filmov
tv
Factory Method Design Pattern
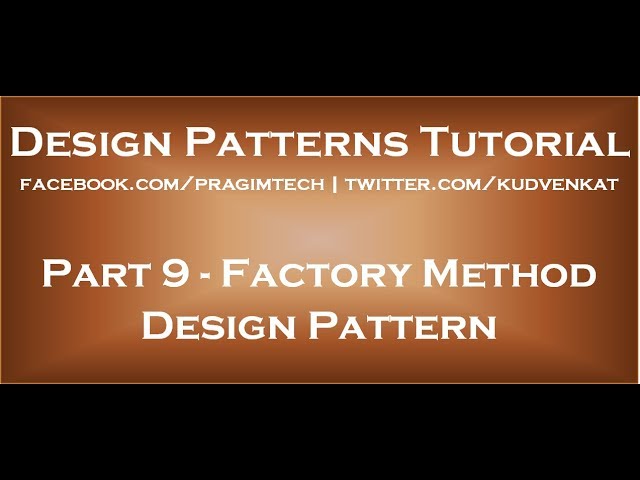
Показать описание
Text version of the video
Healthy diet is very important both for the body and mind. If you like Aarvi Kitchen recipes, please support by sharing, subscribing and liking our YouTube channel. Hope you can help.
Slides
Design Patterns Tutorial playlist
Design Patterns Text articles and slides
All Dot Net and SQL Server Tutorials in English
All Dot Net and SQL Server Tutorials in Arabic
In this tutorial we will learn
1. Simple Factory
2. Factory Method Pattern Implementation
Recap Simple Factory
1. Simple factory abstracts the creation details of the product
2. Simple factory refers to the newly created object through an interface
3. Any new type creation is handed with a change of code in the factory class and not at the client code
Factory Method Pattern Example
Business Requirement
1. Differentiate employees as permanent and contract and segregate their pay scales as well as bonus based on their employee types. ( We have achieved this using simple factory in the Part 8 of the Factory Pattern introduction session)
2. Calculate Permanent employee house rent allowance
3. Calculate Contract employee medical allowance
Steps to solve the above business requirement
Step 1: Add HouseAllowance and MedicalAllowance to the existing Employee table.
CREATE TABLE [dbo].[Employee] (
[Id] INT IDENTITY (1, 1) NOT NULL,
[Name] VARCHAR (50) NOT NULL,
[JobDescription] VARCHAR (50) NOT NULL,
[Number] VARCHAR (50) NOT NULL,
[Department] VARCHAR (50) NOT NULL,
[HourlyPay] DECIMAL (18) NOT NULL,
[Bonus] DECIMAL (18) NOT NULL,
[EmployeeTypeID] INT NOT NULL,
[HouseAllowance] DECIMAL (18) NULL,
[MedicalAllowance] DECIMAL (18) NULL,
PRIMARY KEY CLUSTERED ([Id] ASC),
CONSTRAINT [FK_Employee_EmployeeType] FOREIGN KEY ([EmployeeTypeID]) REFERENCES [dbo].[Employee_Type] ([Id]) );
Step 3: Create FactoryMethod folder under existing Factory folder and add BaseEmployeeFactory class.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Web.Managers;
using Web.Models;
namespace Web.Factory.FactoryMethod
{
public abstract class BaseEmployeeFactory
{
protected Employee _emp;
public BaseEmployeeFactory(Employee emp)
{
_emp = emp;
}
public Employee ApplySalary()
{
IEmployeeManager manager = this.Create();
_emp.Bonus = manager.GetBonus();
_emp.HourlyPay = manager.GetPay();
return _emp;
}
public abstract IEmployeeManager Create();
}
}
Step 4: Create ContractEmployeeFactory class under FactoryMethod folder.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Web.Managers;
using Web.Models;
namespace Web.Factory.FactoryMethod
{
public class ContractEmployeeFactory : BaseEmployeeFactory
{
public ContractEmployeeFactory(Employee emp) : base(emp)
{
}
public override IEmployeeManager Create()
{
ContractEmployeeManager manager = new ContractEmployeeManager();
_emp.MedicalAllowance = manager.GetMedicalAllowance();
return manager;
}
}
}
Step 5: Create PermanentEmployeeFactory class under FactoryMethod folder.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Web.Managers;
using Web.Models;
namespace Web.Factory.FactoryMethod
{
public class PermanentEmployeeFactory : BaseEmployeeFactory
{
public PermanentEmployeeFactory(Employee emp) : base(emp)
{
}
public override IEmployeeManager Create()
{
PermanentEmployeeManager manager = new PermanentEmployeeManager();
_emp.HouseAllowance = manager.GetHouseAllowance();
return manager;
}
}
}
Step 6: Create EmployeeManagerFactory class under FactoryMethod folder and add new Method CreateFactory which returns BaseEmployeeFactory.
CreateFactory method is responsible to return base factory which is the base class of Permanent and Contract Factories.
Healthy diet is very important both for the body and mind. If you like Aarvi Kitchen recipes, please support by sharing, subscribing and liking our YouTube channel. Hope you can help.
Slides
Design Patterns Tutorial playlist
Design Patterns Text articles and slides
All Dot Net and SQL Server Tutorials in English
All Dot Net and SQL Server Tutorials in Arabic
In this tutorial we will learn
1. Simple Factory
2. Factory Method Pattern Implementation
Recap Simple Factory
1. Simple factory abstracts the creation details of the product
2. Simple factory refers to the newly created object through an interface
3. Any new type creation is handed with a change of code in the factory class and not at the client code
Factory Method Pattern Example
Business Requirement
1. Differentiate employees as permanent and contract and segregate their pay scales as well as bonus based on their employee types. ( We have achieved this using simple factory in the Part 8 of the Factory Pattern introduction session)
2. Calculate Permanent employee house rent allowance
3. Calculate Contract employee medical allowance
Steps to solve the above business requirement
Step 1: Add HouseAllowance and MedicalAllowance to the existing Employee table.
CREATE TABLE [dbo].[Employee] (
[Id] INT IDENTITY (1, 1) NOT NULL,
[Name] VARCHAR (50) NOT NULL,
[JobDescription] VARCHAR (50) NOT NULL,
[Number] VARCHAR (50) NOT NULL,
[Department] VARCHAR (50) NOT NULL,
[HourlyPay] DECIMAL (18) NOT NULL,
[Bonus] DECIMAL (18) NOT NULL,
[EmployeeTypeID] INT NOT NULL,
[HouseAllowance] DECIMAL (18) NULL,
[MedicalAllowance] DECIMAL (18) NULL,
PRIMARY KEY CLUSTERED ([Id] ASC),
CONSTRAINT [FK_Employee_EmployeeType] FOREIGN KEY ([EmployeeTypeID]) REFERENCES [dbo].[Employee_Type] ([Id]) );
Step 3: Create FactoryMethod folder under existing Factory folder and add BaseEmployeeFactory class.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Web.Managers;
using Web.Models;
namespace Web.Factory.FactoryMethod
{
public abstract class BaseEmployeeFactory
{
protected Employee _emp;
public BaseEmployeeFactory(Employee emp)
{
_emp = emp;
}
public Employee ApplySalary()
{
IEmployeeManager manager = this.Create();
_emp.Bonus = manager.GetBonus();
_emp.HourlyPay = manager.GetPay();
return _emp;
}
public abstract IEmployeeManager Create();
}
}
Step 4: Create ContractEmployeeFactory class under FactoryMethod folder.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Web.Managers;
using Web.Models;
namespace Web.Factory.FactoryMethod
{
public class ContractEmployeeFactory : BaseEmployeeFactory
{
public ContractEmployeeFactory(Employee emp) : base(emp)
{
}
public override IEmployeeManager Create()
{
ContractEmployeeManager manager = new ContractEmployeeManager();
_emp.MedicalAllowance = manager.GetMedicalAllowance();
return manager;
}
}
}
Step 5: Create PermanentEmployeeFactory class under FactoryMethod folder.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using Web.Managers;
using Web.Models;
namespace Web.Factory.FactoryMethod
{
public class PermanentEmployeeFactory : BaseEmployeeFactory
{
public PermanentEmployeeFactory(Employee emp) : base(emp)
{
}
public override IEmployeeManager Create()
{
PermanentEmployeeManager manager = new PermanentEmployeeManager();
_emp.HouseAllowance = manager.GetHouseAllowance();
return manager;
}
}
}
Step 6: Create EmployeeManagerFactory class under FactoryMethod folder and add new Method CreateFactory which returns BaseEmployeeFactory.
CreateFactory method is responsible to return base factory which is the base class of Permanent and Contract Factories.
Комментарии