filmov
tv
Problem 8-Anagram Check Analysis Using Python - Competitive Programming
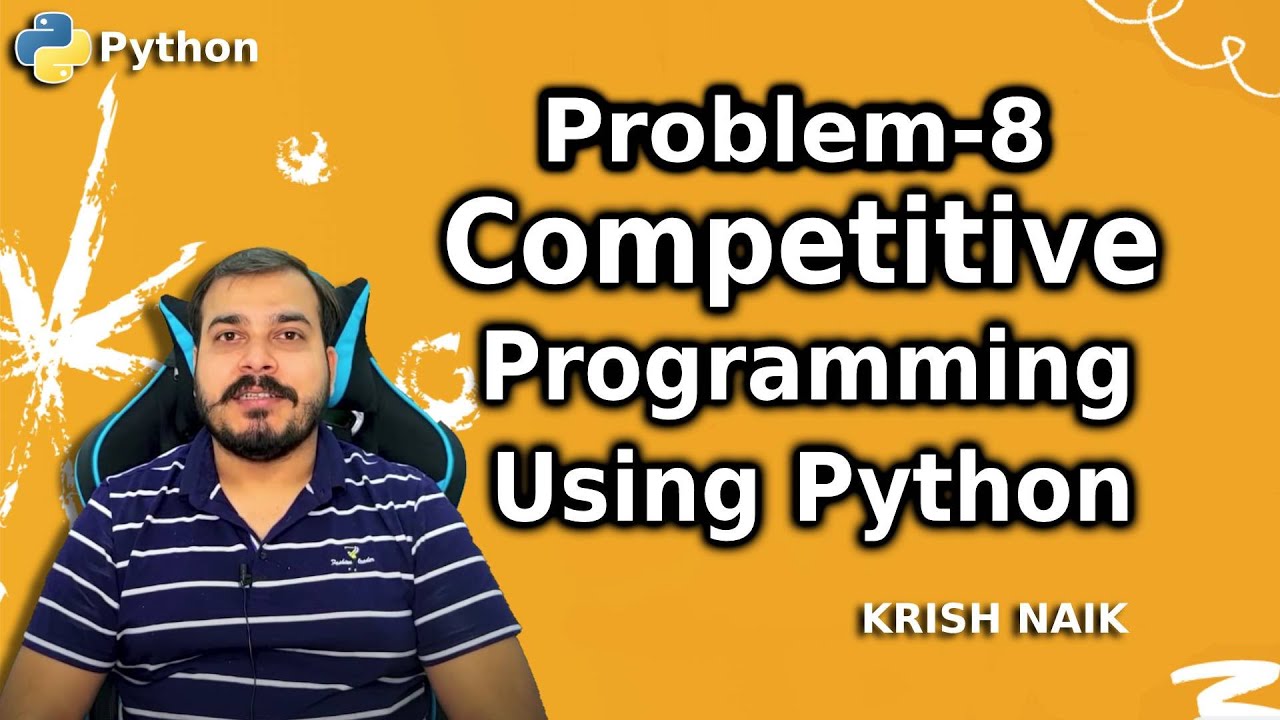
Показать описание
All Playlist In My channel
Please donate if you want to support the channel through GPay UPID,
Please join as a member in my channel to get additional benefits like materials in Data Science, live streaming for Members and many more
Please do subscribe my other channel too
Connect with me here:
Please donate if you want to support the channel through GPay UPID,
Please join as a member in my channel to get additional benefits like materials in Data Science, live streaming for Members and many more
Please do subscribe my other channel too
Connect with me here:
Problem 8-Anagram Check Analysis Using Python - Competitive Programming
Problem 8 Solution-Anagram Check Analysis Using Python - Competitive Programming
Analysis 4 | Anagrams Example 2
Analysis 3 | Anagrams Example 1
Technical Interview: Anagram
Anagram - Python Dictionary
How to Check if Two Strings are Anagram with Python Code 🔥
How to Solve Anagrams Using Python | PyEnchant Library & Permutations
Anagram C program
RS School Basics - s01e08 - Anagram
Python Anagram Check
Simple Python Scripts #5 - Anagram Checker
Check Permutation or Anagrams Between Two Strings {Using a Hash-map / Dictionary}
How to check if Two Strings are Anagrams in Python
An English Lesson Like No Other - What's the Icebreaking Game?
PROGRAM TO CHECK ANAGRAM STRINGS IN PYTHON || PYTHON PROGRAMMING || ANAGRAM STRINGS
Python Problem Solution 3 | Program To Check Given Two Strings Are Anagrams (split() and sort() )
How to find ANAGRAM in Python without in-built functions
Analysis 4 | Anagrams 2
Anagram Generator - CS50 2014 Final Project
India - Technical Interview Workshop
Check if 2 strings are Anagrams | Java | Strings | Data Structures and Algorithms
Amazon Interview Question: Find Anagram in a String | Mock Interview | Preplaced
01_anagram_solution 2
Комментарии