filmov
tv
How to Efficiently Filter JSON Data by Specific Keywords in JavaScript
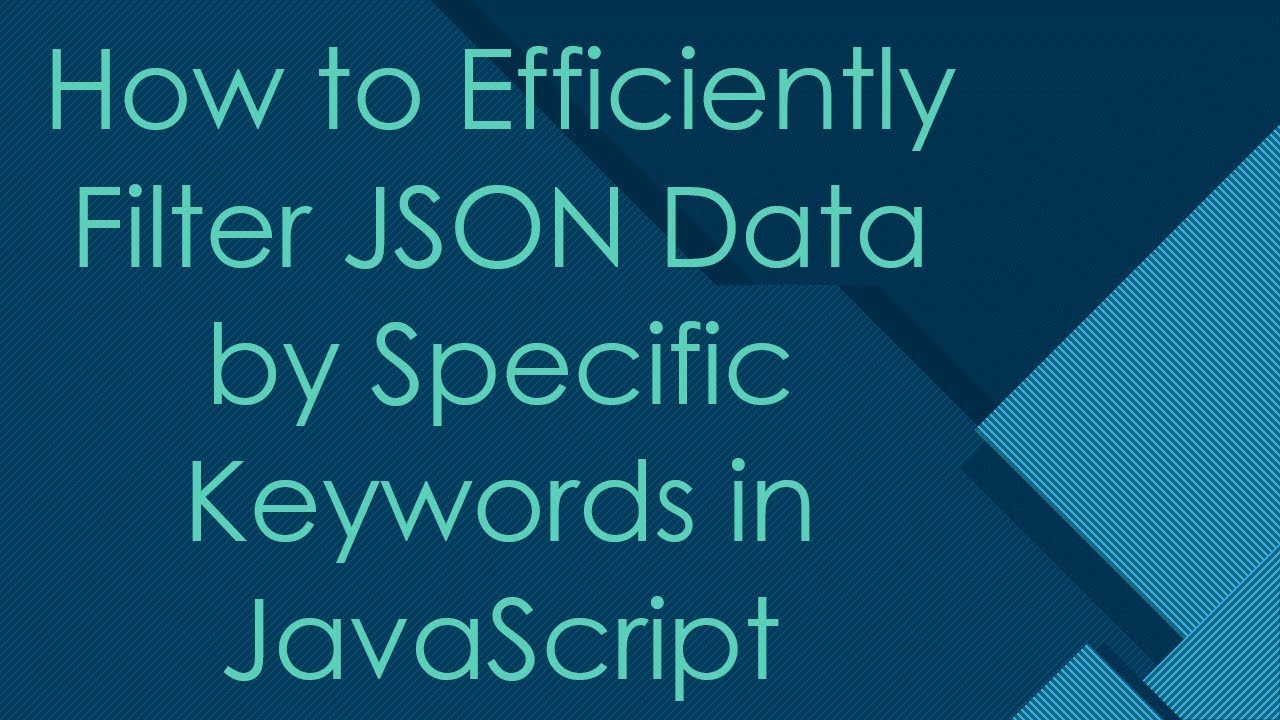
Показать описание
Discover how to filter JSON data to display specific fields based on certain keywords, with practical examples in JavaScript.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to filter JSON data to show fields by certain words?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Efficiently Filter JSON Data by Specific Keywords in JavaScript
If you've ever worked with JSON data, you might have faced the challenge of filtering through it to find specific information. For instance, you may need to extract URLs from a JSON response based on certain keywords present in the data. In this guide, we will explore how to filter JSON data to display only the fields that match specified keywords using JavaScript.
Understanding JSON Structure
Before diving into the solution, let's break down an example JSON structure to understand how to extract the required information. Here we have a sample JSON object containing links and nested thumbnail data:
[[See Video to Reveal this Text or Code Snippet]]
In this JSON structure, each thumbnail object includes a URL (href) and an array of relationship identifiers (rel). Our goal is to filter this data based on keywords present in the rel array.
Step-by-Step Solution
1. Filtering Based on Keywords
To filter the JSON data, we can use JavaScript's array .filter() method along with .includes(). This approach allows us to filter the thumbnail entries depending on whether a specific keyword is present in the rel array.
Here’s how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
In this snippet, filterBy is a function that accepts a keyword (toFilter). It filters the thumbnail array to return only those objects where the rel array includes the specified keyword.
2. Extracting Only the URLs
If you're only interested in extracting the URLs (href) from the objects that match your filter criteria, you can chain the .map() method to the filter function:
[[See Video to Reveal this Text or Code Snippet]]
This function, filterByUrls, will return an array of URLs corresponding to the filtered objects.
3. Excluding Certain Keywords
Sometimes, you may want to also exclude certain keywords. This can easily be implemented by modifying our filter function a bit more:
[[See Video to Reveal this Text or Code Snippet]]
In this modification, if toExclude is set to true, the function will return thumbnails that do not include the specified keyword.
Practical Examples
Let's see these newly defined functions in action:
Filtering for 'twitter':
[[See Video to Reveal this Text or Code Snippet]]
Excluding 'twitter':
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Filtering JSON data in JavaScript is a straightforward task that becomes incredibly useful when handling large datasets. By using .filter() and .map(), you can efficiently extract the information you need based on specific keywords. Depending on your requirements, you can also modify the filter conditions to include or exclude certain values.
Now that you have the tools at your disposal, feel free to experiment with more complex JSON structures and filtering conditions. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to filter JSON data to show fields by certain words?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Efficiently Filter JSON Data by Specific Keywords in JavaScript
If you've ever worked with JSON data, you might have faced the challenge of filtering through it to find specific information. For instance, you may need to extract URLs from a JSON response based on certain keywords present in the data. In this guide, we will explore how to filter JSON data to display only the fields that match specified keywords using JavaScript.
Understanding JSON Structure
Before diving into the solution, let's break down an example JSON structure to understand how to extract the required information. Here we have a sample JSON object containing links and nested thumbnail data:
[[See Video to Reveal this Text or Code Snippet]]
In this JSON structure, each thumbnail object includes a URL (href) and an array of relationship identifiers (rel). Our goal is to filter this data based on keywords present in the rel array.
Step-by-Step Solution
1. Filtering Based on Keywords
To filter the JSON data, we can use JavaScript's array .filter() method along with .includes(). This approach allows us to filter the thumbnail entries depending on whether a specific keyword is present in the rel array.
Here’s how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
In this snippet, filterBy is a function that accepts a keyword (toFilter). It filters the thumbnail array to return only those objects where the rel array includes the specified keyword.
2. Extracting Only the URLs
If you're only interested in extracting the URLs (href) from the objects that match your filter criteria, you can chain the .map() method to the filter function:
[[See Video to Reveal this Text or Code Snippet]]
This function, filterByUrls, will return an array of URLs corresponding to the filtered objects.
3. Excluding Certain Keywords
Sometimes, you may want to also exclude certain keywords. This can easily be implemented by modifying our filter function a bit more:
[[See Video to Reveal this Text or Code Snippet]]
In this modification, if toExclude is set to true, the function will return thumbnails that do not include the specified keyword.
Practical Examples
Let's see these newly defined functions in action:
Filtering for 'twitter':
[[See Video to Reveal this Text or Code Snippet]]
Excluding 'twitter':
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Filtering JSON data in JavaScript is a straightforward task that becomes incredibly useful when handling large datasets. By using .filter() and .map(), you can efficiently extract the information you need based on specific keywords. Depending on your requirements, you can also modify the filter conditions to include or exclude certain values.
Now that you have the tools at your disposal, feel free to experiment with more complex JSON structures and filtering conditions. Happy coding!