filmov
tv
Selection Sort Algorithm Explained (Full Code Included) - Python Algorithms Series for Beginners
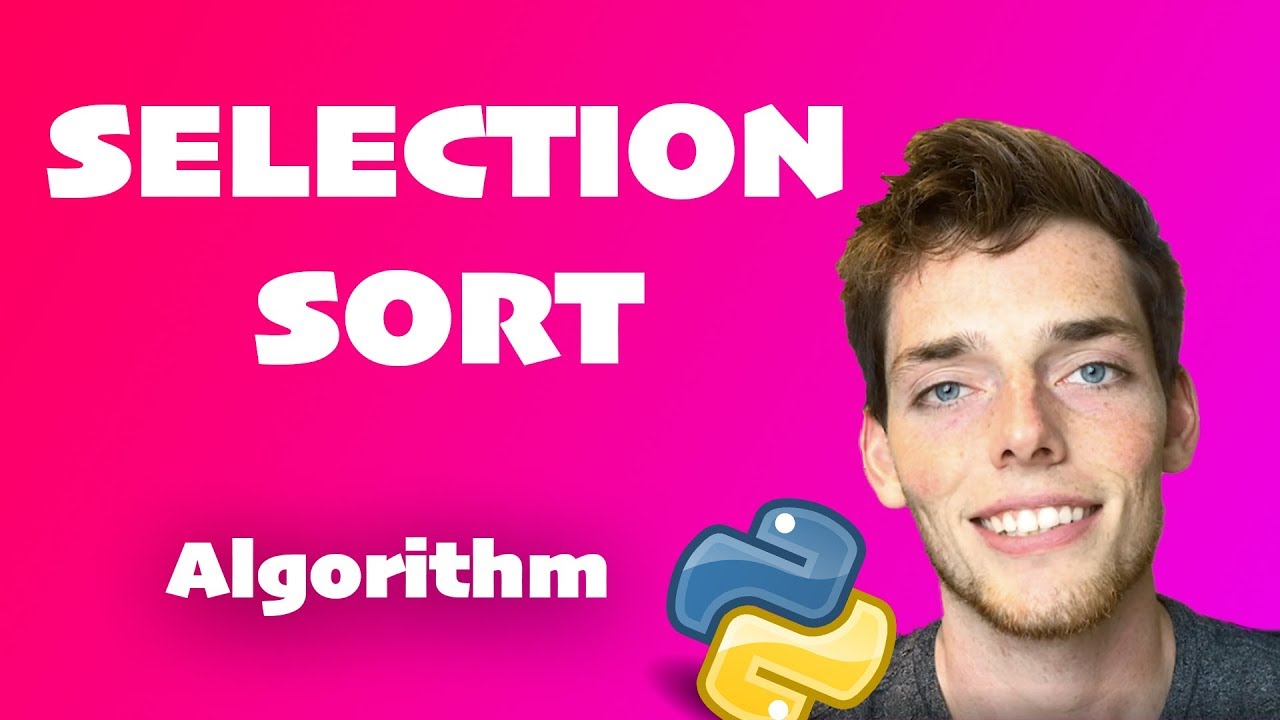
Показать описание
Continuing the Python algorithm series with Selection Sort. Check out the full playlist here:
The Selection Sort Algorithm starts by setting the first item in an unsorted list as the position with the lowest (minimum) value. We then use this to compare each of the items to the right. Whenever we find a new item with a lower value, that becomes our new minimum value and we continue on.
After one iteration of the selection sort algorithm, we create two sublists. One will be for unsorted items and the other will be for sorted ones. We move the minimum item from the unsorted sublist into the last position of our sorted sublist.
After we finish all the iterations, we should be left with only the largest number in our unsorted sublist (which is now sorted to the highest position as it is the highest value.)
The selection sort algorithm has a complexity of O(n^2) still but nearly always outperforms the bubble sort algorithm in situations where writing is important (fewer switches, more efficient per iteration).
If you had any questions about the selection sort algorithm, or any of the other algorithms in this series - please let me know in the comments below!
Join The Socials
Thanks so much for all the support! 5390+ subscribers at the time of writing. Super awesome. I still get so hyped that I get more than 4 views on a new upload. So thankful for you all. I appreciate all the kind words, comments, and support.
#Python #Algorithm #SelectionSort
*****************************************************************
Full code from the video:
def selection_sort(list_a):
indexing_length = range(0, len(list_a)-1)
for i in indexing_length:
min_value = i
for j in range(i+1, len(list_a)):
if list_a[j] #less than list_a[min_value]: #"angled brackets not allowed in youtube description"
min_value = j
if min_value != i:
list_a[min_value], list_a[i] = list_a[i], list_a[min_value]
return list_a
print(selection_sort([7,8,9,8,7,6,5,6,7,8,9,0]))
Packages (& Versions) used in this video:
Python 3.7
Atom Text Editor
*****************************************************************
Code from this tutorial and all my others can be found on my GitHub:
Check out my website:
If you liked the video - please hit the like button. It means more than you know. Thanks for watching and thank you for all your support!!
--- Channel FAQ --
What text editor do you use?
What Equipment do you use to film videos?
What computer do you use/desk setup?
What editing software do you use?
Premiere Pro for video editing
Photoshop for images
After Effects for animations
Do I have any courses available?
Yes & always working on more!
Where do I get my music?
I get all my music from the copyright free Youtube audio library
Let me know if there's anything else you want answered!
-------------------------
Always looking for suggestions on what video to make next -- leave me a comment with your project! Happy Coding!
The Selection Sort Algorithm starts by setting the first item in an unsorted list as the position with the lowest (minimum) value. We then use this to compare each of the items to the right. Whenever we find a new item with a lower value, that becomes our new minimum value and we continue on.
After one iteration of the selection sort algorithm, we create two sublists. One will be for unsorted items and the other will be for sorted ones. We move the minimum item from the unsorted sublist into the last position of our sorted sublist.
After we finish all the iterations, we should be left with only the largest number in our unsorted sublist (which is now sorted to the highest position as it is the highest value.)
The selection sort algorithm has a complexity of O(n^2) still but nearly always outperforms the bubble sort algorithm in situations where writing is important (fewer switches, more efficient per iteration).
If you had any questions about the selection sort algorithm, or any of the other algorithms in this series - please let me know in the comments below!
Join The Socials
Thanks so much for all the support! 5390+ subscribers at the time of writing. Super awesome. I still get so hyped that I get more than 4 views on a new upload. So thankful for you all. I appreciate all the kind words, comments, and support.
#Python #Algorithm #SelectionSort
*****************************************************************
Full code from the video:
def selection_sort(list_a):
indexing_length = range(0, len(list_a)-1)
for i in indexing_length:
min_value = i
for j in range(i+1, len(list_a)):
if list_a[j] #less than list_a[min_value]: #"angled brackets not allowed in youtube description"
min_value = j
if min_value != i:
list_a[min_value], list_a[i] = list_a[i], list_a[min_value]
return list_a
print(selection_sort([7,8,9,8,7,6,5,6,7,8,9,0]))
Packages (& Versions) used in this video:
Python 3.7
Atom Text Editor
*****************************************************************
Code from this tutorial and all my others can be found on my GitHub:
Check out my website:
If you liked the video - please hit the like button. It means more than you know. Thanks for watching and thank you for all your support!!
--- Channel FAQ --
What text editor do you use?
What Equipment do you use to film videos?
What computer do you use/desk setup?
What editing software do you use?
Premiere Pro for video editing
Photoshop for images
After Effects for animations
Do I have any courses available?
Yes & always working on more!
Where do I get my music?
I get all my music from the copyright free Youtube audio library
Let me know if there's anything else you want answered!
-------------------------
Always looking for suggestions on what video to make next -- leave me a comment with your project! Happy Coding!
Комментарии