filmov
tv
`Convert Seconds to Days, Hours, Minutes, Seconds` Using Python
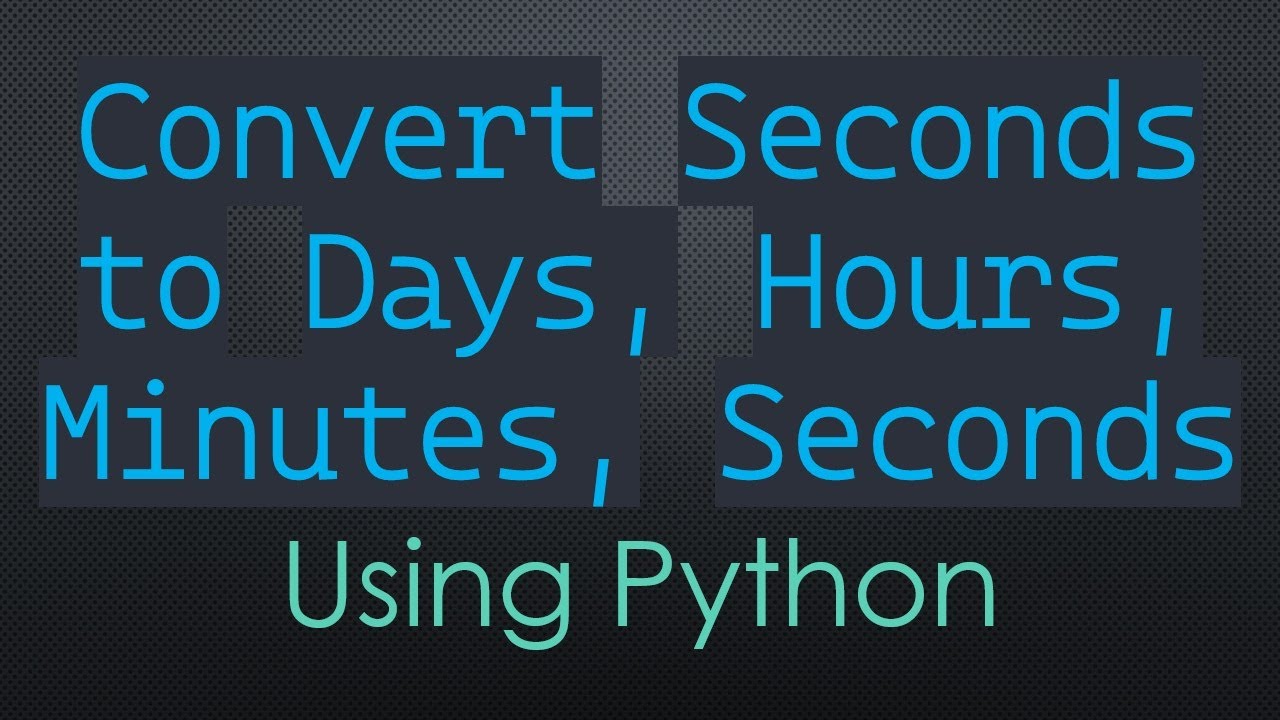
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Looking to convert seconds into days, hours, minutes, and seconds? Learn how to achieve this with a simple Python program.
---
Convert Seconds to Days, Hours, Minutes, and Seconds Using Python
Time conversion is a common requirement in programming, especially when dealing with durations measured in seconds. Whether you're creating a countdown timer, processing timestamps, or simply need to present extensive time intervals in a human-readable format, understanding how to convert seconds into days, hours, minutes, and seconds is essential.
In this guide, we'll discuss how to achieve this conversion effortlessly using Python programming.
Understanding the Basics
Before delving into the Python program, let's grasp the basic concept behind the conversion:
Seconds in a Minute: 60
Seconds in an Hour: 60 minutes x 60 seconds = 3600
Seconds in a Day: 24 hours x 3600 seconds = 86400
By knowing these values, we can decompose a large number of seconds into days, hours, minutes, and seconds.
Python Program to Convert Seconds into Days, Hours, Minutes, and Seconds
Here is a simple Python program to demonstrate this conversion:
[[See Video to Reveal this Text or Code Snippet]]
In this code:
We define a function convert_seconds that takes an integer seconds as an input.
Using floor division (//), we derive the number of days, hours, and minutes.
The modulo operator (%) helps in updating the seconds variable to find the remaining seconds after extracting days, hours, and minutes.
Explanation
Calculate Days:
days = seconds // 86400
This divides the total seconds by 86400 (seconds in a day) to get the number of full days.
Calculate Hours:
Update the remaining seconds using seconds %= 86400
Extract hours by dividing the remaining seconds by 3600 (seconds in an hour).
Calculate Minutes:
Update the remaining seconds using seconds %= 3600
Extract minutes by dividing the remaining seconds by 60.
Extract Remaining Seconds:
After finding days, hours, and minutes, seconds %= 60 will give us the remaining seconds.
By following this approach, you can easily handle the conversion of large time intervals into a more understandable format.
Conclusion
Converting seconds into days, hours, minutes, and seconds is straightforward with Python's arithmetic operations. Whether you are working on event timing or simply need time breakdowns for reporting, this Python program simplifies the task.
Feel free to integrate and adapt this code into your projects for accurate and human-readable time conversions.
---
Summary: Looking to convert seconds into days, hours, minutes, and seconds? Learn how to achieve this with a simple Python program.
---
Convert Seconds to Days, Hours, Minutes, and Seconds Using Python
Time conversion is a common requirement in programming, especially when dealing with durations measured in seconds. Whether you're creating a countdown timer, processing timestamps, or simply need to present extensive time intervals in a human-readable format, understanding how to convert seconds into days, hours, minutes, and seconds is essential.
In this guide, we'll discuss how to achieve this conversion effortlessly using Python programming.
Understanding the Basics
Before delving into the Python program, let's grasp the basic concept behind the conversion:
Seconds in a Minute: 60
Seconds in an Hour: 60 minutes x 60 seconds = 3600
Seconds in a Day: 24 hours x 3600 seconds = 86400
By knowing these values, we can decompose a large number of seconds into days, hours, minutes, and seconds.
Python Program to Convert Seconds into Days, Hours, Minutes, and Seconds
Here is a simple Python program to demonstrate this conversion:
[[See Video to Reveal this Text or Code Snippet]]
In this code:
We define a function convert_seconds that takes an integer seconds as an input.
Using floor division (//), we derive the number of days, hours, and minutes.
The modulo operator (%) helps in updating the seconds variable to find the remaining seconds after extracting days, hours, and minutes.
Explanation
Calculate Days:
days = seconds // 86400
This divides the total seconds by 86400 (seconds in a day) to get the number of full days.
Calculate Hours:
Update the remaining seconds using seconds %= 86400
Extract hours by dividing the remaining seconds by 3600 (seconds in an hour).
Calculate Minutes:
Update the remaining seconds using seconds %= 3600
Extract minutes by dividing the remaining seconds by 60.
Extract Remaining Seconds:
After finding days, hours, and minutes, seconds %= 60 will give us the remaining seconds.
By following this approach, you can easily handle the conversion of large time intervals into a more understandable format.
Conclusion
Converting seconds into days, hours, minutes, and seconds is straightforward with Python's arithmetic operations. Whether you are working on event timing or simply need time breakdowns for reporting, this Python program simplifies the task.
Feel free to integrate and adapt this code into your projects for accurate and human-readable time conversions.