filmov
tv
How to Update a JSON File from a Python Script
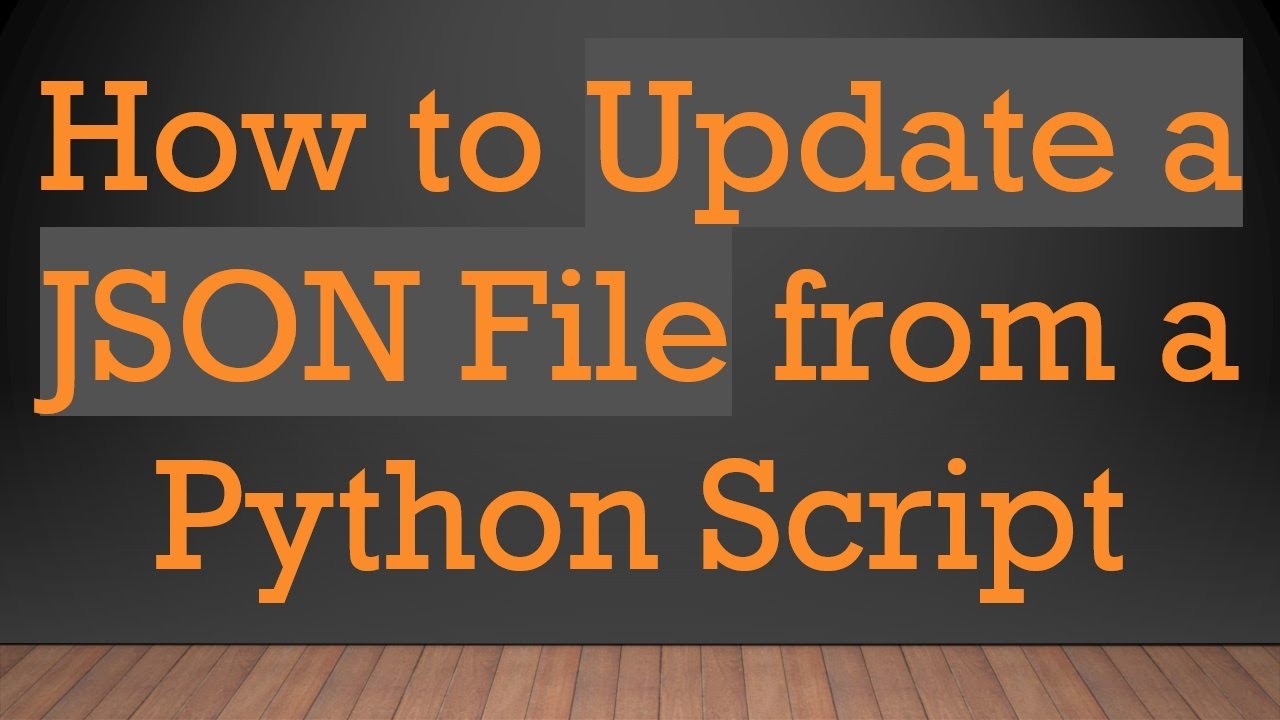
Показать описание
Learn how to efficiently update values in a JSON file using Python. This guide provides clear steps and code examples to help you manage JSON data effectively.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Update Json file from Python script
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Update a JSON File from a Python Script
Working with JSON files is a common task in Python programming, especially when dealing with configuration files or data interchange between client and server. One common issue that developers encounter is updating values in an existing JSON file. If you’ve ever tried to update a specific element within your JSON structure and ended up creating new entries instead, you’re not alone. In this post, we’ll walk you through the steps to correctly update the values in a JSON file using a Python script.
Understanding the JSON Structure
Before diving into the solution, let’s familiarize ourselves with the sample JSON structure we’re dealing with. Here’s the format of the JSON file you might encounter:
[[See Video to Reveal this Text or Code Snippet]]
In the above example, we have a list containing multiple dictionaries. Each dictionary holds key-value pairs. Our goal is to update the value of key2 from "value2" to "newValue" for each item without creating any new entries.
Steps to Update the JSON File
To successfully update the JSON file, we’ll follow a three-step approach: Read, Modify, and Write. Below are detailed instructions on how to implement each step.
1. Reading the JSON File
[[See Video to Reveal this Text or Code Snippet]]
with statement: This ensures the file is properly closed after its block is executed, even if an error occurs.
data: This variable now holds the parsed JSON as a Python dictionary.
2. Modifying the Content
After loading the JSON data, we will loop through the list and update the key2 value as needed. With the update() method, we can easily modify existing values.
[[See Video to Reveal this Text or Code Snippet]]
In this loop, each item represent a dictionary within the list, allowing us to access and update its values directly.
3. Writing the Updated Data Back to the File
[[See Video to Reveal this Text or Code Snippet]]
Opening in write mode ('w') ensures that the old content is replaced with the updated content.
This step effectively saves the modified data, and we now have our desired output.
Complete Code Example
Bringing it all together, here’s a complete code snippet to update the JSON file:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following these steps, you can easily update values within your JSON files using a Python script. This method not only ensures that you modify the existing data without creating duplications but also keeps your JSON structured and organized. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Update Json file from Python script
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Update a JSON File from a Python Script
Working with JSON files is a common task in Python programming, especially when dealing with configuration files or data interchange between client and server. One common issue that developers encounter is updating values in an existing JSON file. If you’ve ever tried to update a specific element within your JSON structure and ended up creating new entries instead, you’re not alone. In this post, we’ll walk you through the steps to correctly update the values in a JSON file using a Python script.
Understanding the JSON Structure
Before diving into the solution, let’s familiarize ourselves with the sample JSON structure we’re dealing with. Here’s the format of the JSON file you might encounter:
[[See Video to Reveal this Text or Code Snippet]]
In the above example, we have a list containing multiple dictionaries. Each dictionary holds key-value pairs. Our goal is to update the value of key2 from "value2" to "newValue" for each item without creating any new entries.
Steps to Update the JSON File
To successfully update the JSON file, we’ll follow a three-step approach: Read, Modify, and Write. Below are detailed instructions on how to implement each step.
1. Reading the JSON File
[[See Video to Reveal this Text or Code Snippet]]
with statement: This ensures the file is properly closed after its block is executed, even if an error occurs.
data: This variable now holds the parsed JSON as a Python dictionary.
2. Modifying the Content
After loading the JSON data, we will loop through the list and update the key2 value as needed. With the update() method, we can easily modify existing values.
[[See Video to Reveal this Text or Code Snippet]]
In this loop, each item represent a dictionary within the list, allowing us to access and update its values directly.
3. Writing the Updated Data Back to the File
[[See Video to Reveal this Text or Code Snippet]]
Opening in write mode ('w') ensures that the old content is replaced with the updated content.
This step effectively saves the modified data, and we now have our desired output.
Complete Code Example
Bringing it all together, here’s a complete code snippet to update the JSON file:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following these steps, you can easily update values within your JSON files using a Python script. This method not only ensures that you modify the existing data without creating duplications but also keeps your JSON structured and organized. Happy coding!