filmov
tv
Python calculator app 🖩
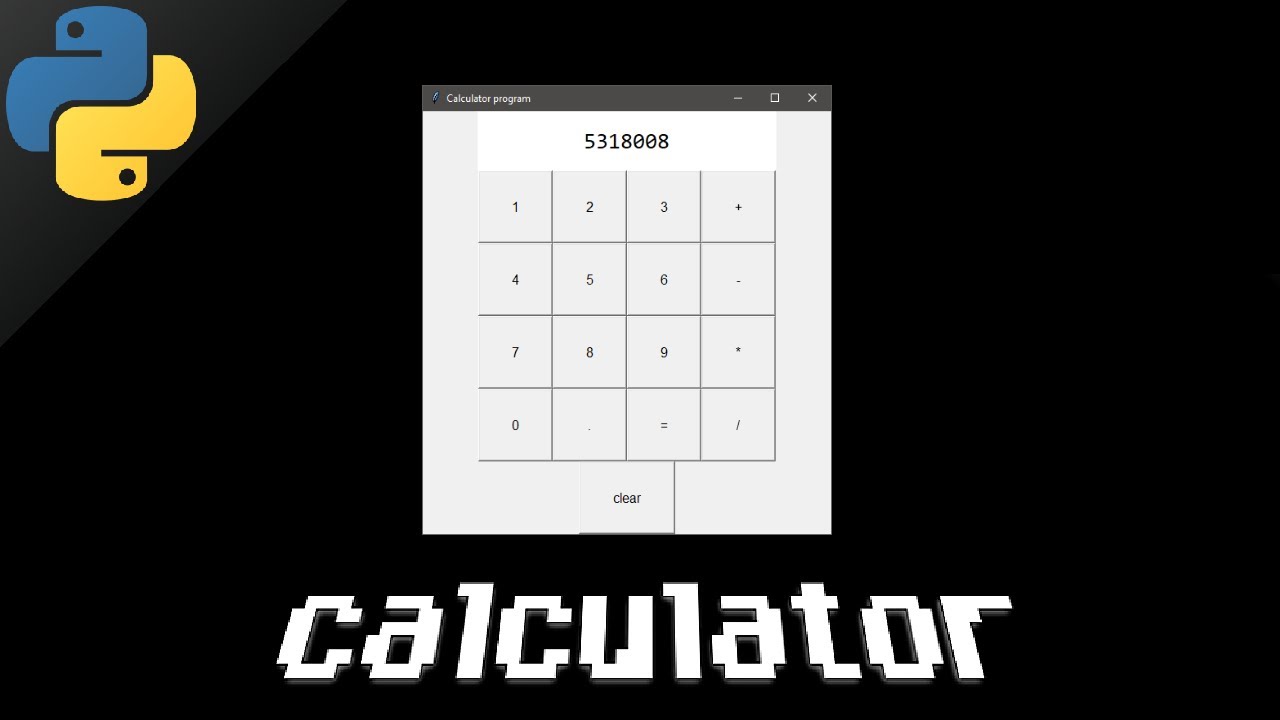
Показать описание
python calculator program project tutorial example explained
#python #calculator #program
# ****************************************************************
# Python Calculator
# ****************************************************************
from tkinter import *
def button_press(num):
global equation_text
equation_text = equation_text + str(num)
def equals():
global equation_text
try:
total = str(eval(equation_text))
equation_text = total
except SyntaxError:
equation_text = ""
except ZeroDivisionError:
equation_text = ""
def clear():
global equation_text
equation_text = ""
window = Tk()
equation_text = ""
equation_label = StringVar()
label = Label(window, textvariable=equation_label, font=('consolas',20), bg="white", width=24, height=2)
frame = Frame(window)
button1 = Button(frame, text=1, height=4, width=9, font=35,
command=lambda: button_press(1))
button2 = Button(frame, text=2, height=4, width=9, font=35,
command=lambda: button_press(2))
button3 = Button(frame, text=3, height=4, width=9, font=35,
command=lambda: button_press(3))
button4 = Button(frame, text=4, height=4, width=9, font=35,
command=lambda: button_press(4))
button5 = Button(frame, text=5, height=4, width=9, font=35,
command=lambda: button_press(5))
button6 = Button(frame, text=6, height=4, width=9, font=35,
command=lambda: button_press(6))
button7 = Button(frame, text=7, height=4, width=9, font=35,
command=lambda: button_press(7))
button8 = Button(frame, text=8, height=4, width=9, font=35,
command=lambda: button_press(8))
button9 = Button(frame, text=9, height=4, width=9, font=35,
command=lambda: button_press(9))
button0 = Button(frame, text=0, height=4, width=9, font=35,
command=lambda: button_press(0))
plus = Button(frame, text='+', height=4, width=9, font=35,
command=lambda: button_press('+'))
minus = Button(frame, text='-', height=4, width=9, font=35,
command=lambda: button_press('-'))
multiply = Button(frame, text='*', height=4, width=9, font=35,
command=lambda: button_press('*'))
divide = Button(frame, text='/', height=4, width=9, font=35,
command=lambda: button_press('/'))
equal = Button(frame, text='=', height=4, width=9, font=35,
command=equals)
decimal = Button(frame, text='.', height=4, width=9, font=35,
command=lambda: button_press('.'))
clear = Button(window, text='clear', height=4, width=12, font=35,
command=clear)
# ****************************************************************
Bro Code merch store 👟 :
===========================================================
===========================================================
music credits 🎼 :
===========================================================
Creative Commons — Attribution-ShareAlike 3.0 Unported— CC BY-SA 3.0
===========================================================
#python #calculator #program
# ****************************************************************
# Python Calculator
# ****************************************************************
from tkinter import *
def button_press(num):
global equation_text
equation_text = equation_text + str(num)
def equals():
global equation_text
try:
total = str(eval(equation_text))
equation_text = total
except SyntaxError:
equation_text = ""
except ZeroDivisionError:
equation_text = ""
def clear():
global equation_text
equation_text = ""
window = Tk()
equation_text = ""
equation_label = StringVar()
label = Label(window, textvariable=equation_label, font=('consolas',20), bg="white", width=24, height=2)
frame = Frame(window)
button1 = Button(frame, text=1, height=4, width=9, font=35,
command=lambda: button_press(1))
button2 = Button(frame, text=2, height=4, width=9, font=35,
command=lambda: button_press(2))
button3 = Button(frame, text=3, height=4, width=9, font=35,
command=lambda: button_press(3))
button4 = Button(frame, text=4, height=4, width=9, font=35,
command=lambda: button_press(4))
button5 = Button(frame, text=5, height=4, width=9, font=35,
command=lambda: button_press(5))
button6 = Button(frame, text=6, height=4, width=9, font=35,
command=lambda: button_press(6))
button7 = Button(frame, text=7, height=4, width=9, font=35,
command=lambda: button_press(7))
button8 = Button(frame, text=8, height=4, width=9, font=35,
command=lambda: button_press(8))
button9 = Button(frame, text=9, height=4, width=9, font=35,
command=lambda: button_press(9))
button0 = Button(frame, text=0, height=4, width=9, font=35,
command=lambda: button_press(0))
plus = Button(frame, text='+', height=4, width=9, font=35,
command=lambda: button_press('+'))
minus = Button(frame, text='-', height=4, width=9, font=35,
command=lambda: button_press('-'))
multiply = Button(frame, text='*', height=4, width=9, font=35,
command=lambda: button_press('*'))
divide = Button(frame, text='/', height=4, width=9, font=35,
command=lambda: button_press('/'))
equal = Button(frame, text='=', height=4, width=9, font=35,
command=equals)
decimal = Button(frame, text='.', height=4, width=9, font=35,
command=lambda: button_press('.'))
clear = Button(window, text='clear', height=4, width=12, font=35,
command=clear)
# ****************************************************************
Bro Code merch store 👟 :
===========================================================
===========================================================
music credits 🎼 :
===========================================================
Creative Commons — Attribution-ShareAlike 3.0 Unported— CC BY-SA 3.0
===========================================================
Комментарии