filmov
tv
Python data structure List tuple set and dictionary using List methods and functions
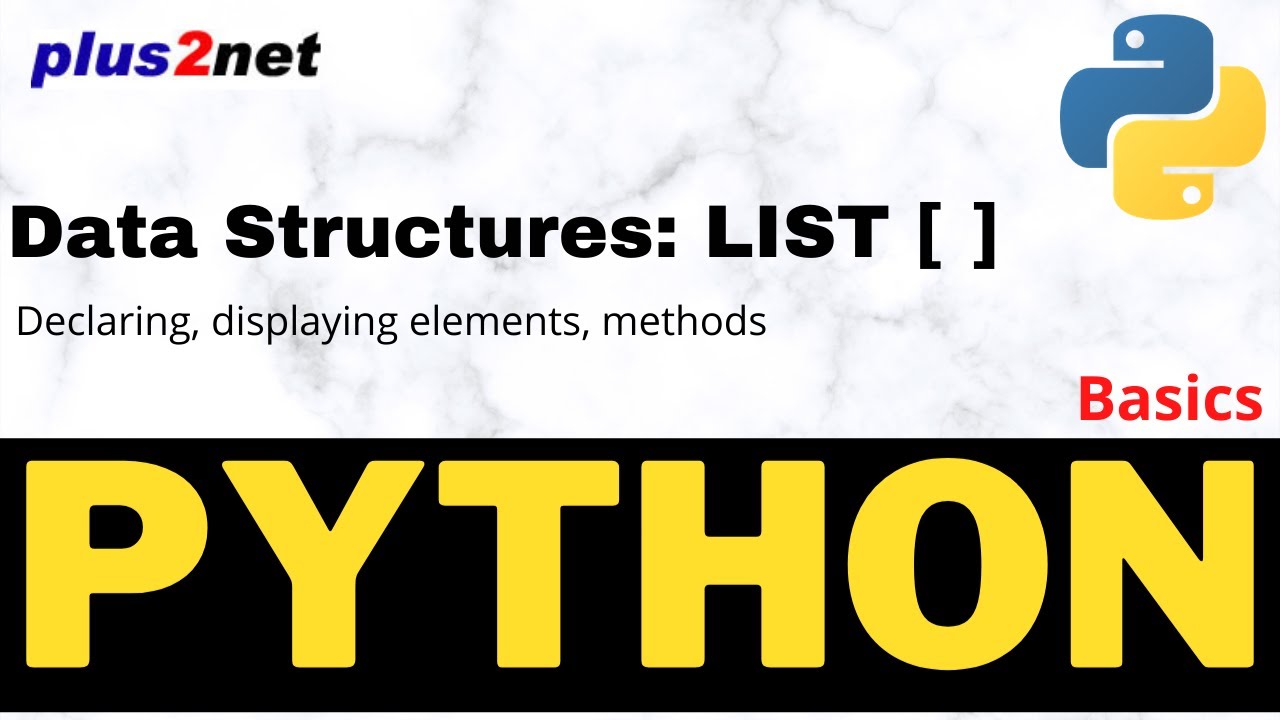
Показать описание
We will be storing multiple data using a single variable like list. There is no concept of array in Python so we will be using these sequential data types to store these data. Here we will discuss more about list in part 1 and then we will move to set , tuple and dictionary in part 2.
Creating a list
We can create an empty list by using its constructor.
my_list=list()
Or we can create like this
my_list=[]
Let us create a list and print the output
my_list=[‘alex’,’Ronn’, ‘John’]
print(my_list)
Using Range to create a list
We can use range to create a list with data from the range. Let us fill a list with elements taken from a range.
x=range(5,50,10)
my_list=list(x)
print(my_list)
Unpacking a list
We can display all elements without any brackets ( as a list ) by using unpacking my_list=['Alex','Ronald','John']
print(*my_list)
Displaying list based on position
Print(my_list[2])
The first element is my_list[0] , second element is my_list[1]. The last element is my_list[-1], all elements starting from 0 position my_list[0:]
All elements from 1 to 4 is my_list[1:4]
Displaying all elements by looping
my_list=['Alex','Ronald','John']
for i in my_list:
print(i)
Methods for list
Append(x) : we can add the element x at the end of the list.
Clear() : removes all the elements from the list
Copy() : Returns a copy of the list.
Count(x) : Returns number of matching x value inside the list
Extend(iterable) : Add one more list
Index(x) : returns position of the input value
Insert(I,x) : adds the value x at the given position
Pop([i]) : add one item to the list
Remove(x) : removes items from the list
Reverse(): reverse the list
Sort(): sort the elements based on key and order.
Using in we can search the presence of an element inside the list.
my_list=['Alex','Ronald','John']
if 'John' in my_list:
print("Yes, included ")
else:
print("No, not included")
We can get total number of elements present inside a list by using len()