filmov
tv
Copy List with Random Pointer - Linked List - Leetcode 138
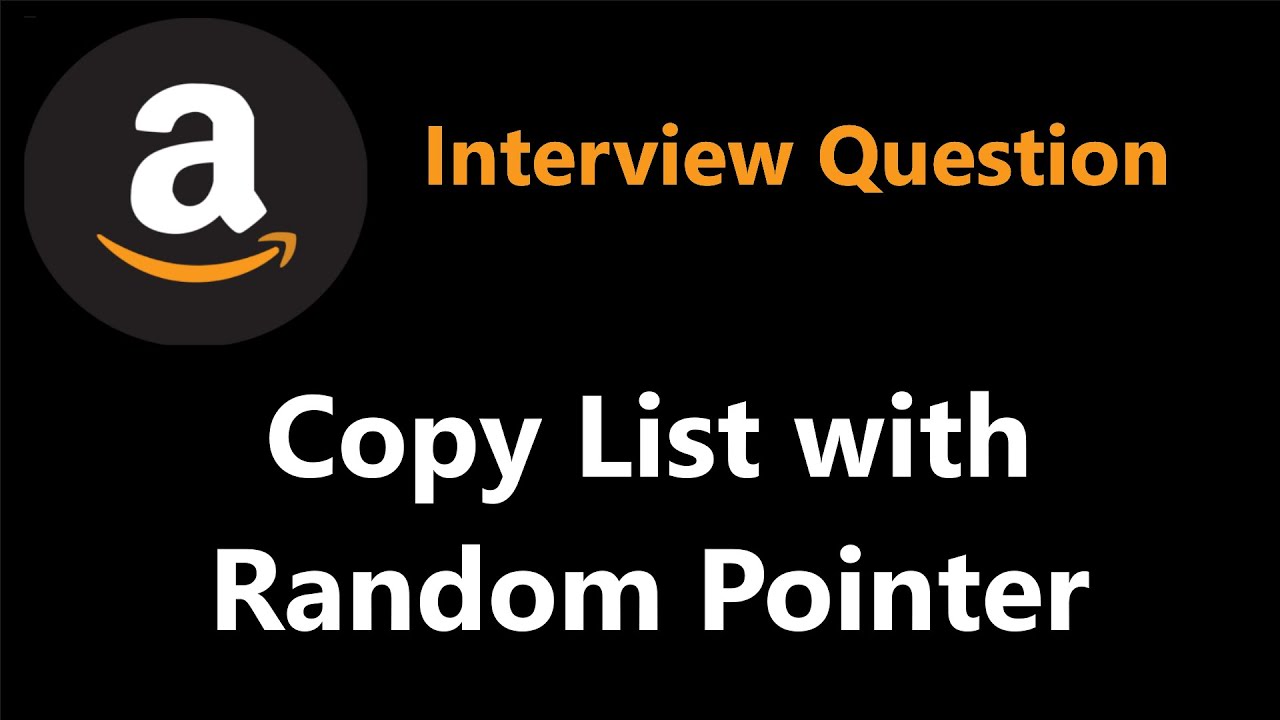
Показать описание
0:00 - Read the problem
5:07 - Coding Explanation
leetcode 138
#sorted #array #python
Copy List with Random Pointer - Linked List - Leetcode 138
L27. Clone a LinkedList with Next and Random Pointers | Copy List with Random Pointers
Copy List With Random Pointer (LeetCode 138) | Full Solution with animations and 3 step process
Clone A Linked List (With Random Pointers) - Linear Space Solution & Tricky Constant Space Solut...
Copy List with Random Pointer - Leetcode 138 - Linked Lists (Python)
COPY LIST WITH RANDOM POINTER | PYTHON | LEETCODE # 138
Leetcode - Copy List with Random Pointer (Python)
Lecture 52: Clone a Linked List with Random Pointers || C++ Placement Course
Copy List with Random Pointer | 2 Approaches | Clear Intuition | Dry Run | Microsoft| Leetcode - 138
Copy List with Random Pointer | Live Coding with Explanation | Leetcode #138
LeetCode 138. Copy List with Random Pointer [IN 3 MINUTES]
The Ultimate Linked List Question! | Copy List With Random Pointer - Leetcode 138
Clone a Linked List with Next and Random Pointer | Important Interview Question | DSA-One Course #40
Copy List with Random Pointer ( NO extra space ) | EP 24
Copy List with Random Pointer ( with extra space ) | EP 23
Clone/Copy a linked list with next and random pointers
Leetcode Solution - 138 Copy List with Random Pointer
LeetCode Tutorial 138. Copy List with Random Pointer
Copy List with Random Pointer | Leetcode 138 | Linked List | Day-12
Разбор задачи 138 leetcode.com Copy List with Random Pointer. Решение на C++
15 min Java Coding Challenge - Copy List With Random Pointer
LeetCode Tutorial 138. Copy List with Random Pointer - Python Explanation
[Java] Leetcode 138. Copy List with Random Pointer (using HashMap) [LinkedList #6]
LeetCode 138. Copy List with Random Pointer
Комментарии