filmov
tv
Generate All Palindromic Decompositions Of A String ('Palindrome Partitioning' on Leetcode)
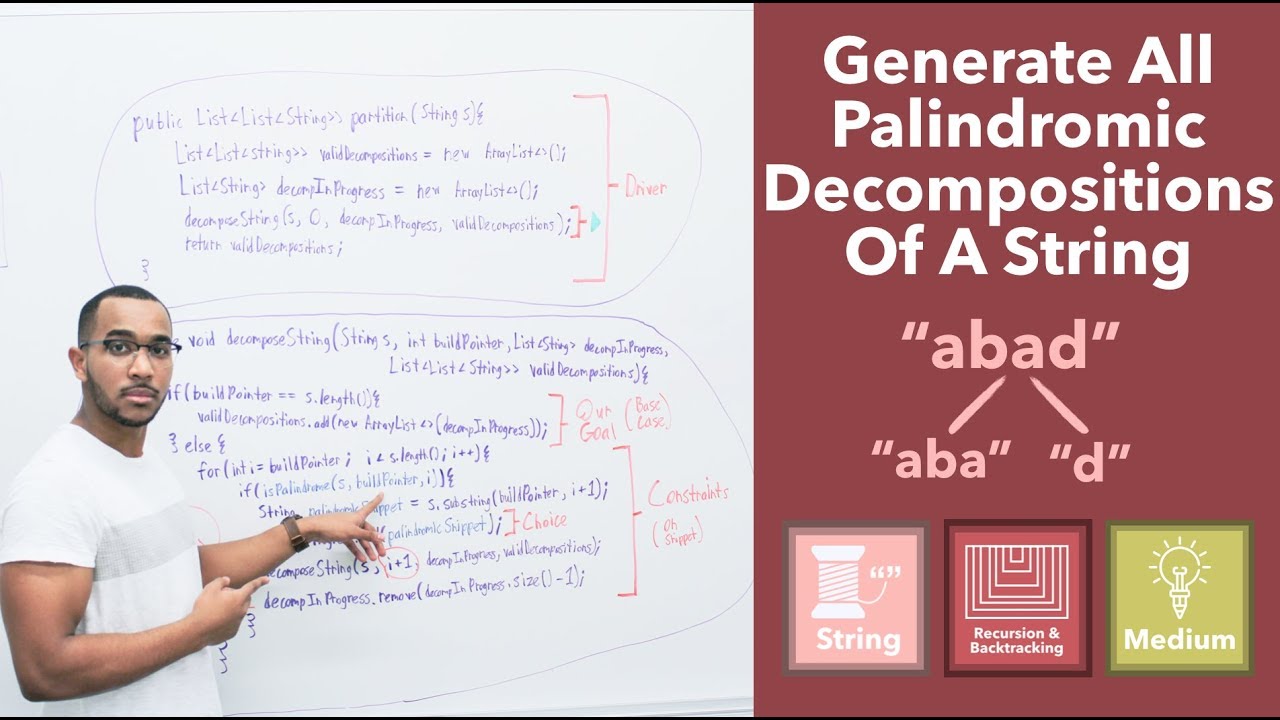
Показать описание
📹 Intuitive Video Explanations
🏃 Run Code As You Learn
💾 Save Progress
❓New Unseen Questions
🔎 Get All Solutions
Question: Given a string, return all decompositions of the string that consist of only palindromes.
Example:
Input: "aab"
Output: [ ["aa","b"], ["a","a","b"] ]
Why is this problem backtracking?
It is backtracking because we will be expressing all of the possibilities of a search space given constraints. When we need to represent many decisions and possibilities, backtracking is the tool you should pull from your pocket.
Approach 1
Generate all decompositions and then only add the palindromic decompositions to our answer.
This is highly exponential in time since there are 2^(n - 1) palindromic decompositions.
This wastes a lot of time since we will continue to build on a decomposition that immediately disqualifies as palindromic.
Approach 2
We will take "snapshots" of snippets as we advance through the string and see if they can add to the decomposition that we want to build.
The 3 Keys To Backtracking:
Our Choice
The start and the end of a "snapshot" that we want to add to a decomposition we are working on.
Our Constraints
Each piece of the decomposition must be a palindrome, we cannot choose and advance on a non-palindrome snippet.
Our Goal
Decompose the whole string. When our decomposition progress index is the length of the array then we know that we have achieved this.
Complexities
Time: O(n * (2^n))
Worst case, but much better than approach 1 since this is a rare worst case where all decompositions turn out to be palindromic (a string of all 1 character).
Our best case becomes greatly improved.
We are basically taking subsets so (2^n) and the O(n) time to copy array to our answer.
Space: O(n)
At worst we will always go n stack frames deep in our recursion since an all single character decomposition is always a palindromic decomposition. This does not include the output array in space.
But let us imagine that we do include the output space.
We will have (2 ^ (n-1)) decompositions of the string.
We will certainly not have (2^n) PALINDROMIC decompositions for the string in a normal case.
The length of each decomposition will very likely not be n in the normal case (n/2 is probably a better approximation but I'm honestly not sure).
So approximate length for each decomposition: (n/2).
MAXIMUM decompositions we could ever make: (2 ^ (n-1)).
So this yields a VERY loose upper bound on the space with the output array included:
O( (n/2) * (2 ^ (n-1)) ) = O( n * 2^n)
REMEMBER THAT n IS THE # OF CHARACTERS IN THE STRING PASSED IN TO US.
I just want to get you thinking about this, it is all about demonstrating that you can think along the right lines and get back on track whether you are exactly right or not.
++++++++++++++++++++++++++++++++++++++++++++++++++
++++++++++++++++++++++++++++++++++++++++++++++++++
This question is number 16.7 in "Elements of Programming Interviews" by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash.
🏃 Run Code As You Learn
💾 Save Progress
❓New Unseen Questions
🔎 Get All Solutions
Question: Given a string, return all decompositions of the string that consist of only palindromes.
Example:
Input: "aab"
Output: [ ["aa","b"], ["a","a","b"] ]
Why is this problem backtracking?
It is backtracking because we will be expressing all of the possibilities of a search space given constraints. When we need to represent many decisions and possibilities, backtracking is the tool you should pull from your pocket.
Approach 1
Generate all decompositions and then only add the palindromic decompositions to our answer.
This is highly exponential in time since there are 2^(n - 1) palindromic decompositions.
This wastes a lot of time since we will continue to build on a decomposition that immediately disqualifies as palindromic.
Approach 2
We will take "snapshots" of snippets as we advance through the string and see if they can add to the decomposition that we want to build.
The 3 Keys To Backtracking:
Our Choice
The start and the end of a "snapshot" that we want to add to a decomposition we are working on.
Our Constraints
Each piece of the decomposition must be a palindrome, we cannot choose and advance on a non-palindrome snippet.
Our Goal
Decompose the whole string. When our decomposition progress index is the length of the array then we know that we have achieved this.
Complexities
Time: O(n * (2^n))
Worst case, but much better than approach 1 since this is a rare worst case where all decompositions turn out to be palindromic (a string of all 1 character).
Our best case becomes greatly improved.
We are basically taking subsets so (2^n) and the O(n) time to copy array to our answer.
Space: O(n)
At worst we will always go n stack frames deep in our recursion since an all single character decomposition is always a palindromic decomposition. This does not include the output array in space.
But let us imagine that we do include the output space.
We will have (2 ^ (n-1)) decompositions of the string.
We will certainly not have (2^n) PALINDROMIC decompositions for the string in a normal case.
The length of each decomposition will very likely not be n in the normal case (n/2 is probably a better approximation but I'm honestly not sure).
So approximate length for each decomposition: (n/2).
MAXIMUM decompositions we could ever make: (2 ^ (n-1)).
So this yields a VERY loose upper bound on the space with the output array included:
O( (n/2) * (2 ^ (n-1)) ) = O( n * 2^n)
REMEMBER THAT n IS THE # OF CHARACTERS IN THE STRING PASSED IN TO US.
I just want to get you thinking about this, it is all about demonstrating that you can think along the right lines and get back on track whether you are exactly right or not.
++++++++++++++++++++++++++++++++++++++++++++++++++
++++++++++++++++++++++++++++++++++++++++++++++++++
This question is number 16.7 in "Elements of Programming Interviews" by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash.
Комментарии