filmov
tv
Intro to MongoDB with C# - Learn what NoSQL is, why it is different than SQL and how to use it in C#
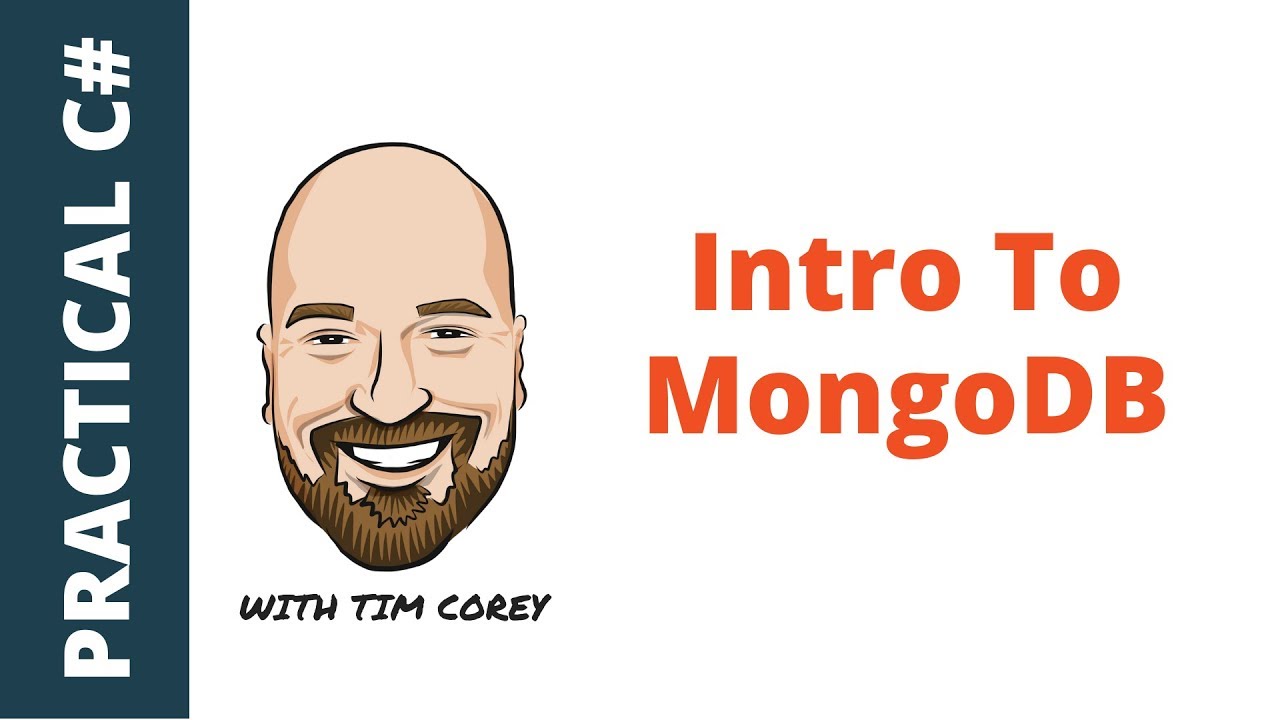
Показать описание
MongoDB is a NoSQL database type that works really well with C#. Learn how to perform CRUD operations, how to modify the schema of a document, and how to get just the data you need back.
This covers MongoDB 4, which is now ACID-compliant and is a direct competitor for major relational databases like Microsoft SQL. See just how easy it is to get started.
Thanks to Ralfs HBK for the channel breakout:
0:00 - Intro
1:27 - Setup: Mongo DB Community Server installation
8:06 - Creating Demo Core Console App
9:35 - MongoDB NuGet
11:04 - Mongo CRUD
12:50 - "Connection string"
14:50 - Connecting to database
17:13 - Data storage in MongoDB
24:39 - Inserting data in database
28:07 - Changing data set structure
30:45 - Inserting different data set in the table and benefit of NoSQL
36:52 - Retrieving data form database
42:00 - MongoDB Query
48:40 - Update and delete methods
54:28 - Insert or Update record
59:00 - Delete record
59:42 - Returning different data model from database record
1:02:55 - Demo code clean up
1:03:20 - MongoDB when and why?
1:15:15 - Summary and concluding remarks
This covers MongoDB 4, which is now ACID-compliant and is a direct competitor for major relational databases like Microsoft SQL. See just how easy it is to get started.
Thanks to Ralfs HBK for the channel breakout:
0:00 - Intro
1:27 - Setup: Mongo DB Community Server installation
8:06 - Creating Demo Core Console App
9:35 - MongoDB NuGet
11:04 - Mongo CRUD
12:50 - "Connection string"
14:50 - Connecting to database
17:13 - Data storage in MongoDB
24:39 - Inserting data in database
28:07 - Changing data set structure
30:45 - Inserting different data set in the table and benefit of NoSQL
36:52 - Retrieving data form database
42:00 - MongoDB Query
48:40 - Update and delete methods
54:28 - Insert or Update record
59:00 - Delete record
59:42 - Returning different data model from database record
1:02:55 - Demo code clean up
1:03:20 - MongoDB when and why?
1:15:15 - Summary and concluding remarks
Комментарии