filmov
tv
Data Structures and Algorithms with Visualizations – Full Course (Java)
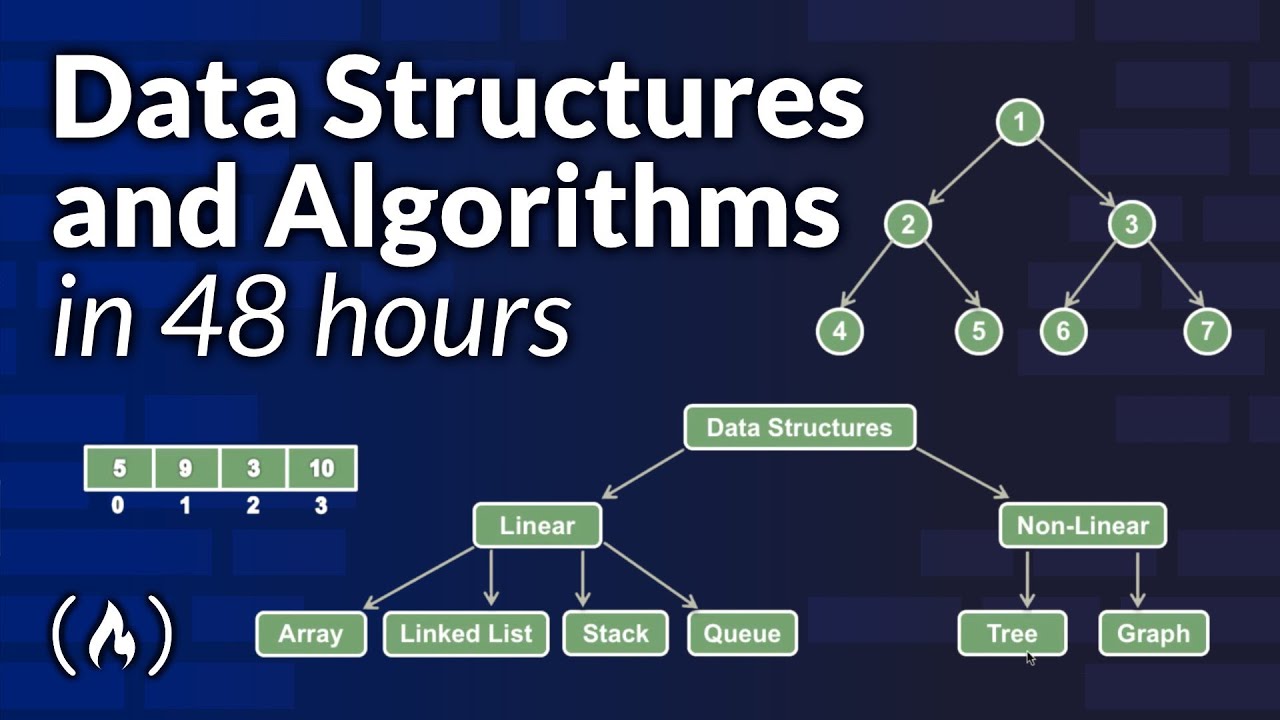
Показать описание
Data Structures and Algorithms is an important aspect of every coding interview. This course will help you prepare for placements, coding interviews, and logic building.
✏️ Course developed by @itsdineshvaryani
The course walks you through multiple Java algorithms, data structure problems, and their solutions with step-by-step visualizations, so that you are actually learning instead of blindly memorizing solutions.
⭐️ Contents ⭐️
00:00:00 Introduction
00:03:46 Introduction to Data Structures
00:03:46 Introduction to Data Structures
00:08:49 Introduction to Algorithms
00:19:43 Time Complexity of an Algorithm
00:25:05 Space Complexity of an Algorithm
00:27:14 Asymptotic Analysis of an Algorithm
00:29:43 Asymptotic Notations
00:39:06 Analysis and Rules to calculate Big O notation
01:06:16 One-Dimensional Array
01:32:38 print elements of an Array
01:44:29 Remove Even Integers from an Array
02:03:54 Reverse an Array
02:16:09 find Minimum value in an Array
02:28:13 Find Second Maximum value in an Array
02:41:03 move Zeroes to end of an Array
03:01:46 resize an Array
03:19:58 Find the Missing Number in an Array
03:34:08 check if a given String is a Palindrome
03:52:52 Create a Singly Linked List
04:02:58 Print elements of a Singly Linked List
04:13:11 Find length of a Singly Linked List
04:22:52 Insert nodes in a Singly Linked List
05:16:40 Delete nodes of a Singly Linked List
06:12:06 search an element in a Singly Linked List
06:22:42 Reverse a Singly Linked List
06:33:27 find nth node from the end of a Singly Linked List
06:46:43 remove duplicate from sorted Singy Linked List
06:57:51 insert a node in a sorted Singly Linked List
07:09:37 remove a given key from Singly Linked List
07:20:10 detect a loop in a Singly Linked List
07:36:58 find start of a loop in a Singly Linked List?
07:57:54 Why Floyd's Cycle Detection algorithm works?
08:17:23 remove loop from a Singly Linked List?
08:37:36 Merge Two Sorted ListsQuestion
09:21:54 LeetCode #2: Add Two Numbers
09:41:53 represent a Doubly Linked List
09:46:06 implement Doubly Linked List
09:52:27 print elements of a Doubly Linked List
10:05:10 insert node at the beginning of a Doubly Linked List
10:15:25 Insert node at the end of a Doubly Linked List
10:25:50 delete first node in a Doubly Linked List
10:41:08 delete last node in a Doubly Linked List
10:55:49 represent a Circular Singly Linked List
11:03:46 implement a Circular Singly Linked List
11:11:20 traverse and print a Circular Singly Linked List
11:22:55 insert node at the start of a Circular Singly Linked List
11:37:48 insert node at the end of a Circular Singly Linked List
11:51:52 remove first node from a Circular Singly Linked List
12:10:37 Stacks
13:08:22 Next Greater Element
13:29:44 Valid Parentheses problem (Balanced Brackets)
13:46:11 represent a Queue
13:52:52 implement a Queue
14:31:59 Generate Binary numbers from 1 to n using a Queue
14:50:36 Binary Trees
20:27:59 Search in a row and column wise sorted matrix
20:49:30 Print a given matrix in Spiral form
21:23:55 Introduction to Priority Queue and Binary Heap
21:32:00 represent a Binary Heap
21:43:41 implement Max Heap
21:56:25 Bottom - Up Reheapify (Swim) in Max Heap
22:09:30 insert in a Max Heap
22:45:34 Top - Down Reheapify (Sink) in Max Heap
23:02:17 delete max element in a Max Heap
23:25:57 Linear Search
23:35:47 Binary Search
23:51:38 Search Insert Position in a Sorted Array
24:13:22 Bubble Sort
24:51:29 Insertion Sort
25:33:57 Selection Sort Algorithm
26:16:06 merge two sorted arrays
26:53:01 Merge Sort
28:33:26 Sort an array of 0’s, 1’s, and 2’s (Dutch National Flag Problem)
29:06:31 Quick Sort Algorithm
30:42:59 Squares of a Sorted Array
31:07:03 Rearrange Sorted Array in Max⧸Min form
31:38:01 Graphs
33:55:55 Number of Islands
34:43:56 Hashing and Hash Tables
37:33:21 Contains Duplicate
37:45:19 Introduction to Intervals and Overlapping Intervals
37:53:40 Merge Intervals
38:15:53 Insert Interval
38:40:02 Trie Data Structures
39:47:59 Dynamic Programming
41:26:02 Kadane's Algorithm for Maximum Sum Subarray
42:12:39 LeetCode: Two Sum
42:54:26 Is Valid Subsequence problem
43:22:23 First Non-Repeating Character in a String
43:48:45 Remove Vowels from a String
44:04:10 Reverse an Integer
44:23:13 Remove Element
44:40:54 Remove Duplicates from Sorted Array
44:58:57 Three Sum problem Animation
45:22:37 Product of an Array except self
45:45:01 Sliding Window Maximum
46:32:09 Maximum Sum Subarray of Size K
46:59:45 LeetCode: Longest Substring Without Repeating Characters
47:24:15 LeetCode: Symmetric Tree
✏️ Course developed by @itsdineshvaryani
The course walks you through multiple Java algorithms, data structure problems, and their solutions with step-by-step visualizations, so that you are actually learning instead of blindly memorizing solutions.
⭐️ Contents ⭐️
00:00:00 Introduction
00:03:46 Introduction to Data Structures
00:03:46 Introduction to Data Structures
00:08:49 Introduction to Algorithms
00:19:43 Time Complexity of an Algorithm
00:25:05 Space Complexity of an Algorithm
00:27:14 Asymptotic Analysis of an Algorithm
00:29:43 Asymptotic Notations
00:39:06 Analysis and Rules to calculate Big O notation
01:06:16 One-Dimensional Array
01:32:38 print elements of an Array
01:44:29 Remove Even Integers from an Array
02:03:54 Reverse an Array
02:16:09 find Minimum value in an Array
02:28:13 Find Second Maximum value in an Array
02:41:03 move Zeroes to end of an Array
03:01:46 resize an Array
03:19:58 Find the Missing Number in an Array
03:34:08 check if a given String is a Palindrome
03:52:52 Create a Singly Linked List
04:02:58 Print elements of a Singly Linked List
04:13:11 Find length of a Singly Linked List
04:22:52 Insert nodes in a Singly Linked List
05:16:40 Delete nodes of a Singly Linked List
06:12:06 search an element in a Singly Linked List
06:22:42 Reverse a Singly Linked List
06:33:27 find nth node from the end of a Singly Linked List
06:46:43 remove duplicate from sorted Singy Linked List
06:57:51 insert a node in a sorted Singly Linked List
07:09:37 remove a given key from Singly Linked List
07:20:10 detect a loop in a Singly Linked List
07:36:58 find start of a loop in a Singly Linked List?
07:57:54 Why Floyd's Cycle Detection algorithm works?
08:17:23 remove loop from a Singly Linked List?
08:37:36 Merge Two Sorted ListsQuestion
09:21:54 LeetCode #2: Add Two Numbers
09:41:53 represent a Doubly Linked List
09:46:06 implement Doubly Linked List
09:52:27 print elements of a Doubly Linked List
10:05:10 insert node at the beginning of a Doubly Linked List
10:15:25 Insert node at the end of a Doubly Linked List
10:25:50 delete first node in a Doubly Linked List
10:41:08 delete last node in a Doubly Linked List
10:55:49 represent a Circular Singly Linked List
11:03:46 implement a Circular Singly Linked List
11:11:20 traverse and print a Circular Singly Linked List
11:22:55 insert node at the start of a Circular Singly Linked List
11:37:48 insert node at the end of a Circular Singly Linked List
11:51:52 remove first node from a Circular Singly Linked List
12:10:37 Stacks
13:08:22 Next Greater Element
13:29:44 Valid Parentheses problem (Balanced Brackets)
13:46:11 represent a Queue
13:52:52 implement a Queue
14:31:59 Generate Binary numbers from 1 to n using a Queue
14:50:36 Binary Trees
20:27:59 Search in a row and column wise sorted matrix
20:49:30 Print a given matrix in Spiral form
21:23:55 Introduction to Priority Queue and Binary Heap
21:32:00 represent a Binary Heap
21:43:41 implement Max Heap
21:56:25 Bottom - Up Reheapify (Swim) in Max Heap
22:09:30 insert in a Max Heap
22:45:34 Top - Down Reheapify (Sink) in Max Heap
23:02:17 delete max element in a Max Heap
23:25:57 Linear Search
23:35:47 Binary Search
23:51:38 Search Insert Position in a Sorted Array
24:13:22 Bubble Sort
24:51:29 Insertion Sort
25:33:57 Selection Sort Algorithm
26:16:06 merge two sorted arrays
26:53:01 Merge Sort
28:33:26 Sort an array of 0’s, 1’s, and 2’s (Dutch National Flag Problem)
29:06:31 Quick Sort Algorithm
30:42:59 Squares of a Sorted Array
31:07:03 Rearrange Sorted Array in Max⧸Min form
31:38:01 Graphs
33:55:55 Number of Islands
34:43:56 Hashing and Hash Tables
37:33:21 Contains Duplicate
37:45:19 Introduction to Intervals and Overlapping Intervals
37:53:40 Merge Intervals
38:15:53 Insert Interval
38:40:02 Trie Data Structures
39:47:59 Dynamic Programming
41:26:02 Kadane's Algorithm for Maximum Sum Subarray
42:12:39 LeetCode: Two Sum
42:54:26 Is Valid Subsequence problem
43:22:23 First Non-Repeating Character in a String
43:48:45 Remove Vowels from a String
44:04:10 Reverse an Integer
44:23:13 Remove Element
44:40:54 Remove Duplicates from Sorted Array
44:58:57 Three Sum problem Animation
45:22:37 Product of an Array except self
45:45:01 Sliding Window Maximum
46:32:09 Maximum Sum Subarray of Size K
46:59:45 LeetCode: Longest Substring Without Repeating Characters
47:24:15 LeetCode: Symmetric Tree
Комментарии