filmov
tv
Kth Smallest Element in a BST - Leetcode 230 - Python
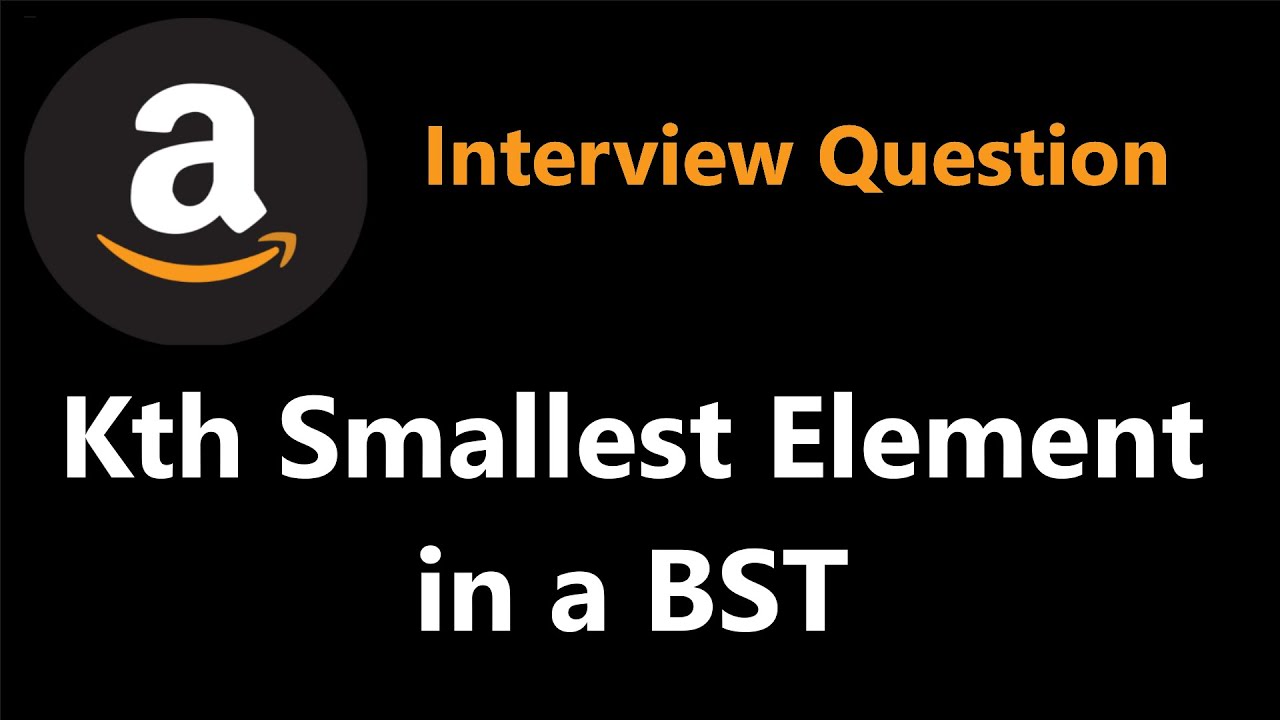
Показать описание
0:00 - Drawing Explanation
7:00 - Coding Solution
leetcode 230
#kth #bst #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Kth Smallest Element in a BST - Leetcode 230 - Python
Kth largest element in an array | Kth smallest element in an array
L45. K-th Smallest/Largest Element in BST
Leetcode - Kth Smallest Element in a BST (Python)
Kth Smallest element in a matrix | Leetcode #378
2 Kth Smallest Element
Kth Smallest Element in a BST | Leetcode #230
Kth Smallest Element in a BST - Leetcode 230 - Trees (Python)
Kth Smallest Element in a BST
Find Kth Smallest element in an array
BST - 17: Get Kth Smallest element in given Binary Search Tree (BST)
Kth Smallest Element in a Sorted Matrix
Leetcode - Kth Smallest Element in a Sorted Matrix (Python)
Find Kth Largest/Smallest Element in an Array | PriorityQueue in Java & C++ | DSA-One Course #33
Kth Smallest Element In An Array | Kth Smallest Element In An Array GeeksForGeeks | Using Heap ||
Kth Smallest Element in a BST - Inorder Traversal
Kth largest element in an array | Kth smallest element in an array
kth largest element in an array | kth smallest element in an array
How to find the kth largest element in an array? (LeetCode .215) - Inside code
Leetcode 378. Kth Smallest Element in a Sorted Matrix [Java]
kth smallest element in an array Solution - Java
Find Kth Largest and Kth Smallest element in a BST | Binary Search Tree | DSA Sheet | Amazon 🔥
Kth Largest Element in an Array - Leetcode 215 - Heaps (Python)
Kth smallest element in a row wise and column wise sorted 2D array
Комментарии