filmov
tv
Lesson 12 - Python Programming (Automate the Boring Stuff with Python)
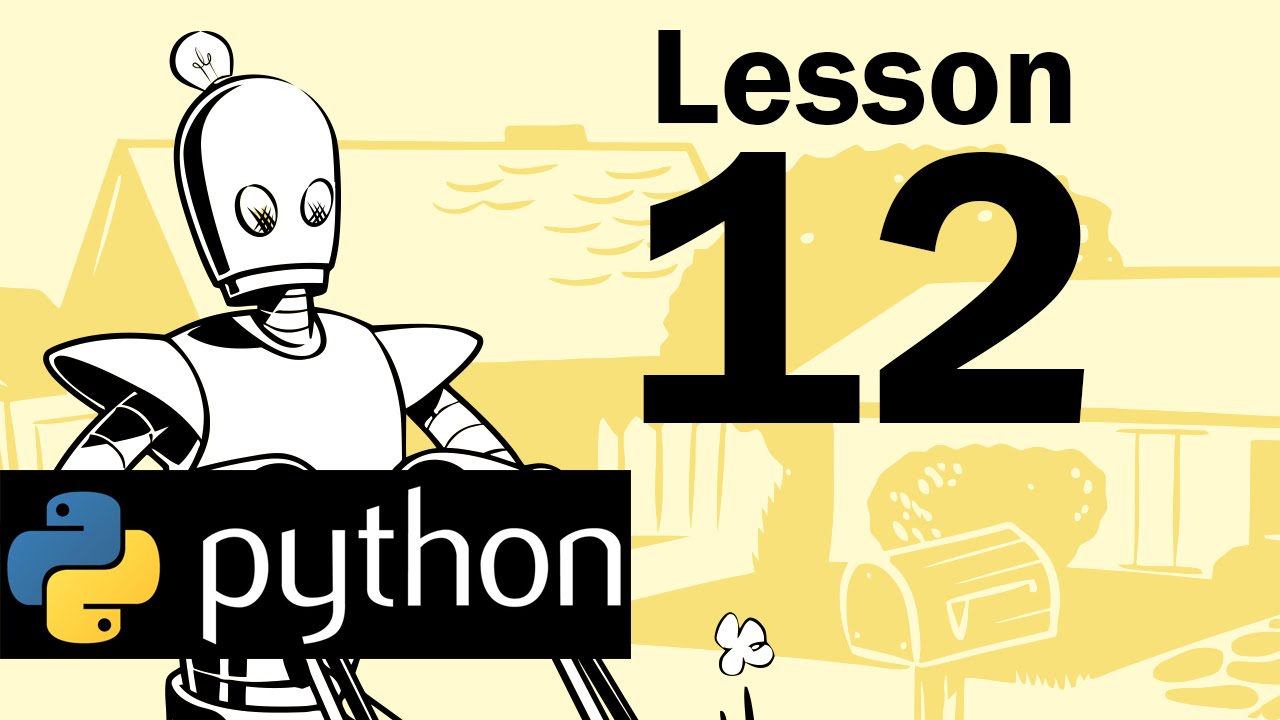
Показать описание
Lesson 12 - Python Programming (Automate the Boring Stuff with Python)
Python Tutorial 12: Simple Python Sorting Program
Lists & Loops - Python | Lesson 12 (Free Coding Bootcamp)
Strings Slicing and Operations on Strings in Python | Python Tutorial - Day #12
Arduino with Python LESSON 12: Calculating Height from Changes in Pressure
Radio Buttons with TKinter - Python Tkinter GUI Tutorial #12
Python Programming Tutorial #12 - Functions
range Function in Python || lesson 12 || Python || Learning Monkey ||
Kontakt Builder KSP Scripting Course Tutorials - Lesson 12 (Learning And Resources)
Tutorial 12- Python Functions, Positional and Keywords Arguments
Guess The Python Output! #python #programming #coding
List Comprehension Basics with Python (Python Tutorial #12)
#20 Python Tutorial for Beginners | While Loop in Python
Python Tutorial #12 - Funktionen
9-Axis IMU LESSON 12: Passing Data From Arduino to Python
GCSE Python Programming Lesson 12 Reading Files
python lesson 12 function
Beaglebone Black LESSON 12: Controlling Servo from Python Using PWM
Python Beginner Tutorial #12 - Useful Functions
Python Lesson 12 - Running Totals
Python Programming Tutorial - 12 - Functions
Functions in Python are easy 📞
Python Tutorial for Beginners - Full Course in 12 Hours (2022)
List Functions | Python | Tutorial 12
Комментарии