filmov
tv
Understanding the AttributeError: Fixing Python Code for Test Score Averages
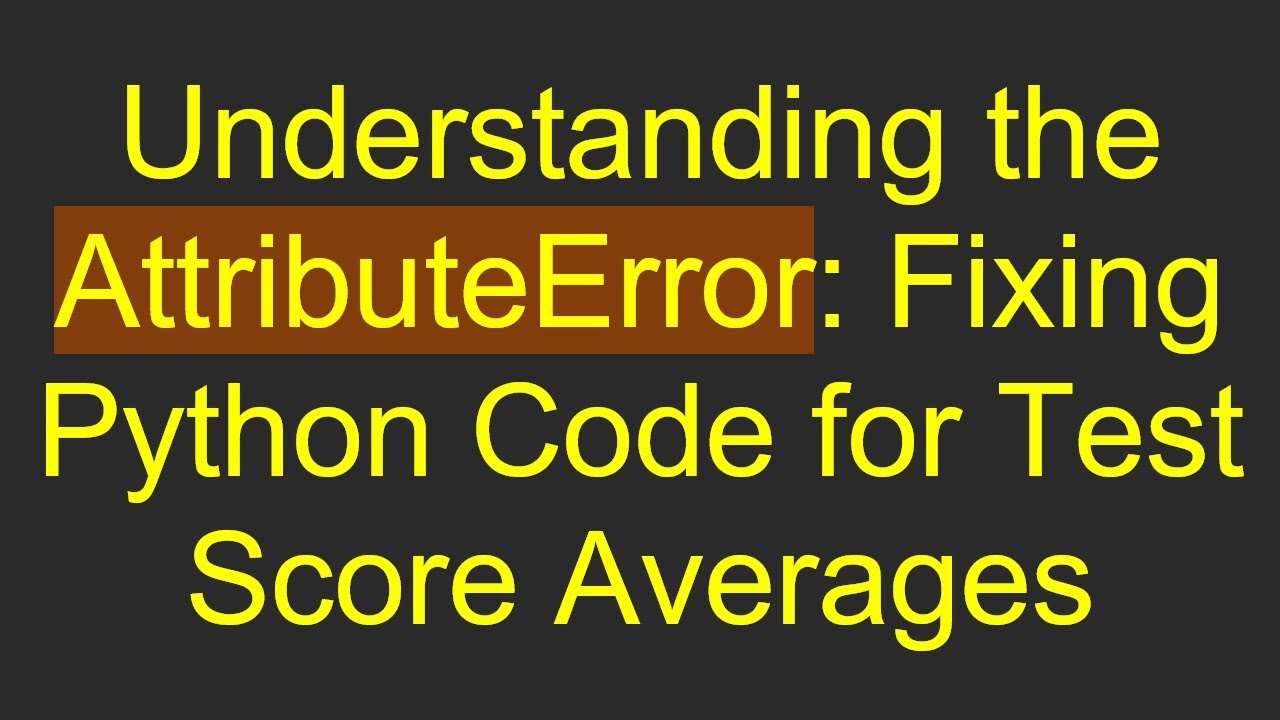
Показать описание
Learn how to resolve the `AttributeError` in Python when calculating average test scores and understand the differences between data types in programming.
---
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the AttributeError: Fixing Python Code for Test Score Averages
When working with Python, errors can sometimes surface, leading to confusion and frustration. One common issue that programmers, especially beginners, might face is the dreaded AttributeError. This post will explore a specific instance of this error that arises when calculating average test scores and how to rectify it effectively. If you've stumbled upon the error message similar to:
[[See Video to Reveal this Text or Code Snippet]]
while attempting to compute your average scores, you've landed in the right place! Let's dive into the problem and uncover a clear solution.
The Problem
You might have encountered code trying to append values to an integer variable. Here's an example code snippet that led to the error:
[[See Video to Reveal this Text or Code Snippet]]
Here’s what went wrong:
You attempted to use the .append() method on n, which is an integer. In Python, integers do not have the append method; it's a characteristic of list objects. This led to the AttributeError.
The Solution
To fix this issue, you can modify your approach. Instead of attempting to append to an integer, you can maintain a running tally of scores directly. Here’s the revised version of your code that resolves the issue:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of Changes
Score Accumulation: Rather than trying to append scores to an integer, the revised function accumulates the total score in a variable.
The score variable is now initialized at zero and updated in each iteration with the input score.
Looping Structure: The loop iterates through 1 to n to prompt for test scores, making the code more comprehensible.
The use of f-strings enhances readability, as it allows dynamic incorporation of the test number directly into the prompt.
Calculating the Average: After collecting all scores, the average is computed straightforwardly by dividing the total score by the number of tests.
Conclusion
By reworking the way scores are collected and stored in your program, we avoid the issues related to data type errors. This example highlights a crucial lesson for Python programmers: understanding how data types work and the methods available to them is vital. Remember, assigning values and using methods suited for those data types is essential in programming.
Now that you know how to fix that pesky AttributeError, you can proceed to calculate your average test scores with confidence. Happy coding!
---
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the AttributeError: Fixing Python Code for Test Score Averages
When working with Python, errors can sometimes surface, leading to confusion and frustration. One common issue that programmers, especially beginners, might face is the dreaded AttributeError. This post will explore a specific instance of this error that arises when calculating average test scores and how to rectify it effectively. If you've stumbled upon the error message similar to:
[[See Video to Reveal this Text or Code Snippet]]
while attempting to compute your average scores, you've landed in the right place! Let's dive into the problem and uncover a clear solution.
The Problem
You might have encountered code trying to append values to an integer variable. Here's an example code snippet that led to the error:
[[See Video to Reveal this Text or Code Snippet]]
Here’s what went wrong:
You attempted to use the .append() method on n, which is an integer. In Python, integers do not have the append method; it's a characteristic of list objects. This led to the AttributeError.
The Solution
To fix this issue, you can modify your approach. Instead of attempting to append to an integer, you can maintain a running tally of scores directly. Here’s the revised version of your code that resolves the issue:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of Changes
Score Accumulation: Rather than trying to append scores to an integer, the revised function accumulates the total score in a variable.
The score variable is now initialized at zero and updated in each iteration with the input score.
Looping Structure: The loop iterates through 1 to n to prompt for test scores, making the code more comprehensible.
The use of f-strings enhances readability, as it allows dynamic incorporation of the test number directly into the prompt.
Calculating the Average: After collecting all scores, the average is computed straightforwardly by dividing the total score by the number of tests.
Conclusion
By reworking the way scores are collected and stored in your program, we avoid the issues related to data type errors. This example highlights a crucial lesson for Python programmers: understanding how data types work and the methods available to them is vital. Remember, assigning values and using methods suited for those data types is essential in programming.
Now that you know how to fix that pesky AttributeError, you can proceed to calculate your average test scores with confidence. Happy coding!