filmov
tv
Converting Primitive Types to Wrapper Classes in Java
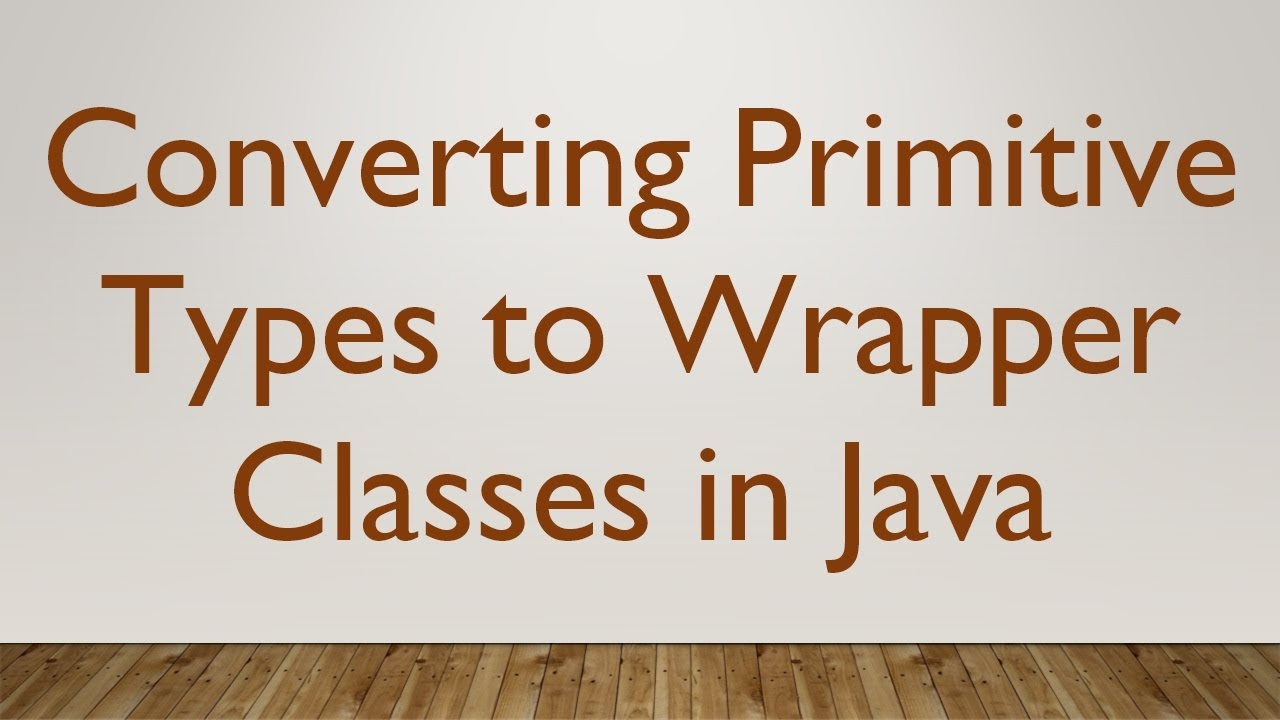
Показать описание
Learn how to convert primitive data types to their corresponding wrapper classes in Java for enhanced functionality and compatibility in your code. Explore the straightforward methods for performing this conversion effortlessly.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
In Java, primitive data types like int, double, boolean, etc., lack the functionalities provided by their corresponding wrapper classes (Integer, Double, Boolean, etc.). Wrapper classes offer additional methods and compatibility with collections and other Java APIs. Therefore, converting primitive types to wrapper classes is a common operation in Java programming. Here's how you can achieve it:
Using Constructors
One straightforward way to convert a primitive type to its wrapper class is by using the constructors provided by the wrapper classes. For example:
[[See Video to Reveal this Text or Code Snippet]]
Similarly, for other primitive types:
[[See Video to Reveal this Text or Code Snippet]]
Using ValueOf() Method
Another convenient approach is to use the valueOf() method provided by the wrapper classes. This method accepts a primitive value as an argument and returns an instance of the corresponding wrapper class. Here's how you can use it:
[[See Video to Reveal this Text or Code Snippet]]
Auto-Boxing (Implicit Conversion)
Java also supports auto-boxing, where primitive types can be automatically converted to their corresponding wrapper classes, and vice versa, without explicit constructor calls or valueOf() method invocations. This feature simplifies the code and enhances readability:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Converting primitive types to wrapper classes in Java is essential for leveraging the additional functionalities provided by wrapper classes and ensuring compatibility with various Java APIs and libraries. Whether you choose to use constructors, the valueOf() method, or rely on auto-boxing, Java offers multiple convenient methods to perform this conversion effortlessly.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
In Java, primitive data types like int, double, boolean, etc., lack the functionalities provided by their corresponding wrapper classes (Integer, Double, Boolean, etc.). Wrapper classes offer additional methods and compatibility with collections and other Java APIs. Therefore, converting primitive types to wrapper classes is a common operation in Java programming. Here's how you can achieve it:
Using Constructors
One straightforward way to convert a primitive type to its wrapper class is by using the constructors provided by the wrapper classes. For example:
[[See Video to Reveal this Text or Code Snippet]]
Similarly, for other primitive types:
[[See Video to Reveal this Text or Code Snippet]]
Using ValueOf() Method
Another convenient approach is to use the valueOf() method provided by the wrapper classes. This method accepts a primitive value as an argument and returns an instance of the corresponding wrapper class. Here's how you can use it:
[[See Video to Reveal this Text or Code Snippet]]
Auto-Boxing (Implicit Conversion)
Java also supports auto-boxing, where primitive types can be automatically converted to their corresponding wrapper classes, and vice versa, without explicit constructor calls or valueOf() method invocations. This feature simplifies the code and enhances readability:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Converting primitive types to wrapper classes in Java is essential for leveraging the additional functionalities provided by wrapper classes and ensuring compatibility with various Java APIs and libraries. Whether you choose to use constructors, the valueOf() method, or rely on auto-boxing, Java offers multiple convenient methods to perform this conversion effortlessly.