filmov
tv
Solving the TypeError When Accessing a JSON Object in JavaScript
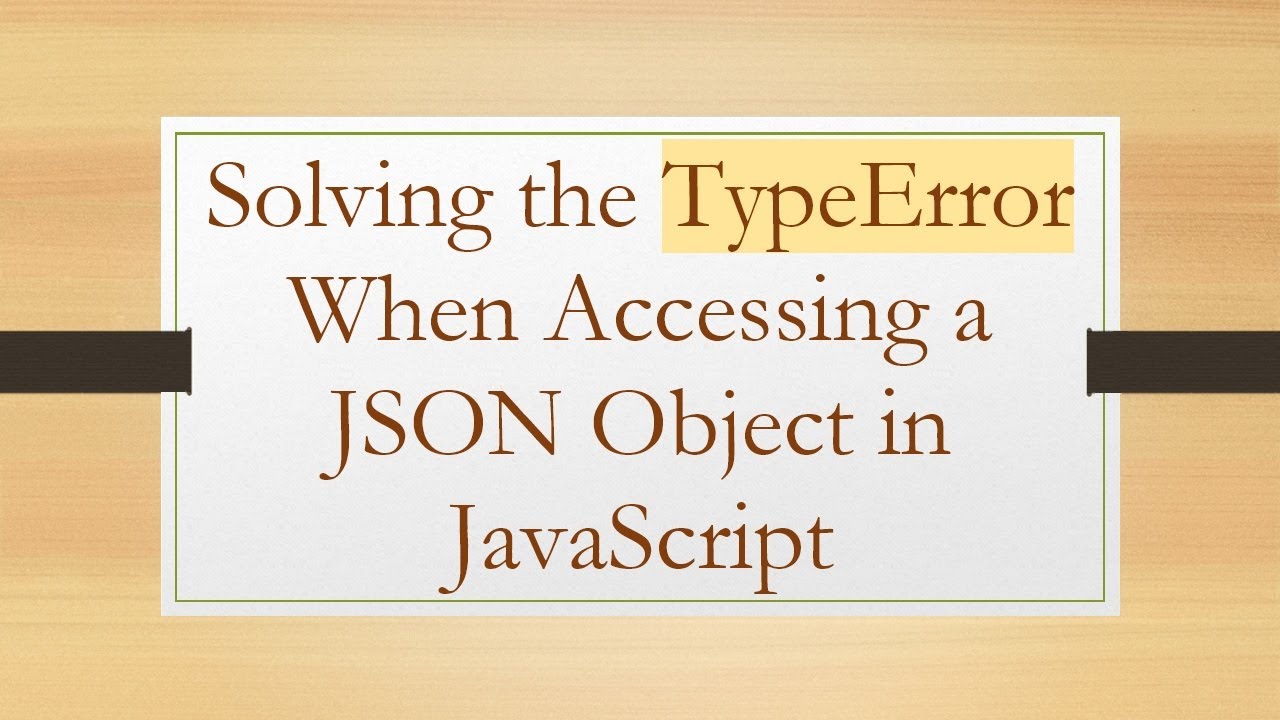
Показать описание
Discover how to debug and solve the `TypeError: Cannot read properties of undefined` when working with JSON objects in JavaScript.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Error while accessing variable of a JSON object
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding and Fixing TypeError in JSON Object Access with JavaScript
When working with APIs in JavaScript, especially using the async/await pattern, developers can sometimes encounter puzzling errors. One common issue is the TypeError stating that properties of undefined cannot be read. This guide addresses this problem by delving into a common scenario involving GIF retrieval from the GIPHY API and how to overcome this error effectively.
The Problem
In the given scenario, a developer is trying to access the URL of a GIF image from a JSON object returned by the GIPHY API. The method used returns a TypeError, specifically when the code attempts to execute this line:
[[See Video to Reveal this Text or Code Snippet]]
The error message states: TypeError: Cannot read properties of undefined (reading 'images'). This indicates that the images property is likely undefined at the time the code is executed, even though the size property seems accessible.
What Could Be Causing This Error?
Network Issues: Sometimes, network issues can result in an incomplete or erroneous response from the API.
Wrong Endpoint or Key: If the API key is invalid or the endpoint is misconfigured, it could also lead to unexpected results.
Data Structure Changes: If the structure of the data returned by the API has changed or differs from what is expected, this kind of error can occur.
Handling Empty Responses: If the API doesn't return any GIFs for the given search query, responseJSON.data[0] would be undefined.
The Solution
To effectively troubleshoot and fix the issue, it’s essential to implement debugging steps that help monitor the responses received from the API and ensure that the expected data structure is achieved at each stage.
Step 1: Inspecting the Response
Modify the gif function to log the details of wordJSON and responseJSON right after fetching them. This allows you to see what your application gets each time:
[[See Video to Reveal this Text or Code Snippet]]
By logging these details, you can check if responseJSON.data[0] exists and if images is defined before trying to access its properties.
Step 2: Validate Response Data
Before accessing images, add a check to ensure that the expected data structure is present:
[[See Video to Reveal this Text or Code Snippet]]
This check ensures that you only attempt to access images if data[0] and images are indeed available, preventing the TypeError from occurring.
Step 3: Review Fetch Requests
Make sure that:
The API key is valid and has proper access to the data requested.
Both APIs are reachable and mitigating errors by implementing robust error handling.
Conclusion
Debugging API responses can be complex, especially when the underlying data structure is not as expected. By logging responses, validating data, and ensuring error handling, you can effectively manage issues like the TypeError: Cannot read properties of undefined. Remember, thoughtful debugging is the key to solving complex problems in JavaScript applications.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Error while accessing variable of a JSON object
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding and Fixing TypeError in JSON Object Access with JavaScript
When working with APIs in JavaScript, especially using the async/await pattern, developers can sometimes encounter puzzling errors. One common issue is the TypeError stating that properties of undefined cannot be read. This guide addresses this problem by delving into a common scenario involving GIF retrieval from the GIPHY API and how to overcome this error effectively.
The Problem
In the given scenario, a developer is trying to access the URL of a GIF image from a JSON object returned by the GIPHY API. The method used returns a TypeError, specifically when the code attempts to execute this line:
[[See Video to Reveal this Text or Code Snippet]]
The error message states: TypeError: Cannot read properties of undefined (reading 'images'). This indicates that the images property is likely undefined at the time the code is executed, even though the size property seems accessible.
What Could Be Causing This Error?
Network Issues: Sometimes, network issues can result in an incomplete or erroneous response from the API.
Wrong Endpoint or Key: If the API key is invalid or the endpoint is misconfigured, it could also lead to unexpected results.
Data Structure Changes: If the structure of the data returned by the API has changed or differs from what is expected, this kind of error can occur.
Handling Empty Responses: If the API doesn't return any GIFs for the given search query, responseJSON.data[0] would be undefined.
The Solution
To effectively troubleshoot and fix the issue, it’s essential to implement debugging steps that help monitor the responses received from the API and ensure that the expected data structure is achieved at each stage.
Step 1: Inspecting the Response
Modify the gif function to log the details of wordJSON and responseJSON right after fetching them. This allows you to see what your application gets each time:
[[See Video to Reveal this Text or Code Snippet]]
By logging these details, you can check if responseJSON.data[0] exists and if images is defined before trying to access its properties.
Step 2: Validate Response Data
Before accessing images, add a check to ensure that the expected data structure is present:
[[See Video to Reveal this Text or Code Snippet]]
This check ensures that you only attempt to access images if data[0] and images are indeed available, preventing the TypeError from occurring.
Step 3: Review Fetch Requests
Make sure that:
The API key is valid and has proper access to the data requested.
Both APIs are reachable and mitigating errors by implementing robust error handling.
Conclusion
Debugging API responses can be complex, especially when the underlying data structure is not as expected. By logging responses, validating data, and ensuring error handling, you can effectively manage issues like the TypeError: Cannot read properties of undefined. Remember, thoughtful debugging is the key to solving complex problems in JavaScript applications.