filmov
tv
LeetCode Daily: Find the Length of the Longest Common Prefix Solution in Java | September 24, 2024
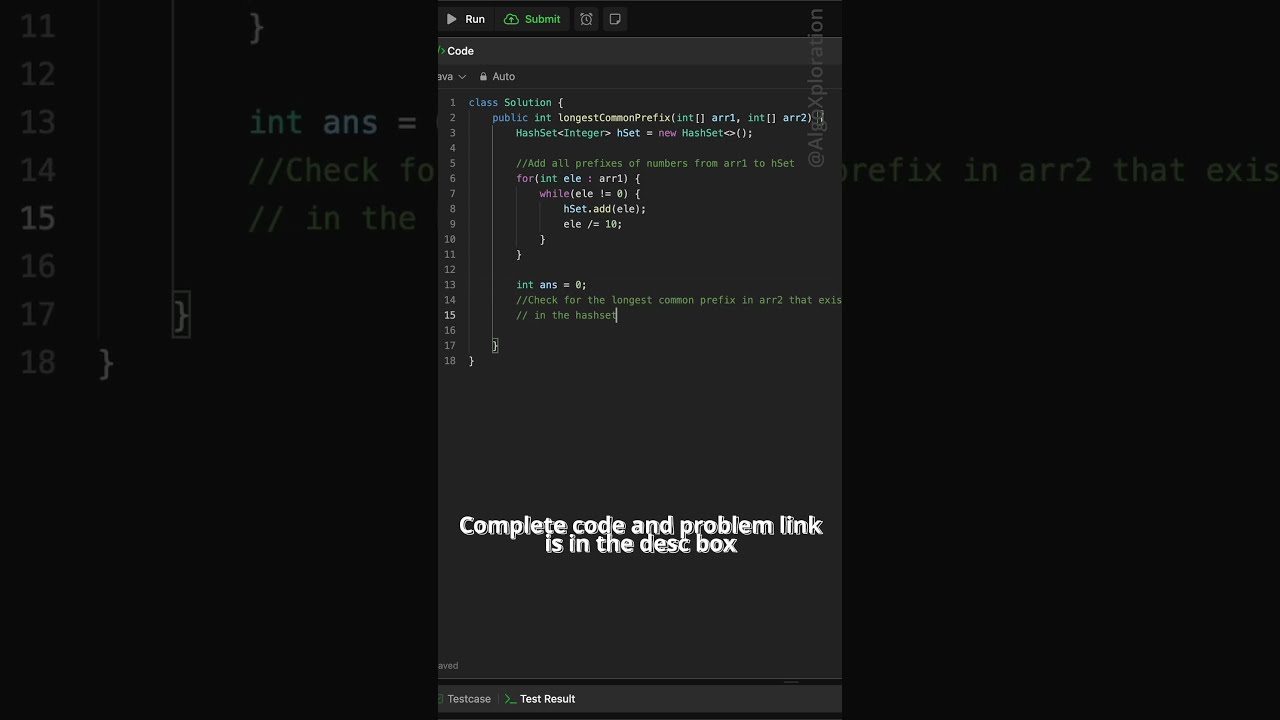
Показать описание
🔍 LeetCode Problem of the Day: Find the Length of the Longest Common Prefix
In today's LeetCode daily challenge, we solve the Find the Length of the Longest Common Prefix problem by identifying the longest common prefix between two arrays of integers. The solution is implemented in Java.
👉 Solution: Pinned in the comments.
🌟 Problem Description:
Given two integer arrays, the task is to find the length of the longest common prefix of any pair of numbers (one from each array). The numbers are considered to have a common prefix if their digits match from the leftmost side.
🔑 Key Points:
Prefix Matching: Add all the prefixes of the numbers from the first array into a hash set.
Common Prefix Check: For each number in the second array, check if any of its prefixes exists in the hash set.
Efficient Traversal: The problem is solved by iterating through both arrays and reducing the numbers digit by digit to extract the prefixes.
📝 Code Explanation:
Step 1: For each number in the first array, generate all possible prefixes by continuously dividing the number by 10 and add them to a hash set.
Step 2: For each number in the second array, check if any of its prefixes exist in the hash set and track the maximum length of the common prefix.
Helper Function: A helper method is used to calculate the length of a number's digits for comparison.
📅 Daily Solutions: Subscribe for daily LeetCode solutions explained step-by-step in Java!
👥 Join the Community:
Share your thoughts on the problem in the comments. Discuss different approaches with fellow coders. Like, share, and subscribe for more daily coding challenges!
#LeetCode #Coding #Programming #TechInterview #DailyChallenge #LongestCommonPrefix #PrefixMatching #Java
In today's LeetCode daily challenge, we solve the Find the Length of the Longest Common Prefix problem by identifying the longest common prefix between two arrays of integers. The solution is implemented in Java.
👉 Solution: Pinned in the comments.
🌟 Problem Description:
Given two integer arrays, the task is to find the length of the longest common prefix of any pair of numbers (one from each array). The numbers are considered to have a common prefix if their digits match from the leftmost side.
🔑 Key Points:
Prefix Matching: Add all the prefixes of the numbers from the first array into a hash set.
Common Prefix Check: For each number in the second array, check if any of its prefixes exists in the hash set.
Efficient Traversal: The problem is solved by iterating through both arrays and reducing the numbers digit by digit to extract the prefixes.
📝 Code Explanation:
Step 1: For each number in the first array, generate all possible prefixes by continuously dividing the number by 10 and add them to a hash set.
Step 2: For each number in the second array, check if any of its prefixes exist in the hash set and track the maximum length of the common prefix.
Helper Function: A helper method is used to calculate the length of a number's digits for comparison.
📅 Daily Solutions: Subscribe for daily LeetCode solutions explained step-by-step in Java!
👥 Join the Community:
Share your thoughts on the problem in the comments. Discuss different approaches with fellow coders. Like, share, and subscribe for more daily coding challenges!
#LeetCode #Coding #Programming #TechInterview #DailyChallenge #LongestCommonPrefix #PrefixMatching #Java
Комментарии