filmov
tv
Minimum Array End - Leetcode 3133 - Python
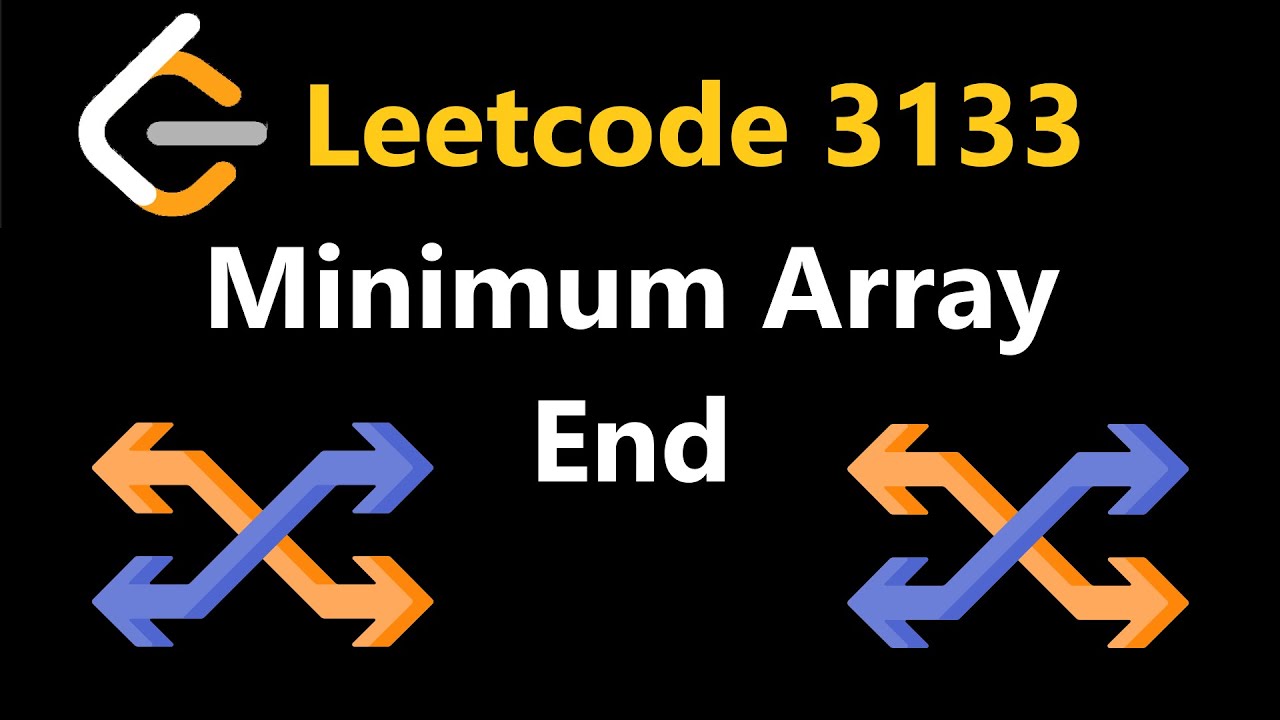
Показать описание
0:00 - Read the problem
0:30 - Drawing Explanation
9:08 - Coding Explanation
10:50 - Drawing Explanation
19:27 - Coding Explanation
leetcode 3133
#neetcode #leetcode #python
Minimum Array End - Leetcode 3133 - Python
Minimum Array End | Leetcode 3133
3133. Minimum Array End (Leetcode Medium)
Minimum Array End | How much you know about AND | Detailed | Leetcode 3133 | codestorywithMIK
3133. Minimum Array End - Day 9/30 Leetcode November Challenge
3133 Minimum Array End || How to 🤔 in Interview || Bit Manipulation + Simulation
Leetcode 3133. Minimum Array End
3133. Minimum Array End | Leetcode Daily (POTD) 9 Nov 2024 | Medium | Java | Hindi
LeetCode 3133 - Minimum Array End - Java
3133. Minimum Array End | Bit Interweaving | Bit Interleaving | Bit Manipulation | 2 Pointers
Minimum Array End - LEETCODE WEEKLY CONTEST 395
leetcode 3133. Minimum Array End
[November 9, 2024, LeetCode daily] 3133. Minimum Array End - Python, solution explained
Minimum Array End | How much you know about AND | Detailed | Leetcode 3133 | SP CREATIVE
LeetCode | 3133. Minimum Array End | Bit Manipulation
LeetCode Problem 3133: Minimum Array End
Minimum Array End | LeetCode 3133 | Java
Leetcode 3133 || Minimum Array End || Java Solution
LeetCode 3133 Minimum Array End
09. Solving leetcode problems with PHP - #3133. Minimum Array End
3133. Minimum Array End | Editorial | Leetcode |#dailychallenge #leetcodechallenge #bitwise
3133. Minimum Array End | LeetCode Daily Solution #coding #leetcodejava #array
How many LeetCode problems should you solve? #leetcode #techinterview #developer #softwareengineer
LeetCode: 3133 Minimum Array End | C++ Solution
Комментарии