filmov
tv
5 Python BAD Practices To Avoid
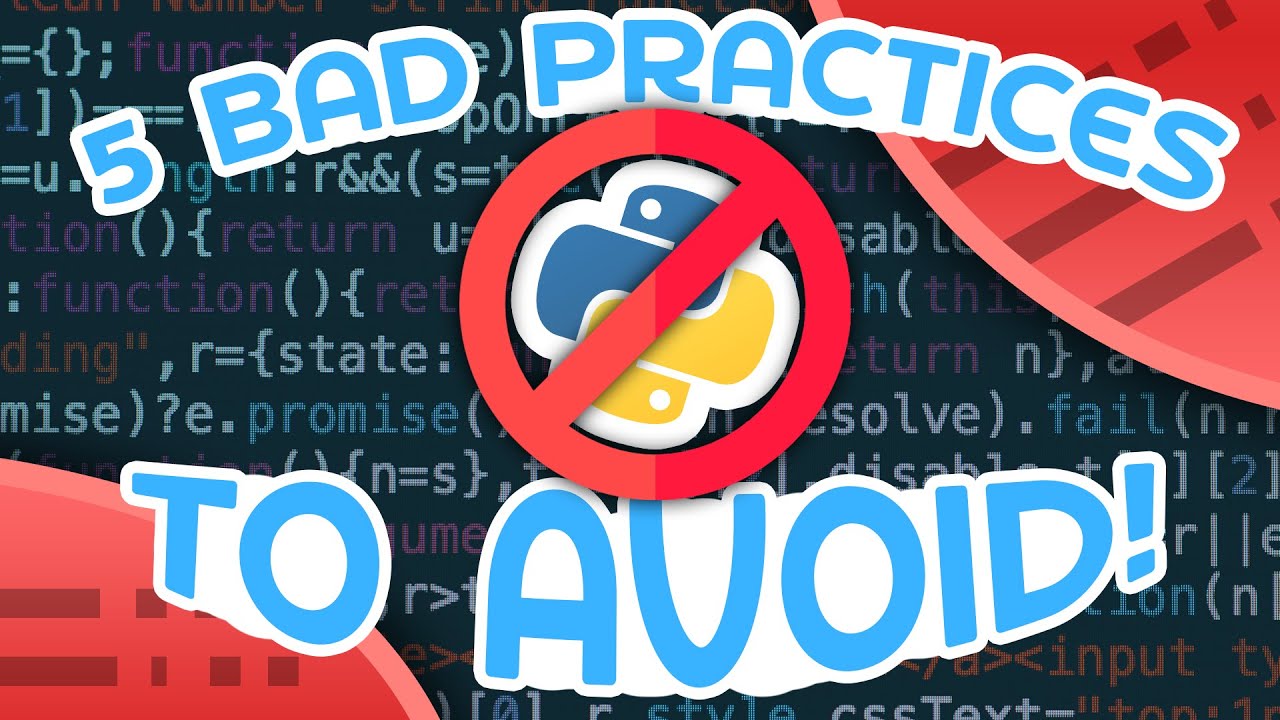
Показать описание
Welcome back to another video! In this video, I'll be covering five bad python practices you should be avoiding. Most of these are things that seem normal or natural for people to do, however, they can lead to problems later and can make code look messy and unreadable.
⭐️ Timestamps ⭐️
00:00 | Overview
01:47 | Mutable Default Parameters
06:24 | Not Using A Default Dict
11:27 | Not Using A Context Manager
14:54 | Not Using Enumerate
17:19 | Overriding Built-In Keywords
◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️
💰 Courses & Merch 💰
🔗 Social Medias 🔗
🎬 My YouTube Gear 🎬
💸 Donations 💸
◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️
⭐️ Tags ⭐️
- Tech With Tim
- Python
- Bad Practices
- Messy Code
- Python Programming
- Vidyard
⭐️ Hashtags ⭐️
#TechWithTim #Python
⭐️ Timestamps ⭐️
00:00 | Overview
01:47 | Mutable Default Parameters
06:24 | Not Using A Default Dict
11:27 | Not Using A Context Manager
14:54 | Not Using Enumerate
17:19 | Overriding Built-In Keywords
◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️
💰 Courses & Merch 💰
🔗 Social Medias 🔗
🎬 My YouTube Gear 🎬
💸 Donations 💸
◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️◼️
⭐️ Tags ⭐️
- Tech With Tim
- Python
- Bad Practices
- Messy Code
- Python Programming
- Vidyard
⭐️ Hashtags ⭐️
#TechWithTim #Python
5 Python BAD Practices To Avoid
5 Good Python Habits
25 nooby Python habits you need to ditch
Python's 5 Worst Features
New Python Coders Be Like...
Python worst Practices
The Truth About Learning Python in 2024
Please NEVER Do THIS In Python :(
ML with Python : Zero to Hero | Video 12 | Sentiment Analysis | Venkat Reddy AI Classes
Python vs C++ Speed Comparison
5 Uncommon Python Features I Love
5 Common Python Mistakes and How to Fix Them
5 Effective Tips to Learn Python Fast (Pro Hacks)🔥
Create a Spiderman using python coding |python programer| #tech #python #coding
7 Python Code Smells: Olfactory Offenses To Avoid At All Costs
Developer Last Expression 😂 #shorts #developer #ytshorts #uiux #python #flutterdevelopment
AVOID Making 'THIS Stupid Mistake' In Python (It's bad)
#5 Python Tutorial for Beginners | List in Python
Python Decorators in 1 Minute!
Automating My Life with Python: The Ultimate Guide | Code With Me
5 Tips For Object-Oriented Programming Done Well - In Python
Everyone Gets THIS Wrong In Python At Least Once
Can you solve this 150 years old puzzle? #shorts
11 Tips And Tricks To Write Better Python Code
Комментарии