filmov
tv
How to Overcome Time Limit Exceeded in Python Programming
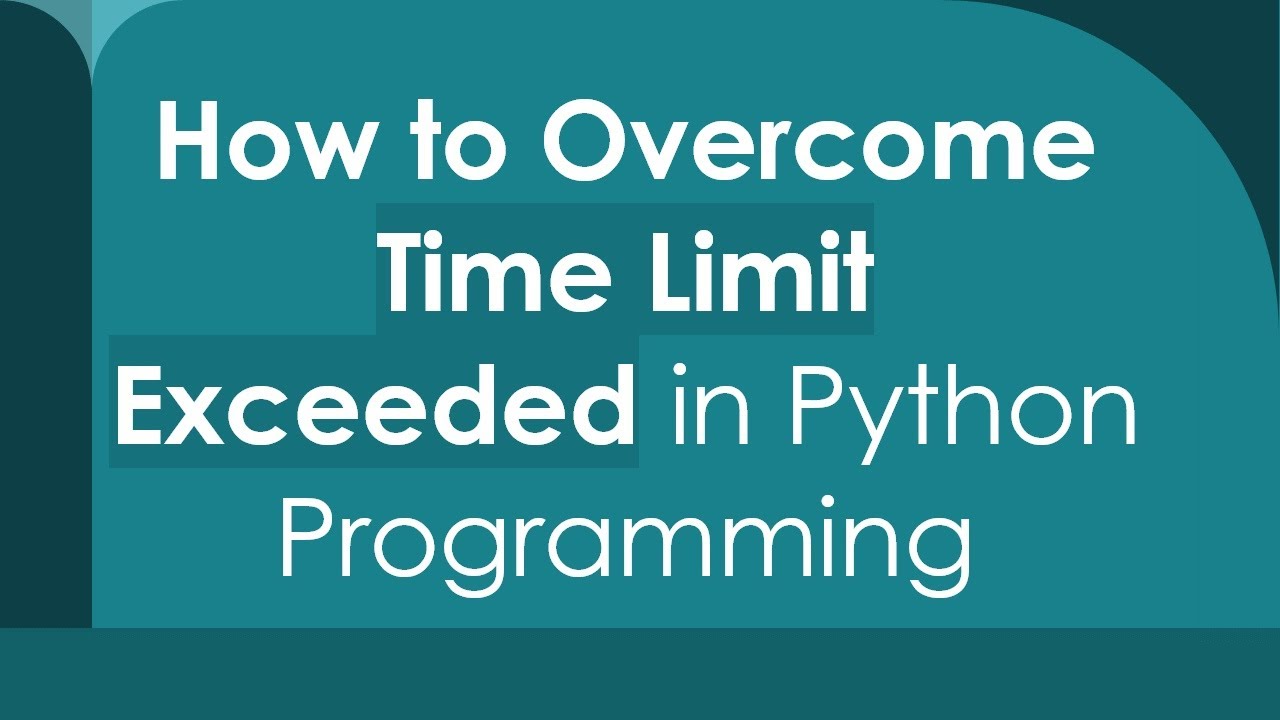
Показать описание
Summary: Facing a `Time Limit Exceeded` error in your Python program? Learn effective strategies and techniques to optimize your code and improve performance right here.
---
How to Overcome Time Limit Exceeded in Python Programming
Encountering a Time Limit Exceeded (TLE) error can be frustrating, especially when you're so close to getting your algorithm to work. This error typically occurs in competitive programming or coding challenges where every millisecond counts. Let's dive into practical methods and techniques to overcome this hurdle in Python.
Understanding the Time Limit Exceeded Error
The TLE error happens when your program takes longer to execute than the allowed time limit. Each problem generally comes with specified constraints and limits, and going beyond these will result in this error. Here are some common causes:
Inefficient Algorithm: The logic you have implemented may not be optimal.
Unnecessary Computations: Redundant or repeated calculations within loops can slow down your program.
I/O Operations: Frequent input and output operations can consume valuable time.
What to Do If Time Limit Exceeded
Optimize Your Algorithms
Focus on refining your algorithm. Go for algorithms with lower time complexity. For instance, using a sorting algorithm with O(n log n) complexity rather than O(n^2) can save precious execution time.
Binary Search: Instead of linear search (O(n)), use binary search (O(log n)) for sorted data.
Dynamic Programming: Helps in reducing the repeated calculations for overlapping subproblems.
Reduce Redundant Calculations
Avoid unnecessary repeated calculations. Use memoization to store and reuse the results of expensive function calls.
[[See Video to Reveal this Text or Code Snippet]]
Efficient Input/Output Operations
Reduce the I/O operation times by using efficient methods.
[[See Video to Reveal this Text or Code Snippet]]
Additional Tips
Breaking Early: If your problem can be solved before considering all elements, break out of loops as early as possible.
[[See Video to Reveal this Text or Code Snippet]]
Using Built-in Functions: Python's built-in functions (sum(), max(), min(), etc.) are highly optimized in C and generally faster than custom implementations.
Conclusion
Overcoming the Time Limit Exceeded error involves understanding the problem constraints and adopting best practices for coding and optimization. By focusing on efficient algorithms, minimizing redundant operations, and opting for effective I/O methods, you can enhance the performance of your Python programs and tackle the TLE error head-on. Always remember, optimizing code is not just about making it run faster but also making it smarter!
Happy coding!
---
How to Overcome Time Limit Exceeded in Python Programming
Encountering a Time Limit Exceeded (TLE) error can be frustrating, especially when you're so close to getting your algorithm to work. This error typically occurs in competitive programming or coding challenges where every millisecond counts. Let's dive into practical methods and techniques to overcome this hurdle in Python.
Understanding the Time Limit Exceeded Error
The TLE error happens when your program takes longer to execute than the allowed time limit. Each problem generally comes with specified constraints and limits, and going beyond these will result in this error. Here are some common causes:
Inefficient Algorithm: The logic you have implemented may not be optimal.
Unnecessary Computations: Redundant or repeated calculations within loops can slow down your program.
I/O Operations: Frequent input and output operations can consume valuable time.
What to Do If Time Limit Exceeded
Optimize Your Algorithms
Focus on refining your algorithm. Go for algorithms with lower time complexity. For instance, using a sorting algorithm with O(n log n) complexity rather than O(n^2) can save precious execution time.
Binary Search: Instead of linear search (O(n)), use binary search (O(log n)) for sorted data.
Dynamic Programming: Helps in reducing the repeated calculations for overlapping subproblems.
Reduce Redundant Calculations
Avoid unnecessary repeated calculations. Use memoization to store and reuse the results of expensive function calls.
[[See Video to Reveal this Text or Code Snippet]]
Efficient Input/Output Operations
Reduce the I/O operation times by using efficient methods.
[[See Video to Reveal this Text or Code Snippet]]
Additional Tips
Breaking Early: If your problem can be solved before considering all elements, break out of loops as early as possible.
[[See Video to Reveal this Text or Code Snippet]]
Using Built-in Functions: Python's built-in functions (sum(), max(), min(), etc.) are highly optimized in C and generally faster than custom implementations.
Conclusion
Overcoming the Time Limit Exceeded error involves understanding the problem constraints and adopting best practices for coding and optimization. By focusing on efficient algorithms, minimizing redundant operations, and opting for effective I/O methods, you can enhance the performance of your Python programs and tackle the TLE error head-on. Always remember, optimizing code is not just about making it run faster but also making it smarter!
Happy coding!