filmov
tv
Mastering UTC and GMT Date Parsing in JavaScript
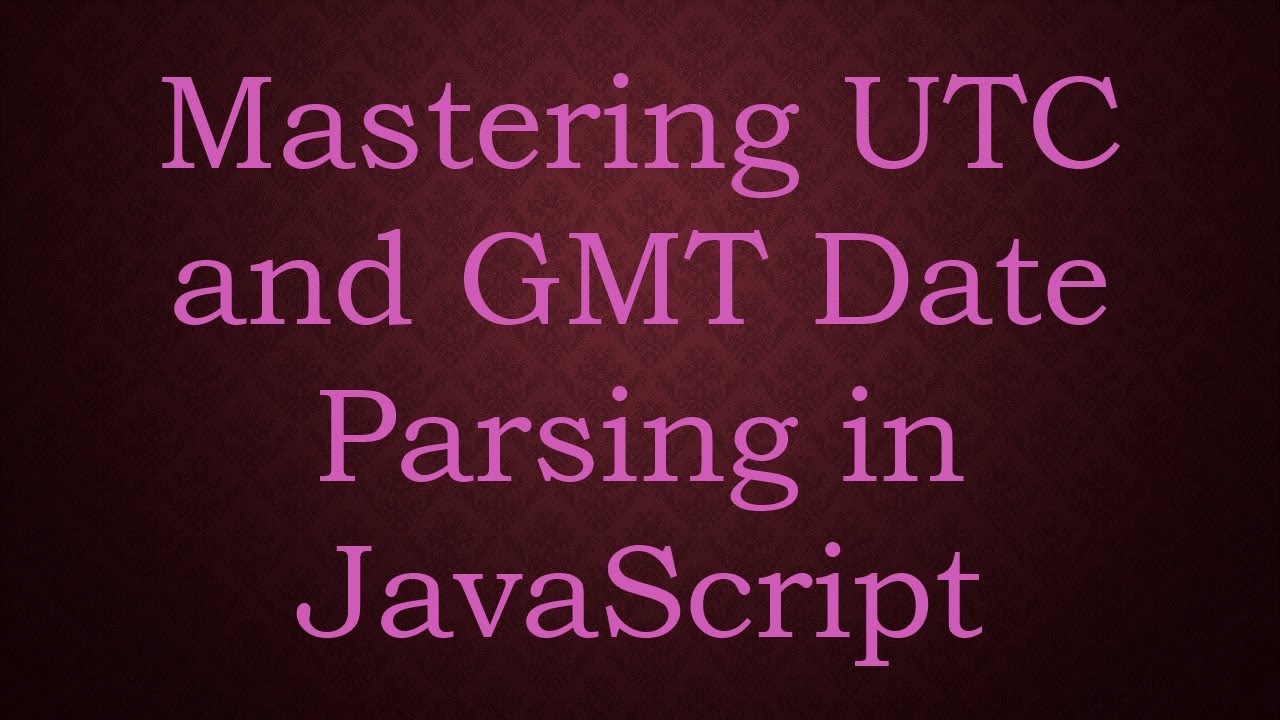
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to parse UTC and GMT dates and timestamps in JavaScript effectively. This guide covers various methods to handle dates in universal formats like UTC and GMT.
---
Mastering UTC and GMT Date Parsing in JavaScript
Parsing dates and times in JavaScript can get confusing, especially when dealing with universal formats like UTC and GMT. Understanding how to effectively parse these dates is crucial for ensuring your web applications handle time data correctly. This guide will guide you through the various methods for parsing UTC and GMT dates and timestamps in JavaScript.
Parsing a UTC Date in JavaScript
When dealing with dates and times in JavaScript, the Date object is your go-to tool. To parse a UTC date, you can directly utilize the Date constructor with a UTC date string. Here's an example:
[[See Video to Reveal this Text or Code Snippet]]
The Z at the end of the date string denotes that the time is in UTC. The Date object will correctly interpret this and store the date in UTC.
Parsing a UTC Timestamp
If you're working with timestamps, the process is just as straightforward. JavaScript timestamps are already in milliseconds from the Unix epoch in UTC. To parse a UTC timestamp, simply pass it to the Date constructor:
[[See Video to Reveal this Text or Code Snippet]]
By default, the Date object will interpret the timestamp as a UTC timestamp and create a date accordingly.
Forcing a Date to be Parsed as UTC
If you're working with local date strings but need to parse them as UTC, you need to manipulate the string before passing it to the Date constructor. Here's a solution using a custom function:
[[See Video to Reveal this Text or Code Snippet]]
This function ensures that the date string is treated as a UTC date string by appending the Z to enforce the UTC interpretation.
Parsing a GMT Date in JavaScript
While UTC and GMT are often used interchangeably, there can be slight differences in their interpretations. However, JavaScript's Date object treats both the same. To parse a GMT date, you can use the same techniques as for UTC dates:
[[See Video to Reveal this Text or Code Snippet]]
Just like with UTC, the Date constructor recognizes the GMT suffix and parses the date accordingly.
Working with Different Time Zones
Although our main focus has been on UTC and GMT, it's important to mention that parsing dates from various time zones may require similar adjustments to your date strings. The key is specifying the time zone in the string format.
Conclusion
Handling UTC and GMT date formats in JavaScript shouldn't be daunting. By understanding how to utilize the Date object for parsing, you can ensure that your applications correctly interpret and manipulate date and time data regardless of the format.
In summary:
Use ISO 8601 date strings (yyyy-mm-ddThh:mm:ssZ) for straightforward UTC parsing.
Timestamps are treated as UTC by default.
To enforce a UTC interpretation for different formats, modify the date string to include Z or GMT.
For different time zones, specify the time zone in the date string.
By following these guidelines, you'll be well-equipped to handle UTC and GMT date parsing in JavaScript.
---
Summary: Learn how to parse UTC and GMT dates and timestamps in JavaScript effectively. This guide covers various methods to handle dates in universal formats like UTC and GMT.
---
Mastering UTC and GMT Date Parsing in JavaScript
Parsing dates and times in JavaScript can get confusing, especially when dealing with universal formats like UTC and GMT. Understanding how to effectively parse these dates is crucial for ensuring your web applications handle time data correctly. This guide will guide you through the various methods for parsing UTC and GMT dates and timestamps in JavaScript.
Parsing a UTC Date in JavaScript
When dealing with dates and times in JavaScript, the Date object is your go-to tool. To parse a UTC date, you can directly utilize the Date constructor with a UTC date string. Here's an example:
[[See Video to Reveal this Text or Code Snippet]]
The Z at the end of the date string denotes that the time is in UTC. The Date object will correctly interpret this and store the date in UTC.
Parsing a UTC Timestamp
If you're working with timestamps, the process is just as straightforward. JavaScript timestamps are already in milliseconds from the Unix epoch in UTC. To parse a UTC timestamp, simply pass it to the Date constructor:
[[See Video to Reveal this Text or Code Snippet]]
By default, the Date object will interpret the timestamp as a UTC timestamp and create a date accordingly.
Forcing a Date to be Parsed as UTC
If you're working with local date strings but need to parse them as UTC, you need to manipulate the string before passing it to the Date constructor. Here's a solution using a custom function:
[[See Video to Reveal this Text or Code Snippet]]
This function ensures that the date string is treated as a UTC date string by appending the Z to enforce the UTC interpretation.
Parsing a GMT Date in JavaScript
While UTC and GMT are often used interchangeably, there can be slight differences in their interpretations. However, JavaScript's Date object treats both the same. To parse a GMT date, you can use the same techniques as for UTC dates:
[[See Video to Reveal this Text or Code Snippet]]
Just like with UTC, the Date constructor recognizes the GMT suffix and parses the date accordingly.
Working with Different Time Zones
Although our main focus has been on UTC and GMT, it's important to mention that parsing dates from various time zones may require similar adjustments to your date strings. The key is specifying the time zone in the string format.
Conclusion
Handling UTC and GMT date formats in JavaScript shouldn't be daunting. By understanding how to utilize the Date object for parsing, you can ensure that your applications correctly interpret and manipulate date and time data regardless of the format.
In summary:
Use ISO 8601 date strings (yyyy-mm-ddThh:mm:ssZ) for straightforward UTC parsing.
Timestamps are treated as UTC by default.
To enforce a UTC interpretation for different formats, modify the date string to include Z or GMT.
For different time zones, specify the time zone in the date string.
By following these guidelines, you'll be well-equipped to handle UTC and GMT date parsing in JavaScript.