filmov
tv
FASTER Ray Tracing with Multithreading // Ray Tracing series
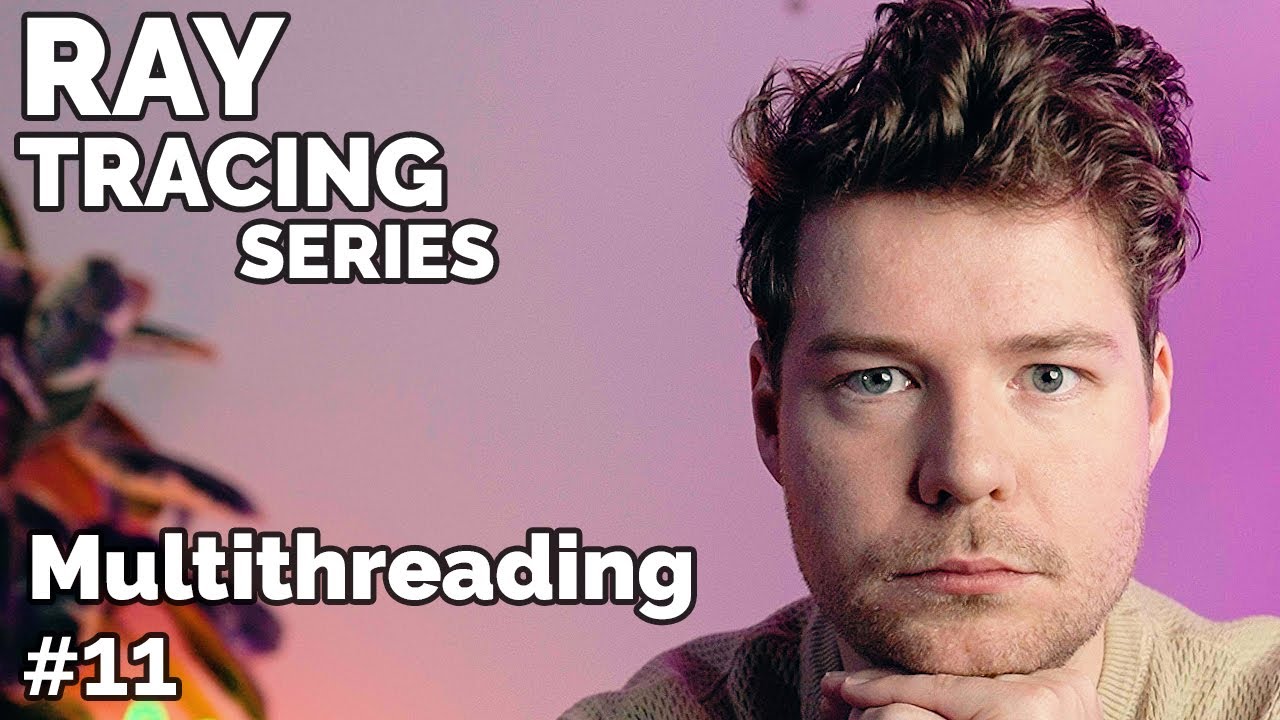
Показать описание
🧭 FOLLOW ME
📚 RESOURCES (in order of complexity)
💾 SOFTWARE you'll need installed to follow this series
CHAPTERS
0:00 - Optimization
1:25 - Multithreading and Rendering
7:05 - Making our code multithreaded
Welcome to the exciting new Ray Tracing Series! Ray tracing is very common technique for generating photo-realistic digital imagery, which is exactly what we'll be doing in this series. Aside from learning all about ray tracing and the math to goes into it, as well as how to implement it, we'll also be focusing on performance and optimization in C++ to make our renderer as efficient as possible. We'll eventually switch to using the GPU instead of the CPU (using Vulkan) to run our ray tracing algorithms, as this will be much faster that using the CPU. This will also be a great introduction to leveraging the power of the GPU in the software you write. All of the code episode-by-episode will be released, and if you need help check out the raytracing-series channel on my Discord server. I'm really looking forward to this series and I hope you are too! ❤️
This video is sponsored by Brilliant.
#RayTracing
Комментарии