sinusoidal current sources with 90° shift.
-------------------------
📌 Circuit Design
-------------------------
All grounded terminals are connected together.
The L293D chip has 16 pins with 4 inputs (IN1, IN2, IN3 and IN4) and 4 outputs (OUT1, OUT2, OUT3 and OUT4). The 4 outputs are connected to the bipolar stepper motor as shown in the circuit diagram.
The 4 inputs are connected as follows:
IN1 to Arduino pin 8
IN2 to Arduino pin 9
IN3 to Arduino pin 10
IN4 to Arduino pin 11
The L293D has 2 VCC pins: VCC1 (pin #16) and VCC2 (pin #8). VCC1 is connected to Arduino +5V pin. VCC2 is connected to another power source (positive terminal) with voltage equal to motor nominal voltage, it’s labeled in the circuit diagram as V_Motor (V_Motor = motor voltage). So if we have a stepper motor with nominal voltage of 5V we’ve to connect VCC2 to +5V (Arduino 5V output shouldn’t be used) and if the stepper motor nominal voltage is 12V we’ve to connect VCC2 to +12V (negative terminal of this source is connected to circuit ground)…
The 10k ohm potentiometer is used to control the speed of the stepper motor, its output pin is connected to Arduino analog pin 0.
The push button which is connected to Arduino pin 4 is used to change the rotation direction of the stepper motor.
-------------------------
📌 Tags
-------------------------
#Arduino #stepper_motor #electronics
-------------------------
📌 The Code
-------------------------
📌 Tune With us so you never miss any update
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Copyright Disclaimer under Section 107 of the copyright act 1976
--------------------------------------------------------------------------------------------------------------------------------------------------------------
Copyright Disclaimer under Section 107 of the copyright act 1976, allowance is made for fair use for purposes such as criticism, comment, news reporting, scholarship, and research. Fair use is a use permitted by copyright statute that might otherwise be infringing. Non-profit, educational or personal use tips the balance in favour of fair use.
---------------------------------------------------------------------------------------------------------------------------------------------------------------
About Electronics Developer : YouTube certified creative essentials and asset monetization
---------------------------------------------------------------------------------------------------------------------------------------------------------------
📌Introduction
-------------------------
This small post shows how to control speed and direction of rotation of bipolar stepper motor using Arduino UNO board and L293D motor driver chip.
The stepper motor used in this example is just a PC CD-ROM (or DVD-ROM) drive which has 4 wires.
Proteus simulation of the example is provided at the end of the topic.
Basically there are two types of stepper motors: bipolar and unipolar. The bipolar stepper motor is a two-phase brushless motor which has two coils (windings), this motor has 4 wires (2 wires for each coil).
The other type is the unipolar stepper motor, it is 4-phase brushless motor which has 5 or 6 wires.The popular controlling modes of of the stepper motor are: full step and half step. The full step can be divided into 2 types: one-phase and two-phase.In full step one-phase mode the driver energizes one coil at a time. This type of controlling requires the least amount of power but provides the least torque.
In full step two-phase mode the driver energizes the two coils at the same time. This mode provides the highest torque but it requires twice as much power as one-phase mode.
Half step mode is a combination of the two full step modes (one-phase and two-phase). This mode increases accuracy by dividing each step by 2. it requires power in-between one-phase and two-phase modes, torque also is in-between.
There is another controlling type called microstepping, this type is more accurate than the half step mode, it requires two sinusoidal current sources with 90° shift.
-------------------------
📌 Circuit Design
-------------------------
All grounded terminals are connected together.
The L293D chip has 16 pins with 4 inputs (IN1, IN2, IN3 and IN4) and 4 outputs (OUT1, OUT2, OUT3 and OUT4). The 4 outputs are connected to the bipolar stepper motor as shown in the circuit diagram.
The 4 inputs are connected as follows:
IN1 to Arduino pin 8
IN2 to Arduino pin 9
IN3 to Arduino pin 10
IN4 to Arduino pin 11
The L293D has 2 VCC pins: VCC1 (pin #16) and VCC2 (pin #8). VCC1 is connected to Arduino +5V pin. VCC2 is connected to another power source (positive terminal) with voltage equal to motor nominal voltage, it’s labeled in the circuit diagram as V_Motor (V_Motor = motor voltage). So if we have a stepper motor with nominal voltage of 5V we’ve to connect VCC2 to +5V (Arduino 5V output shouldn’t be used) and if the stepper motor nominal voltage is 12V we’ve to connect VCC2 to +12V (negative terminal of this source is connected to circuit ground)…
The 10k ohm potentiometer is used to control the speed of the stepper motor, its output pin is connected to Arduino analog pin 0.
The push button which is connected to Arduino pin 4 is used to change the rotation direction of the stepper motor.
-------------------------
📌 Tags
-------------------------
#Arduino #stepper_motor #electronics
-------------------------
📌 The Code
-------------------------
📌 Tune With us so you never miss any update
-------------------------------------------------------------------------------------------------------------------------------------------------------------
Copyright Disclaimer under Section 107 of the copyright act 1976
--------------------------------------------------------------------------------------------------------------------------------------------------------------
Copyright Disclaimer under Section 107 of the copyright act 1976, allowance is made for fair use for purposes such as criticism, comment, news reporting, scholarship, and research. Fair use is a use permitted by copyright statute that might otherwise be infringing. Non-profit, educational or personal use tips the balance in favour of fair use.
---------------------------------------------------------------------------------------------------------------------------------------------------------------
About Electronics Developer : YouTube certified creative essentials and asset monetization
---------------------------------------------------------------------------------------------------------------------------------------------------------------
Комментарии