filmov
tv
Data Structures and Algorithms In C#: Array + Array Insertions (Part 1)
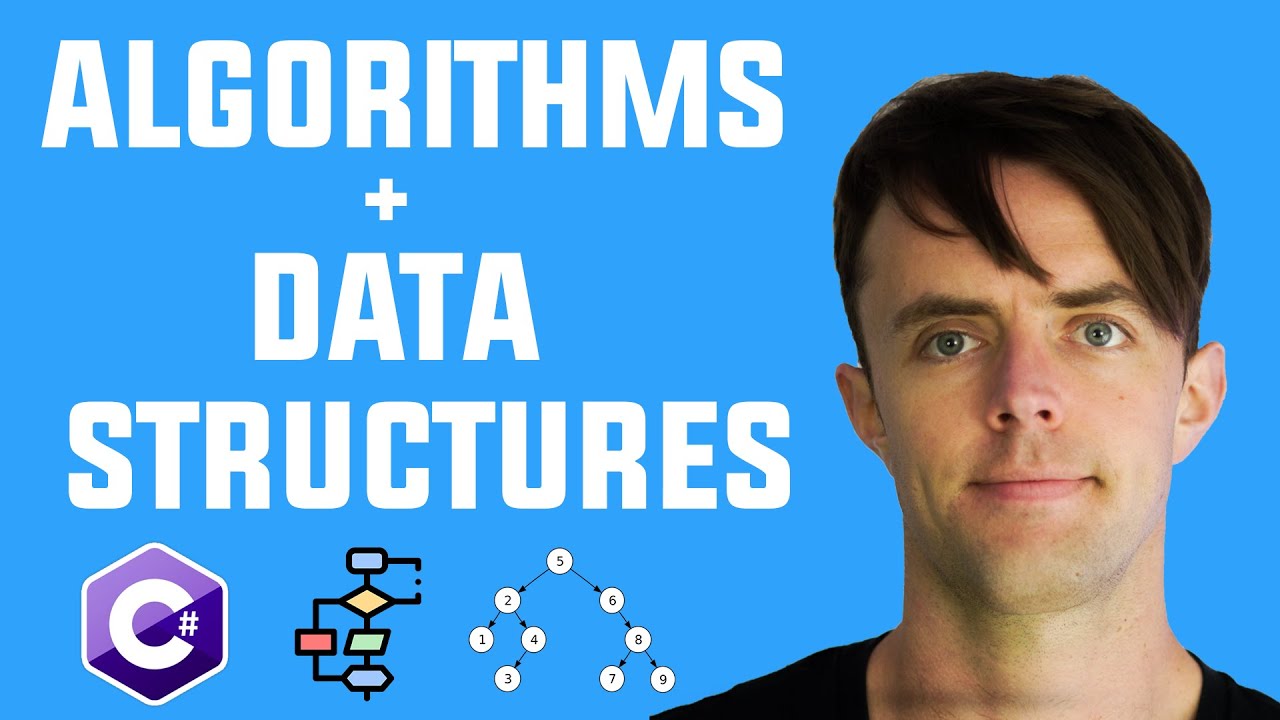
Показать описание
Data Structures and Algorithms In C#: Array + Array Insertions (Part 1)
Fastest way to learn Data Structures and Algorithms
Algorithms and Data Structures Tutorial - Full Course for Beginners
How I mastered Data Structures and Algorithms
Data Structures and Algorithms for Beginners
How I Mastered Data Structures and Algorithms
How I Would Learn Data Structures & Algorithms in 2024 (if I was starting over)
10 Key Data Structures We Use Every Day
Learn Data Structures and Algorithms for free 📈
Data Structures and Algorithms in Python - Full Course for Beginners
Data Structures and Algorithms with Visualizations – Full Course (Java)
What are data structures and algorithms? 📈
The Best Order to learn Algorithms & Data Structures?
How to ACTUALLY Master Data Structures FAST (with real coding examples)
Top 6 Coding Interview Concepts (Data Structures & Algorithms)
How Data Structures & Algorithms are Actually Used
Data Structures & Algorithms Roadmap - What You NEED To Learn
Data Structures and Algorithms in JavaScript - Full Course for Beginners
Top 7 Data Structures for Interviews Explained SIMPLY
Data Structures And Algorithms in Python - Python Data Structures Full Tutorial (2020)
How I'd Learn Data Structures & Algorithms For Free
How I mastered Data Structures and Algorithms #dsa #codinginterview #leetcode
Best Order to Learn Algorithms & Data Structures
4 Data Structures You Need to Know
DSA Complete Series 🚀 : Data Structures & Algorithms | by Shradha Khapra Ma'am
Комментарии