filmov
tv
Abstract Level 2 |Assignment Set-5|Object Oriented Programming Using Python|NM|Infosys Springboard
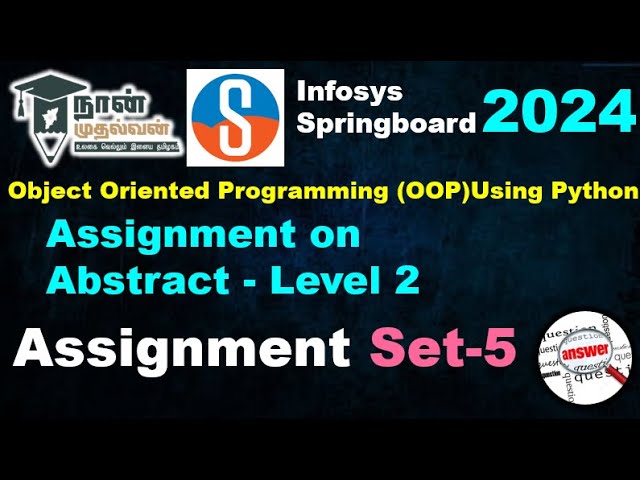
Показать описание
#naanmudhalvan #infosys #springboard
#answers
#objectorientedprogrammingusingpython
#answersdotcom
#abstractlevel2
Assignment on Abstract - Level 2
Problem Statement
Softsytems Ltd is a private firm that provides software solutions to its customers.
The management wants to calculate salary for the employees. There are two types of employees namely graduates who are in probation period and laterals who are experienced joiners in the company.
Write a python program based on the class diagram given below.
Class Description:
Employee class:
validate_basic_salary(): Basic salary of an employee is always more than 3000
If basic salary is valid, return true. Else return false
validate_qualification(): Employee should posses either a "Bachelors" or "Masters" degree
If qualification is valid, return true. Else return false
Graduate class:
validate_job_band(): Graduates can be in "A", "B" or "C" job band
If job band is valid, return true. Else return false
calculate_gross_salary(): Calculate gross salary
Validate basic salary, qualification and job band
If valid,
Compute gross salary as basic salary + PF+ TPI amount + incentive PF is 12% of basic salary Identify TPI amount based on cgpa Identify incentive based on job band.Incentive should be applied on basic salary (Refer tables given)
Return gross salary
Else return -1
Job Band
A
B
C
D
E
F
Incentive %
4
6
10
13
16
20
CGPA
4 to 4.25
4.26 to 4.5
4.51 to 4.75
4.76 to 5
TPI Amount
1000
1700
3200
5000
Lateral class:
validate_job_band(): Laterals can be in "D", "E" or "F" job band
If job band is valid, return true. Else return false
calculate_gross_salary(): Calculate gross salary
Validate basic salary, qualification and job band
If valid,
Compute gross salary as basic salary + PF + SME bonus + incentive PF is 12% of basic salary Identify SME bonus based on skill set Identify incentive based on job band.Incentive should be applied on basic salary (Refer tables given)
Return gross salary
Else return -1
Skill Set
SME Bonus
AGP
6500
AGPT
8200
AGDEV
11500
Perform case sensitive string comparison.
For testing:
Create objects of Graduate and Lateral classes
Invoke calculate_gross_salary() on Graduate and Lateral objects
Display the details
#answers
#objectorientedprogrammingusingpython
#answersdotcom
#abstractlevel2
Assignment on Abstract - Level 2
Problem Statement
Softsytems Ltd is a private firm that provides software solutions to its customers.
The management wants to calculate salary for the employees. There are two types of employees namely graduates who are in probation period and laterals who are experienced joiners in the company.
Write a python program based on the class diagram given below.
Class Description:
Employee class:
validate_basic_salary(): Basic salary of an employee is always more than 3000
If basic salary is valid, return true. Else return false
validate_qualification(): Employee should posses either a "Bachelors" or "Masters" degree
If qualification is valid, return true. Else return false
Graduate class:
validate_job_band(): Graduates can be in "A", "B" or "C" job band
If job band is valid, return true. Else return false
calculate_gross_salary(): Calculate gross salary
Validate basic salary, qualification and job band
If valid,
Compute gross salary as basic salary + PF+ TPI amount + incentive PF is 12% of basic salary Identify TPI amount based on cgpa Identify incentive based on job band.Incentive should be applied on basic salary (Refer tables given)
Return gross salary
Else return -1
Job Band
A
B
C
D
E
F
Incentive %
4
6
10
13
16
20
CGPA
4 to 4.25
4.26 to 4.5
4.51 to 4.75
4.76 to 5
TPI Amount
1000
1700
3200
5000
Lateral class:
validate_job_band(): Laterals can be in "D", "E" or "F" job band
If job band is valid, return true. Else return false
calculate_gross_salary(): Calculate gross salary
Validate basic salary, qualification and job band
If valid,
Compute gross salary as basic salary + PF + SME bonus + incentive PF is 12% of basic salary Identify SME bonus based on skill set Identify incentive based on job band.Incentive should be applied on basic salary (Refer tables given)
Return gross salary
Else return -1
Skill Set
SME Bonus
AGP
6500
AGPT
8200
AGDEV
11500
Perform case sensitive string comparison.
For testing:
Create objects of Graduate and Lateral classes
Invoke calculate_gross_salary() on Graduate and Lateral objects
Display the details
Комментарии