filmov
tv
One Problem, Five Programming Languages LIVE!
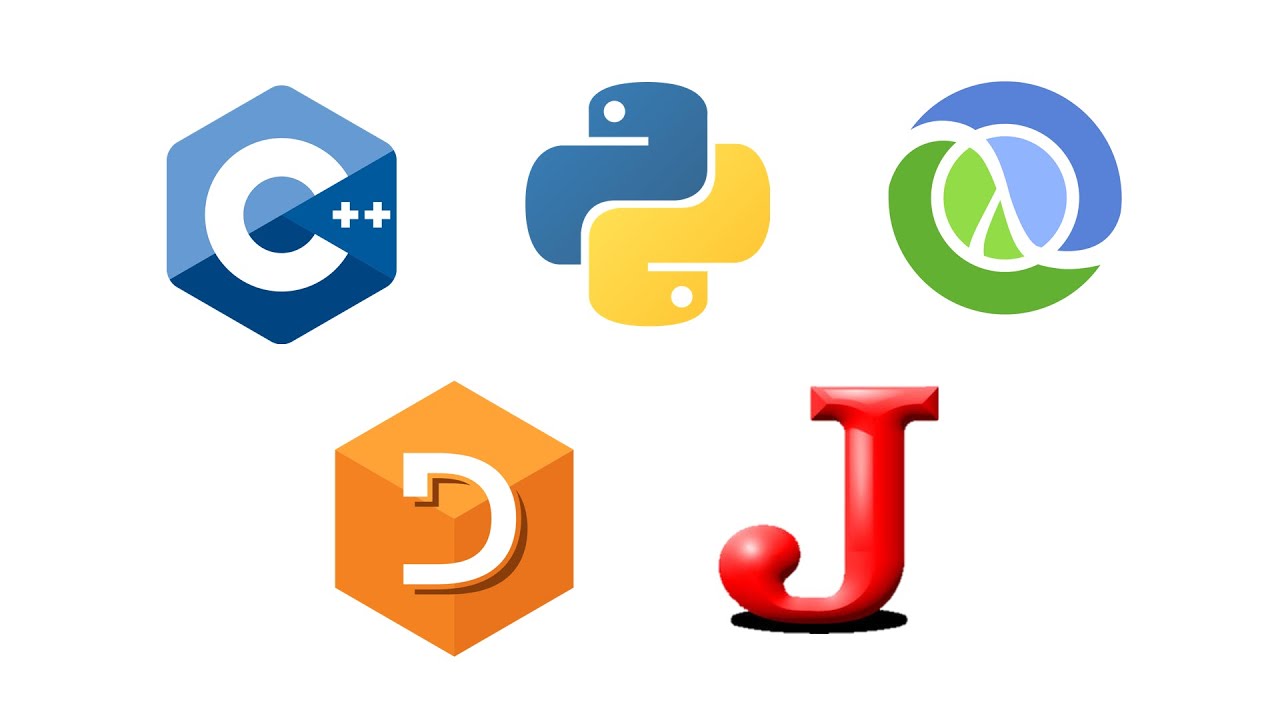
Показать описание
We will solve one programming problem in multiple programming languages (C++, APL, J, Clojure, Python & maybe more)
One Problem, Five Programming Languages LIVE!
The 5 most HATED programming languages 👩💻 #programming #technology #software #career
1 Problem, 16 Programming Languages (C++ vs Rust vs Haskell vs Python vs APL...)
STOP Learning These Programming Languages (for Beginners)
Top 5 Fastest Programming Languages: Rust, C++, Swift, Java, and 90 more compared!
Coding for 1 Month Versus 1 Year #shorts #coding
5 Math Skills Every Programmer Needs
Comment yes for more body language videos! #selfhelp #personaldevelopment #selfimprovement
Computational Thinking and Programming - Course Overview by Ms. D Naga Sudha
Coding - Expectation vs Reality | Programming - Expectation vs Reality | Codeiyapa #Shorts
Programming vs Coding - What's the difference?
Top 5 Most Loved Programming Languages 👩💻 #programming #technology #software #career
My Brain after 569 Leetcode Problems
Learn Five (5) Programming Languages in One Course | Chapter 09
3 Tips I’ve learned after 2000 hours of Leetcode
Types of Errors in Programming
How to Learn to Code - 8 Hard Truths
Why so many Coding Languages are created?
Junior Developer v/s Senior Developer😛 #shorts #funny
Learn Five (5) Programming Languages in One Course | Chapter 04
[Mike's Advice] The Five Programming [Languages/Paradigms/Styles] You Should Explore
Learn Five (5) Programming Languages in One Course | Chapter 06
The Generations of Programming Languages | Computer Science History
Learn Five (5) Programming Languages in One Course | Chapter 08
Комментарии