filmov
tv
Angular Firebase CRUD App with NoSQL Database Tutorial
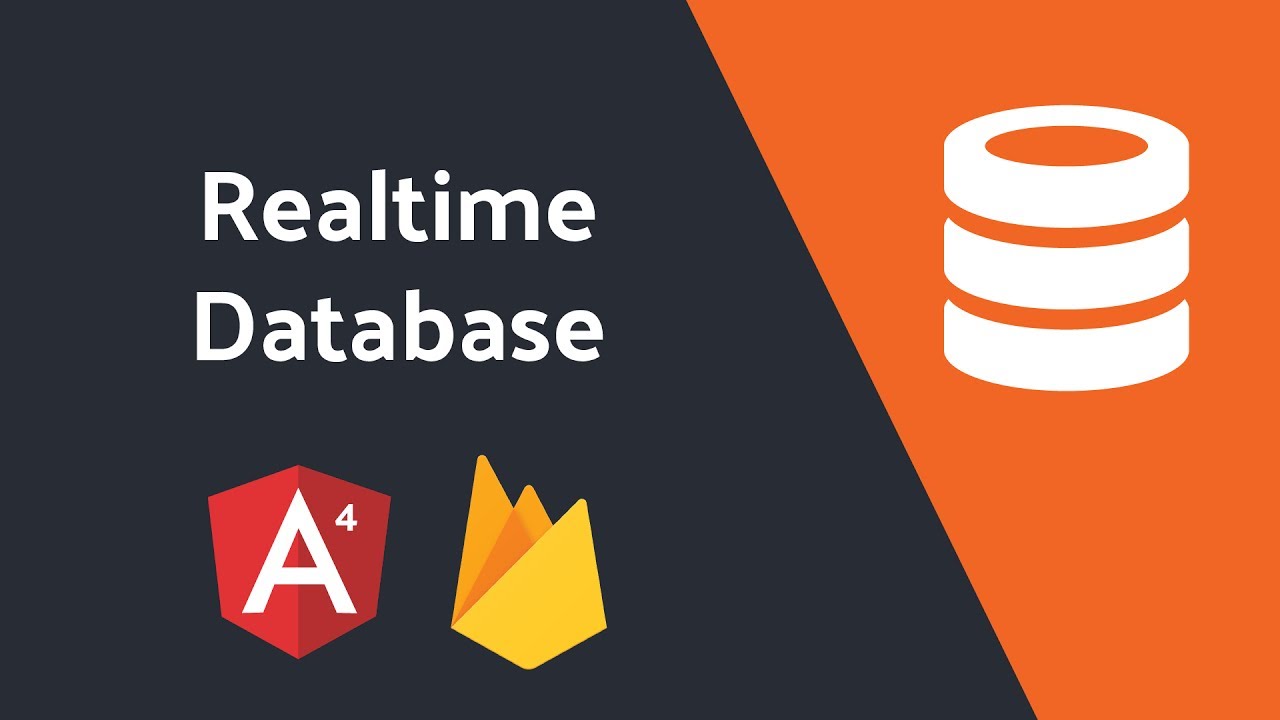
Показать описание
In this episode we're going to build todo list feature that performs the SQL-style CRUD operations of create, read, update, and delete. NoSQL use different terms for these operations, so heres a basic translation when working with Firebase.
create -- push
read -- list or object
update -- update or set
delete -- remove
Angular apps work with asynchronous data streams using RxJS reactive extensions for JavaScript. A data stream can be anything, but in this context we are talking about objects from the Firebase realtime database.
At this point, it is assumed you have the AngularFire2 package installed and bootstrapped into your app. If not, go to the docs or checkout this video.
Step 1 - Generate the Files
Start by generating the necessary files from the command line. The feature is a simple list of todo items that can be modified by users.
ng g service items/shared/item
ng g class items/shared/item
ng g component items/items-list
ng g component items/item-detail
ng g component items/item-form
Step 2 - Define an Item Class
We will give Items their own TypeScript class to keep our data organized.
Step 3 - Building the Service
All the CRUD magic happens in the service, which performs six different operations.
1. Get a list of items
2. Get a single item
3. Create a new item
4. Update an existing item
5. Delete a single item
6. Delete an entire list of items
Let's start by importing dependencies and declaring public variables.
When we get an item or list of items, they will returned as an observable, which can then be unwrapped asynchronously in a component. The functions for getting an item or list will return the observable so it can be set as a public variable.
All other functions will return void because they operate on the items variable or take a specific key as an argument. Any component watching the items variable will be automatically updated when these functions are called.
All of these operations are defined in the AngularFire2 docs, so I recommend you check those out.
Step 4 - Build the List Component
The list component will serve as a parent component that iterates over items in the database. First, we need to define a public items variable during the NgOnInit lifecycle hook. This variable holds the Firebase List observable.
Then we use the ngFor directive with the async pipe to iterate over the observable. The async pipe is critical because the observable will be empty on initial rendering, so we need to tell angular to handle it differently than a regular JavaScript object.
Step 5 - Build the Item Detail Component
In the template itself, we display the item attributes and add the CRUD functions to the cooresponding buttons.
Step 6 - Build the Item Form Component
There are several different form validation methods in Angular. In this example, I am going to use a template driven approach using the if-then-else else syntax introduced in Angular 4. This method is only ideal for small simple forms because all the logic will be defined in the HTML.
We define two different templates named valid and errors. We can then display these templates conditionally based on the form state.
The final step is to submit the form to create a new item. In this case, I just use the click event to trigger the `createItem` method from the service.
That's all for Firebase CRUD, see you next time.
Комментарии