filmov
tv
Solving the undefined variable Problem in Python
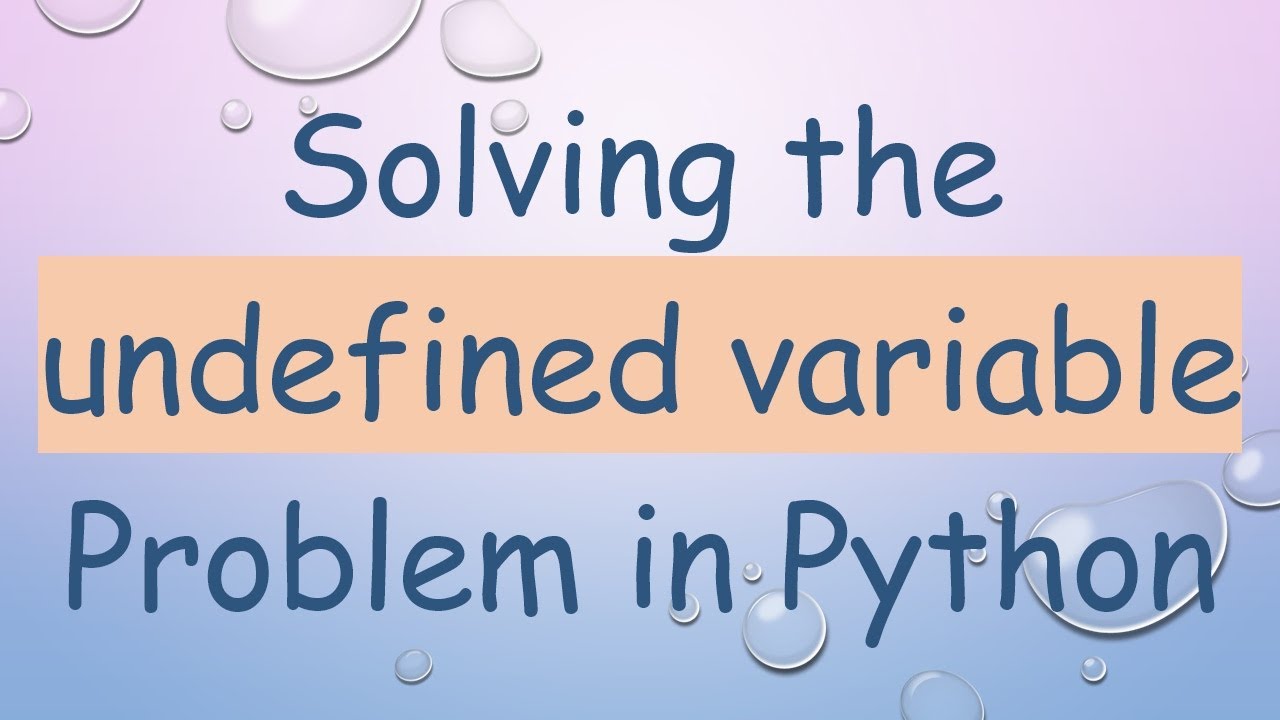
Показать описание
Learn how to fix the `undefined variable` problem in your Python code and enhance your quiz application with effective coding strategies.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: I am having problem in my variable "p. Can someone help me?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding and Fixing the Undefined Variable Problem in Python
When writing code, especially in Python, it's common to encounter errors that can disrupt the functionality of your program. One such problem is related to an undefined variable, which can lead to confusion and frustration. If you’re facing issues with a variable such as "p" in your coding context, this guide is designed to help you understand the problem and how to fix it effectively.
The Problem: Undefined Variable "p"
Imagine you’re developing a quiz application, where users answer questions and score points. However, you notice that the variable "p", which is supposed to represent the correct answer to the questions, remains undefined when you call it in your loop.
Here's a brief example of how this issue arises in a simplified code structure:
[[See Video to Reveal this Text or Code Snippet]]
The challenge lies in the scope of the variable "p". It is defined within the function but is not accessible outside of it. Thus, when you attempt to use "p" in your loop, it throws an undefined error.
Breaking Down the Solution
1. Understanding Variable Scope
In Python, a variable defined within a function is local to that function. Therefore, it is not accessible outside unless returned. This is the key reason the variable "p" remains undefined in your loop.
2. Returning Values from Functions
To make "p" accessible, you need to return the answer from your functions. This way, you can store the return value back in the variable when you call the function.
[[See Video to Reveal this Text or Code Snippet]]
3. Using a List of Functions
Instead of duplicating code, you can create a list of question functions and call them randomly. This will make the code cleaner and more efficient.
Here’s how you can implement this:
[[See Video to Reveal this Text or Code Snippet]]
4. Handling Integer and String Concatenation
Additionally, ensure that when you are concatenating strings and numbers (like the total score), you convert numbers to strings to avoid type errors:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Fixing the undefined variable issue in Python requires understanding variable scope and function returns. By adjusting how you manage your variables and employing effective programming practices, you can enhance your coding efforts and prevent future issues.
It’s all about clear structure and logical flow in your code! With a few adjustments, your quiz application can enhance user experience and function seamlessly. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: I am having problem in my variable "p. Can someone help me?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding and Fixing the Undefined Variable Problem in Python
When writing code, especially in Python, it's common to encounter errors that can disrupt the functionality of your program. One such problem is related to an undefined variable, which can lead to confusion and frustration. If you’re facing issues with a variable such as "p" in your coding context, this guide is designed to help you understand the problem and how to fix it effectively.
The Problem: Undefined Variable "p"
Imagine you’re developing a quiz application, where users answer questions and score points. However, you notice that the variable "p", which is supposed to represent the correct answer to the questions, remains undefined when you call it in your loop.
Here's a brief example of how this issue arises in a simplified code structure:
[[See Video to Reveal this Text or Code Snippet]]
The challenge lies in the scope of the variable "p". It is defined within the function but is not accessible outside of it. Thus, when you attempt to use "p" in your loop, it throws an undefined error.
Breaking Down the Solution
1. Understanding Variable Scope
In Python, a variable defined within a function is local to that function. Therefore, it is not accessible outside unless returned. This is the key reason the variable "p" remains undefined in your loop.
2. Returning Values from Functions
To make "p" accessible, you need to return the answer from your functions. This way, you can store the return value back in the variable when you call the function.
[[See Video to Reveal this Text or Code Snippet]]
3. Using a List of Functions
Instead of duplicating code, you can create a list of question functions and call them randomly. This will make the code cleaner and more efficient.
Here’s how you can implement this:
[[See Video to Reveal this Text or Code Snippet]]
4. Handling Integer and String Concatenation
Additionally, ensure that when you are concatenating strings and numbers (like the total score), you convert numbers to strings to avoid type errors:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Fixing the undefined variable issue in Python requires understanding variable scope and function returns. By adjusting how you manage your variables and employing effective programming practices, you can enhance your coding efforts and prevent future issues.
It’s all about clear structure and logical flow in your code! With a few adjustments, your quiz application can enhance user experience and function seamlessly. Happy coding!