filmov
tv
LeetCode Daily: Longest Subarray With Maximum Bitwise AND Solution in Java | September 14, 2024
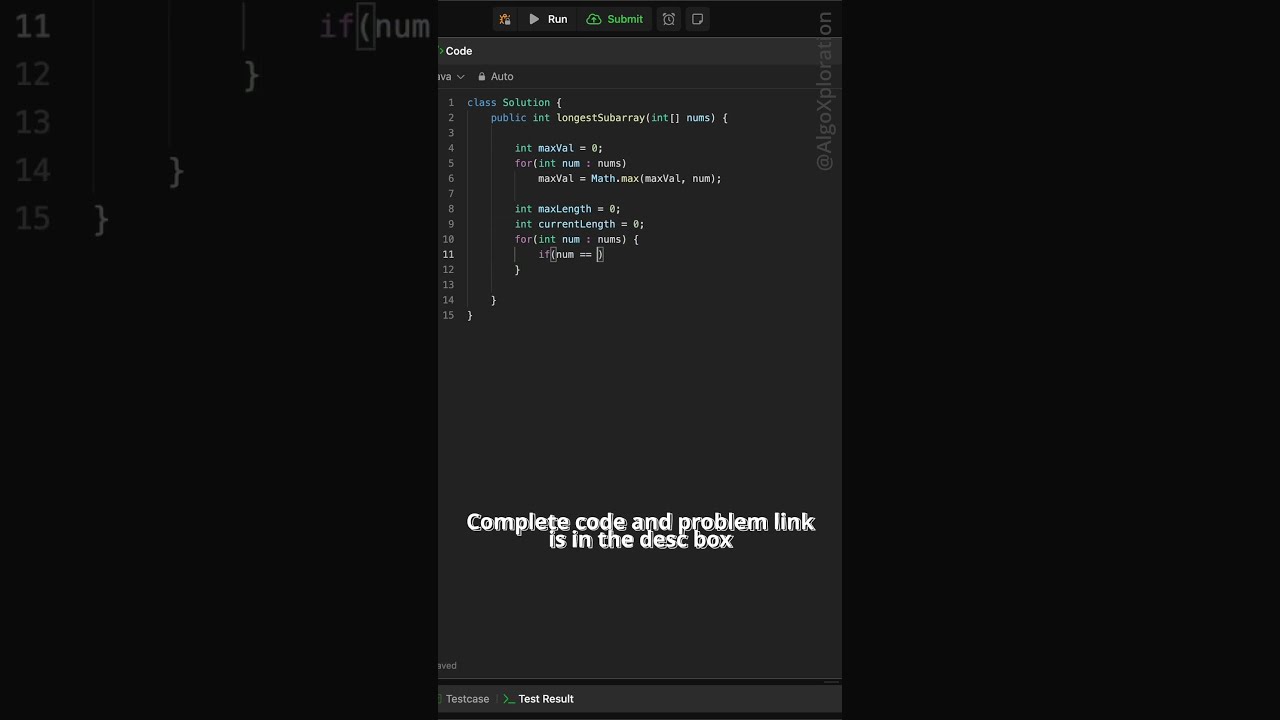
Показать описание
🔍 LeetCode Problem of the Day: 2419. Longest Subarray With Maximum Bitwise AND
In today's LeetCode daily challenge, we solve the Longest Subarray With Maximum Bitwise AND problem by identifying the longest subarray where all elements are equal to the maximum value in the given array. The solution is implemented in Java.
👉 Solution: Pinned in the comments.
🌟 Problem Description:
Given an array of integers, the task is to find the longest subarray where all elements have the maximum bitwise AND value from the entire array.
🔑 Key Points:
Max Value Identification: First, identify the maximum value in the array.
Longest Subarray: Traverse the array to find the longest contiguous subarray where each element equals this maximum value.
Efficiency: The solution runs in linear time, O(n), by scanning the array twice.
📝 Code Explanation:
Step 1: Iterate through the array to find the maximum value.
Step 2: Traverse the array again to calculate the longest subarray where the elements match the maximum value.
Optimization: The length of the longest contiguous subarray is updated whenever we encounter the maximum value.
📅 Daily Solutions:
Subscribe for daily LeetCode solutions explained step-by-step in Java!
👥 Join the Community:
Share your thoughts on the problem in the comments. Discuss different approaches with fellow coders. Like, share, and subscribe for more daily coding challenges!
#LeetCode #Coding #Programming #TechInterview #DailyChallenge #LongestSubarray #BitwiseAND #Java
In today's LeetCode daily challenge, we solve the Longest Subarray With Maximum Bitwise AND problem by identifying the longest subarray where all elements are equal to the maximum value in the given array. The solution is implemented in Java.
👉 Solution: Pinned in the comments.
🌟 Problem Description:
Given an array of integers, the task is to find the longest subarray where all elements have the maximum bitwise AND value from the entire array.
🔑 Key Points:
Max Value Identification: First, identify the maximum value in the array.
Longest Subarray: Traverse the array to find the longest contiguous subarray where each element equals this maximum value.
Efficiency: The solution runs in linear time, O(n), by scanning the array twice.
📝 Code Explanation:
Step 1: Iterate through the array to find the maximum value.
Step 2: Traverse the array again to calculate the longest subarray where the elements match the maximum value.
Optimization: The length of the longest contiguous subarray is updated whenever we encounter the maximum value.
📅 Daily Solutions:
Subscribe for daily LeetCode solutions explained step-by-step in Java!
👥 Join the Community:
Share your thoughts on the problem in the comments. Discuss different approaches with fellow coders. Like, share, and subscribe for more daily coding challenges!
#LeetCode #Coding #Programming #TechInterview #DailyChallenge #LongestSubarray #BitwiseAND #Java
Комментарии