filmov
tv
Resolving JSON Saving Issues in Your Python Code: Tips and Solutions
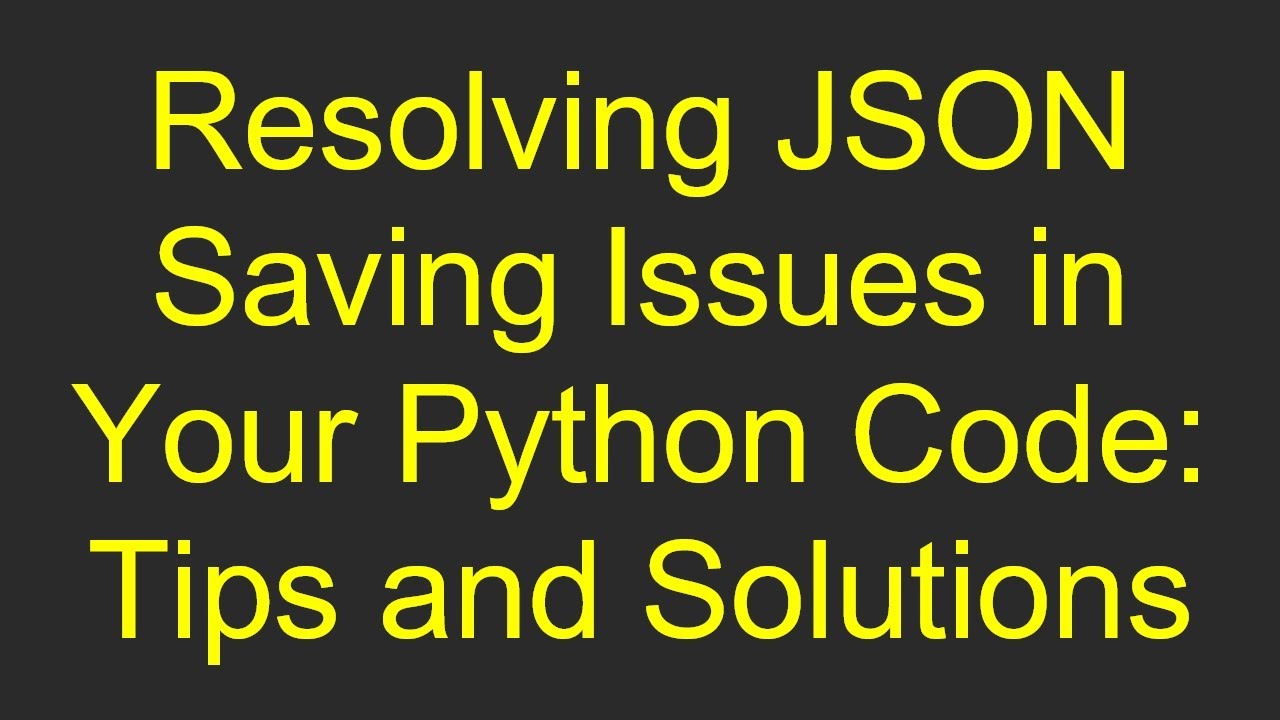
Показать описание
Learn how to fix the JSON loading and saving issues in your Python script, especially when handling mutable and immutable data types.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: json loading correctly but not saving completely
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing JSON Loading and Saving Issues in Python
Handling data correctly is a crucial aspect of programming, especially when it comes to saving and loading information in a structured format like JSON. If you are experiencing issues where your JSON data loads correctly but doesn't save completely, you're not alone. In this guide, we'll delve into a common problem encountered while working with JSON in Python and offer some solutions to help you get back on track.
The Problem
Consider this scenario: You have a Python script that should maintain an inventory of items and a user's money, but when you try to save the updated data, it seems like the money doesn't save properly. You encounter a TypeError: 'int' object is not iterable, which usually indicates that you're trying to loop over something that isn't iterable, like an integer. This can be frustrating, especially after having spent time debugging.
Initial Observations
The error arises when you manipulate the data structures (like dictionaries) incorrectly.
Changes are reflected during runtime, but the updates aren't being saved as expected.
Understanding the Cause
Before we explore a solution, it's important to understand the underlying issue in your script. In your code snippet:
[[See Video to Reveal this Text or Code Snippet]]
The Solution
To fix these issues, you can take one of the following approaches:
Approach 1: Update money in the Data Dictionary Directly
Modify Money Directly:
When you update the money after a purchase:
[[See Video to Reveal this Text or Code Snippet]]
Save Data Properly:
[[See Video to Reveal this Text or Code Snippet]]
Approach 2: Save with Proper JSON File Naming
It's also a best practice to use .json as the file extension for JSON files for clarity and to adhere to file type standards:
Additional Suggestions
Error Handling: Make sure your try-except block doesn’t just create an empty file when one isn’t found. You can start with default values.
Item Purchase Validation: Ensure the user can’t purchase excessive quantities of items that exceed their money by adding proper input validation checks.
Conclusion
By understanding the nature of mutable and immutable data types, and by updating your data structure directly, you can solve JSON saving issues in Python. Implementing these adjustments in your code will ensure that both your inventory and money are saved correctly and persistently. Remember, choosing the right file extension and being careful with error handling will prevent future headaches.
Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: json loading correctly but not saving completely
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing JSON Loading and Saving Issues in Python
Handling data correctly is a crucial aspect of programming, especially when it comes to saving and loading information in a structured format like JSON. If you are experiencing issues where your JSON data loads correctly but doesn't save completely, you're not alone. In this guide, we'll delve into a common problem encountered while working with JSON in Python and offer some solutions to help you get back on track.
The Problem
Consider this scenario: You have a Python script that should maintain an inventory of items and a user's money, but when you try to save the updated data, it seems like the money doesn't save properly. You encounter a TypeError: 'int' object is not iterable, which usually indicates that you're trying to loop over something that isn't iterable, like an integer. This can be frustrating, especially after having spent time debugging.
Initial Observations
The error arises when you manipulate the data structures (like dictionaries) incorrectly.
Changes are reflected during runtime, but the updates aren't being saved as expected.
Understanding the Cause
Before we explore a solution, it's important to understand the underlying issue in your script. In your code snippet:
[[See Video to Reveal this Text or Code Snippet]]
The Solution
To fix these issues, you can take one of the following approaches:
Approach 1: Update money in the Data Dictionary Directly
Modify Money Directly:
When you update the money after a purchase:
[[See Video to Reveal this Text or Code Snippet]]
Save Data Properly:
[[See Video to Reveal this Text or Code Snippet]]
Approach 2: Save with Proper JSON File Naming
It's also a best practice to use .json as the file extension for JSON files for clarity and to adhere to file type standards:
Additional Suggestions
Error Handling: Make sure your try-except block doesn’t just create an empty file when one isn’t found. You can start with default values.
Item Purchase Validation: Ensure the user can’t purchase excessive quantities of items that exceed their money by adding proper input validation checks.
Conclusion
By understanding the nature of mutable and immutable data types, and by updating your data structure directly, you can solve JSON saving issues in Python. Implementing these adjustments in your code will ensure that both your inventory and money are saved correctly and persistently. Remember, choosing the right file extension and being careful with error handling will prevent future headaches.
Happy coding!